In the Go framework development process, automated testing is crucial to ensure code reliability and shorten the release cycle. This article introduces the steps for automated testing using the Go language and related tools: Tool selection: The Go standard library provides "go test", the assertion library "testify" is used for concise testing, "go-mockgen" generates mock object code, "ginkgo" " then supports BDD testing. Test types: Including unit testing (single function) and integration testing (component interaction). Test Example: The Sum function unit test example demonstrates running tests using "go test". BDD style testing: Use "ginkgo" to write behavior-driven development tests, focusing on
Automated testing in the Go framework development process
In the modern Go framework development process , automated testing is indispensable. Through automated testing, we can ensure the reliability and stability of the code and shorten the software release cycle. This article will guide you step by step to implement automated testing using the Go language and related tools.
Testing tools in the Go ecosystem
The Go language community provides a wealth of testing tools. The following are some commonly used tools:
- go test: Built-in testing tools in the Go standard library.
- testify: A popular assertion library for writing clear and concise test cases.
- go-mockgen: A tool that can automatically generate mock object code.
- ginkgo: A BDD-style testing framework.
Test type
In Go framework development, two types of test cases usually need to be written:
- Unit test: Test a single function or component in the application.
- Integration testing: Test the interaction of different components in the application.
Automated Test Example
The following is a complete automated test example showing how to test a simple Go function.
// sum.go package main import "testing" func Sum(a, b int) int { return a + b } func TestSum(t *testing.T) { // 定义测试用例 testCases := []struct { input1 int input2 int expectedOutput int }{ {1, 2, 3}, {3, 4, 7}, {-1, 0, -1}, } for _, tc := range testCases { // 运行测试 result := Sum(tc.input1, tc.input2) // 使用 testify 的断言函数进行判断 if result != tc.expectedOutput { t.Errorf("Error: expected %d, got %d", tc.expectedOutput, result) } } }
Run the test using go test
To run the test, use the following command:
go test
This command will run all functions starting with Test
.
Writing BDD style tests using ginkgo
BDD (Behavior Driven Development) style testing focuses on user stories and application behavior. You can use ginkgo to write BDD test cases.
The following is an example of writing a test case using ginkgo:
package sum import ( "testing" . "github.com/onsi/ginkgo" . "github.com/onsi/gomega" ) var _ = Describe("Sum function", func() { It("adds two numbers correctly", func() { result := Sum(1, 2) Expect(result).To(Equal(3)) }) })
To run a ginkgo test, use the following command:
ginkgo
Conclusion
Automated testing is An integral part of the Go framework development process. By using the Go language and tools provided by the community, you can easily write and run automated test cases to ensure the reliability and stability of your application.
The above is the detailed content of Automated testing in the golang framework development process. For more information, please follow other related articles on the PHP Chinese website!
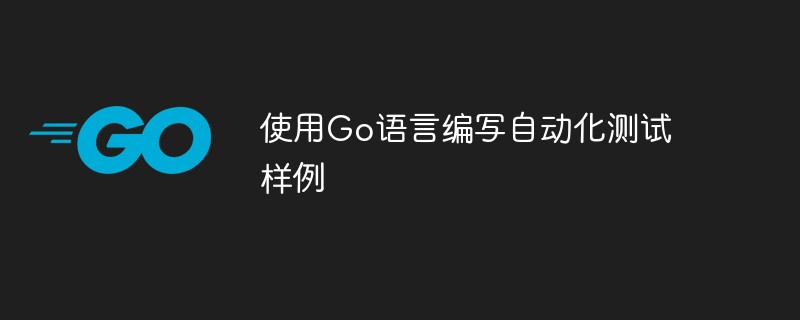
随着软件开发的迅速发展,自动化测试在开发过程中扮演着越来越重要的角色。相较于人工测试,自动化测试可以提高测试的效率和准确性,减少交付的时间和成本。因此,掌握自动化测试变得非常必要。Go语言是一门现代化的、高效的编程语言,由于其特有的并发模型、内存管理和垃圾回收机制,使得它在Web应用、网络编程、大规模并发、分布式系统等领域有着广泛的应用。而在自动化测试方面,
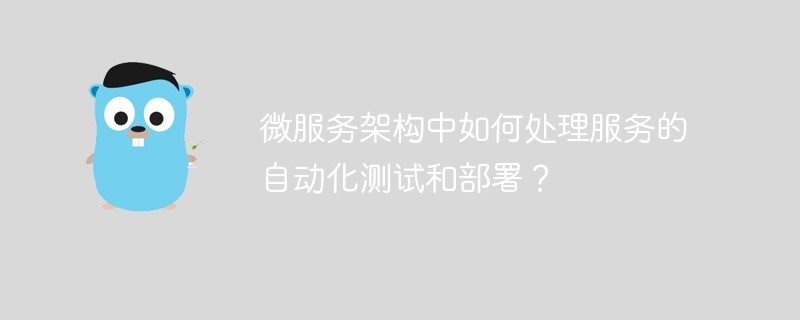
随着互联网技术的快速发展,微服务架构也越来越被广泛应用。使用微服务架构可以有效避免单体应用的复杂度和代码耦合,提高应用的可扩展性和可维护性。然而,与单体应用不同,在微服务架构中,服务数量庞大,每个服务都需要进行自动化测试和部署,以确保服务的质量和可靠性。本文将针对微服务架构中如何处理服务的自动化测试和部署进行探讨。一、微服务架构中的自动化测试自动化测试是保证
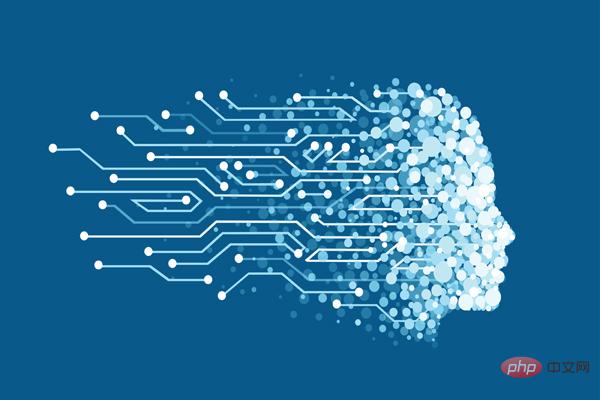
译者 | 陈峻审校 | 孙淑娟近年来,自动化测试已经发生了重大的迭代。它在很大程度上协助QA团队减少了人为错误的可能。虽然目前有许多工具可以被用于自动化测试,但合适的工具一直是自动化测试成败与否的关键。同时,随着人工智能、机器学习和神经网络在各个领域的广泛运用,面向人工智能的自动化测试也需要通过合适的工具,来承担重复性的工作,以节省项目团队宝贵的时间,去执行更加复杂和关键的任务。下面,我将和您深入探讨面向未来的AI自动化测试工具。什么是人工智能(AI)自动化测试?AI自动化测试意味着现有的软件
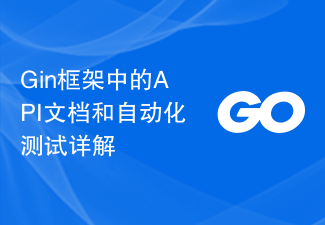
Gin是一个用Golang编写的Web框架,它具有高效、轻量、灵活等优点,性能相对较高,并且易于使用。在Gin框架开发中,API文档和自动化测试十分重要。本文将深入探讨Gin框架中的API文档和自动化测试。一、API文档API文档用于记录所有API接口的详细信息,方便其他开发人员使用和理解。Gin框架提供了多种API文档工具,包括Swagger、GoSwa
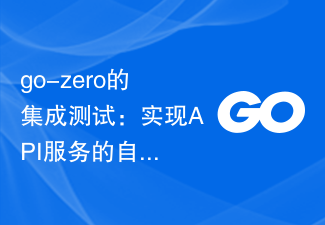
随着互联网企业的不断壮大,软件开发的复杂性越来越高,测试工作也越来越重要。为了保证程序的正确性和稳定性,必须进行各种类型的测试。其中自动化测试是一种非常重要的方式,它可以提高测试工作效率,减少错误率,并且允许重复执行测试用例以便早发现问题,但是在实际操作过程中,我们也会遇到种种的问题,比如测试工具的选择、测试用例的编写以及测试环境的搭建等问题。go-zero
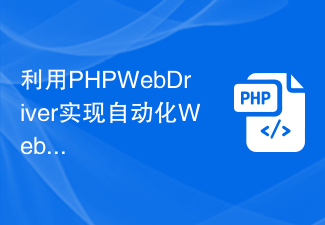
随着Web应用程序的普及和互联网的飞速发展,WebUI测试已经成为软件开发过程中不可忽视的一环。自动化WebUI测试是提高测试效率,缩短项目周期的有效手段。本文将介绍利用PHPWebDriver实现自动化WebUI测试的最佳实践。一、什么是PHPWebDriver?PHPWebDriver是一个基于WebBrowserAutomationA
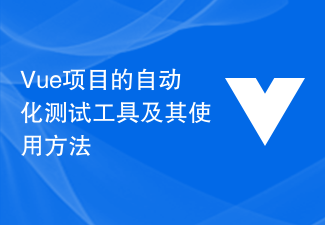
随着Vue技术的不断发展,越来越多的企业开始使用Vue来开发前端应用。但是,在开发过程中,如何保证代码的质量和稳定性呢?这时候,自动化测试就成为了必不可少的一环。本文将介绍Vue项目中的自动化测试工具及其使用方法,帮助开发者更好地进行测试和验证。一、自动化测试的概述自动化测试是指使用自动化工具来执行测试方案,并发布测试结果。与手动测试相比,自动化测试可以更快
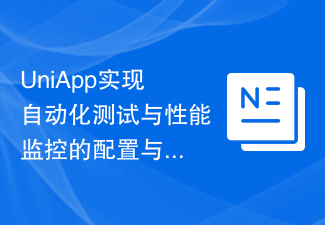
UniApp是一款跨平台的应用开发框架,可以快速开发出同时适配多个平台的应用程序。在开发过程中,我们经常需要进行自动化测试和性能监控来保证应用的质量和性能。本文将为大家介绍UniApp如何配置和使用自动化测试与性能监控的工具。一、自动化测试配置与使用指南下载并安装必要的工具UniApp的自动化测试依赖于Node.js和WebdriverIO。首先,我们需要下


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
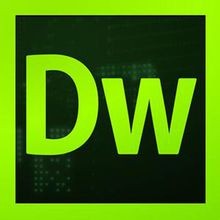
Dreamweaver CS6
Visual web development tools
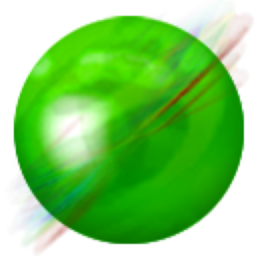
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
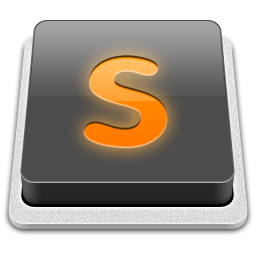
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
