In Go language functions, GC automatically reclaims memory that is no longer used. The implementation method is: tracking mark: GC thread marks all reachable objects. Clear: Clear objects marked as garbage and reclaim their memory. For example, the slice s created in function f is marked as garbage and collected when f returns.
Implementation of GC in Go language function
In Go language, garbage collection (GC) is automatically performed by the runtime to reclaim memory that is no longer in use. When a function returns, the GC examines the objects referenced in the function's stack frame and marks objects that are no longer needed as garbage.
The implementation method of GC
The GC of Go language adopts the generational mark-clear algorithm:
- Tracking mark ( Mark): The GC thread will traverse the heap memory and mark all reachable objects. A reachable object refers to an object that can be traced through a pointer from the program root object (such as global variables, method values).
- Sweep: After the marking is completed, the GC thread will clear all objects marked as garbage and reclaim the memory they occupy.
Practical case
The following code demonstrates the behavior of GC in a function:
package main import "fmt" import "runtime" func main() { // 创建一个匿名函数,并在其内部分配内存 f := func() { var s []int for i := 0; i < 1000000; i++ { s = append(s, i) } } // 调用匿名函数 f() // GC 标记函数堆栈帧中的对象 runtime.GC() // GC 清除不再需要的对象 runtime.GC() }
In this example, anonymous functionf
Creates a s
slice and appends 1 million integers to it. When f
returns, the s
slice is no longer referenced, so the GC marks it as garbage and reclaims the memory it occupies.
By running runtime.GC()
before and after the anonymous function returns, we can force the GC to execute immediately and observe how the memory occupied by f
is released of.
The above is the detailed content of How is GC implemented in golang functions?. For more information, please follow other related articles on the PHP Chinese website!
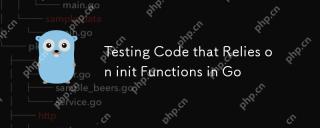
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
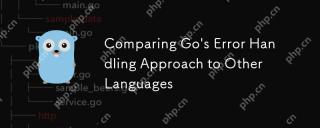
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
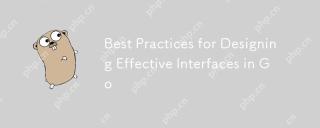
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
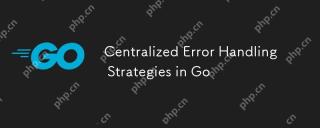
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.
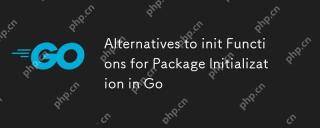
InGo,alternativestoinitfunctionsincludecustominitializationfunctionsandsingletons.1)Custominitializationfunctionsallowexplicitcontroloverwheninitializationoccurs,usefulfordelayedorconditionalsetups.2)Singletonsensureone-timeinitializationinconcurrent
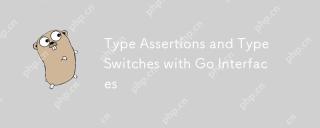
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
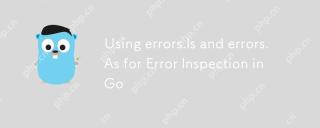
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
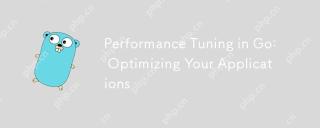
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
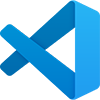
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
