


How to integrate external data sources in C++ to enrich the analysis process?
Integrating external data sources in C++ can greatly expand data analysis capabilities. The steps include selecting a connector that is compatible with the target data source, establishing a connection based on the data source requirements, and querying using SQL. An example of connecting to MySQL using the ODBC connector shows how to extract the data results. Integrating external data sources enriches the analysis process and enables more informed decisions.
How to integrate external data sources in C++ to enrich the analysis process
Introduction
In the data analysis process, integrating external data sources can greatly expand the breadth and depth of analysis. As a powerful programming language, C++ provides rich functions and can be easily integrated with various external data sources. This article will guide you in seamlessly integrating external data sources into your analysis process using C++.
Steps
- Select a connector: First, you need to select a connector that is compatible with the target data source. Popular connectors include ODBC, JDBC, and ADO.NET.
- Establish a connection: Use the selected connector to establish a connection based on the specific requirements of the data source. You need to provide a connection string, which includes the data source name, username, and password.
- Execute a query: After connecting, you can use SQL to query the data source. You can perform select, insert, update, and delete operations.
Practical case
The following is an example of using the ODBC connector to integrate a MySQL database:
#include <iostream> #include <sql.h> using namespace std; int main() { // 连接字符串 const char* dsn = "DSN=my_mysql_db"; const char* user = "root"; const char* password = "secret"; // 连接 SQLHENV env; SQLHDBC dbc; SQLAllocHandle(SQL_HANDLE_ENV, SQL_NULL_HANDLE, &env); SQLAllocHandle(SQL_HANDLE_DBC, env, &dbc); SQLConnect(dbc, (SQLCHAR*)dsn, SQL_NTS, (SQLCHAR*)user, SQL_NTS, (SQLCHAR*)password, SQL_NTS); // 查询 SQLHSTMT stmt; SQLAllocHandle(SQL_HANDLE_STMT, dbc, &stmt); SQLPrepare(stmt, (SQLCHAR*)"SELECT * FROM customer", SQL_NTS); SQLExecute(stmt); // 提取结果 SQLINTEGER id; SQLCHAR name[256]; SQLGetData(stmt, 1, SQL_C_SLONG, &id, sizeof(id), NULL); SQLGetData(stmt, 2, SQL_C_CHAR, name, sizeof(name), NULL); cout << "ID: " << id << endl; cout << "Name: " << name << endl; // 释放资源 SQLFreeHandle(SQL_HANDLE_STMT, stmt); SQLDisconnect(dbc); SQLFreeHandle(SQL_HANDLE_DBC, dbc); SQLFreeHandle(SQL_HANDLE_ENV, env); return 0; }
Conclusion
Integrating external data sources can greatly enhance data analysis capabilities in C++. By following the steps outlined in this article, you can seamlessly connect to a variety of data sources, enrich your analysis process, and make more informed decisions.
The above is the detailed content of How to integrate external data sources in C++ to enrich the analysis process?. For more information, please follow other related articles on the PHP Chinese website!
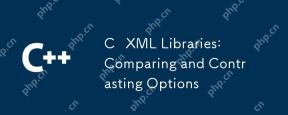
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
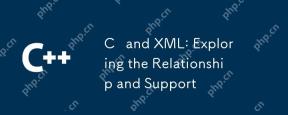
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
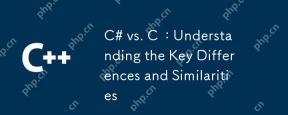
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
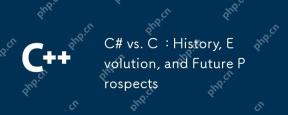
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
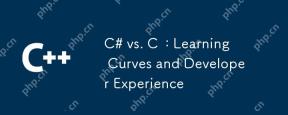
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
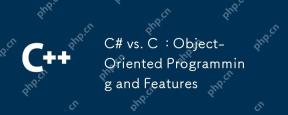
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
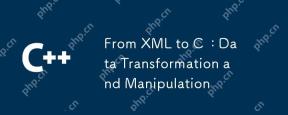
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
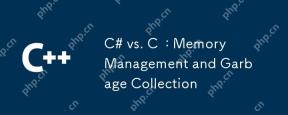
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
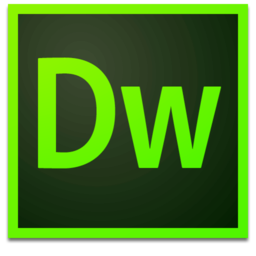
Dreamweaver Mac version
Visual web development tools
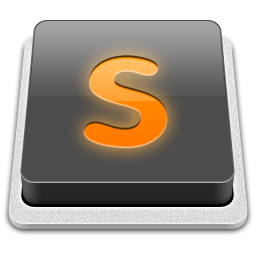
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
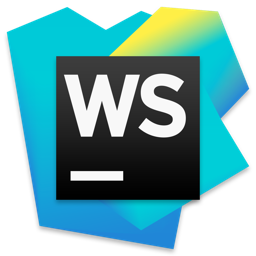
WebStorm Mac version
Useful JavaScript development tools