Building multi-tenant web applications in C involves isolating each tenant's data. This can be achieved in two main ways: using containers (like an unordered map) or using scope isolation (limiting the scope of a variable). The container approach stores each tenant's data in the container (key: tenant ID, value: tenant data), while the scope isolation approach limits the scope of variables to a specific block of code, enabling multi-tenancy.
Building Multi-Tenant Web Applications in C
Introduction
Multiple Tenant web applications allow multiple tenants (customers) to share the same application instance while still maintaining data isolation. This is useful for SaaS applications with a large number of tenants. This article will guide you on how to build multi-tenant applications in C using modern C frameworks.
Multi-tenancy using containers
One way to create multi-tenant applications in C is to use containers. Containers are lightweight data structures used to store data objects. We can use different containers to store data for each tenant, for example:
std::unordered_map<int, TenantData> tenant_data;
In this code, tenant_data
is an unordered map that stores key-value pairs. The key is the tenant ID and the value is the tenant data.
Multi-tenancy using scope isolation
Another way to implement multi-tenancy in C is to use scope isolation. Scope isolation refers to limiting the scope of a variable so that it is only visible within a given block. We can implement multi-tenancy using scope isolation introduced in C 11:
{ TenantData tenant_data; // 在此范围内访问 tenant_data } // 在此范围之外无法访问 tenant_data
Practical Case
Consider a SaaS application with tenant data. We can use containers to store data for each tenant. The following code shows how to achieve this using an unordered map:
#include <sstream> #include <iostream> #include <map> class TenantData { public: int id; std::string name; }; std::map<int, TenantData> tenant_data; // 在容器中创建或更新租户数据 void createOrUpdateTenantData(int id, std::string name) { tenant_data[id] = TenantData{id, name}; } // 从容器中获取租户数据 TenantData getTenantData(int id) { return tenant_data[id]; } // 打印所有租户数据 void printAllTenantData() { for (const auto& [id, data] : tenant_data) { std::cout << "Tenant " << id << ": " << data.name << std::endl; } } int main() { // 创建或更新一些租户数据 createOrUpdateTenantData(1, "Tenant 1"); createOrUpdateTenantData(2, "Tenant 2"); createOrUpdateTenantData(3, "Tenant 3"); // 获取特定租户的数据 TenantData tenant1_data = getTenantData(1); std::cout << "Tenant 1: " << tenant1_data.name << std::endl; // 打印所有租户的数据 printAllTenantData(); return 0; }
Conclusion
By using containers or scope isolation, you can build powerful Multi-tenant web applications. By isolating each tenant's data, you can ensure data security between tenants and maintain application performance.
The above is the detailed content of How to create a multi-tenant web application in C++?. For more information, please follow other related articles on the PHP Chinese website!
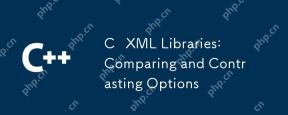
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
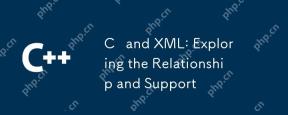
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
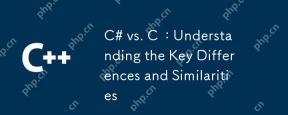
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
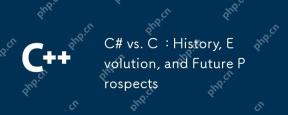
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
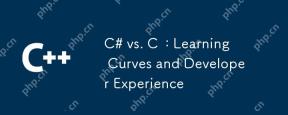
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
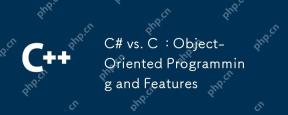
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
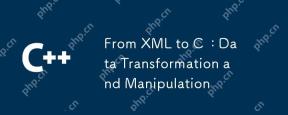
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
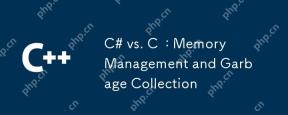
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
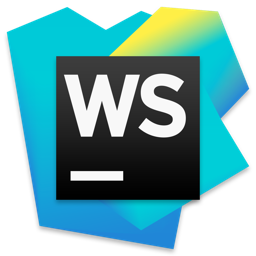
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
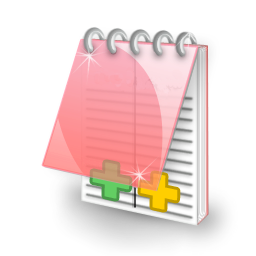
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software