Common pitfalls and solutions in C++ concurrent programming
Common pitfalls and solutions: Data race: Use synchronization mechanisms (such as mutexes) to ensure data integrity. Deadlock: Use deadlock detection or resource acquisition sequential design. Priority inversion: Use priority inheritance or ceiling protocols. Thread starvation: Use fair or time slice scheduling algorithms. Non-cancellable operations: Use cancelable threads or tasks to implement cancellation functionality.
C Common pitfalls and solutions of concurrent programming
Concurrent programming is the use of multiple cores or processors to execute multiple tasks at the same time. A programming technique for tasks. In C, concurrency can be achieved using threads, tasks, or coroutines. However, there are some common pitfalls in concurrent programming that, if not addressed, can lead to deadlocks, data races, and performance issues.
1. Data competition
Data competition means that when multiple threads access the same memory, there is no appropriate synchronization mechanism to ensure the integrity of the data. This can lead to data inconsistency issues.
Solution: Use mutexes, locks, or atomic variables to synchronize access to shared data.
2. Deadlock
Deadlock occurs when two or more threads wait for each other to release resources. It causes all involved threads to wait indefinitely.
Solution: Use deadlock detection and recovery mechanisms, or carefully design the order of resource acquisition between threads.
3. Priority inversion
Priority inversion means that a low-priority thread occupies the resources required by a high-priority thread, resulting in high Priority threads cannot obtain necessary resources.
Solution: Use priority inheritance or a priority ceiling protocol to prevent priority inversion.
4. Thread starvation
Thread starvation means that a thread cannot obtain execution time for a long time, causing it to be unable to complete its task.
Solution: Use fair scheduling algorithm or time slice scheduling algorithm to ensure that each thread gets appropriate execution time.
5. Non-cancellable operation
Non-cancellable operation means that once a thread is started, it cannot be canceled by other threads.
Solution: Use cancelable threads, tasks or coroutines to implement cancelable operations.
Practical case
The following is an example of using threads in C to implement concurrent calculations:
#include <iostream> #include <thread> using namespace std; void printMessage(const string& message) { cout << "Thread " << this_thread::get_id() << ": " << message << endl; } int main() { thread t1(printMessage, "Hello, world!"); thread t2(printMessage, "Goodbye, world!"); t1.join(); t2.join(); return 0; }
In this example, two threads are concurrent print information. By using the join()
method, the main thread waits for the two child threads to complete execution.
The above is the detailed content of Common pitfalls and solutions in C++ concurrent programming. For more information, please follow other related articles on the PHP Chinese website!
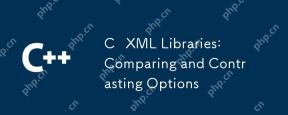
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
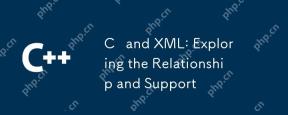
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
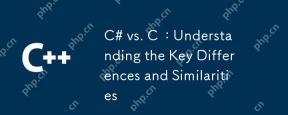
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
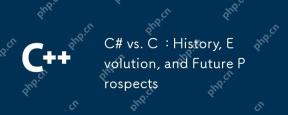
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
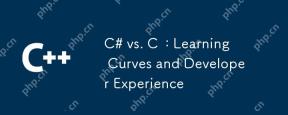
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
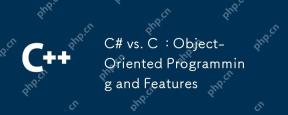
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
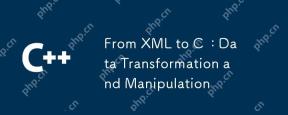
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
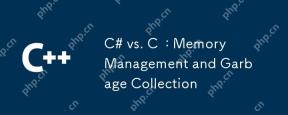
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Notepad++7.3.1
Easy-to-use and free code editor