


In the previous blog, I wrote about using javascript to generate multiple groups of text , which can prevent data input from being displayed. Now we need to write these inputs into the database, and here we use json to pass them in.
First, let’s write about how the background generates the data to be transmitted
[html]
function generateDtb() {
//Write
var txtName = document.getElementById("txtName").value;
//Create array
var dtb = new Array();
//Write data to the array through loop and return
for (var i = 0; i var row = new Object();
row.Name = txtName;
row.fullMoney = firstGroup[i].value;
row.discount = secondGroup[i].value;
dtb.push( row);
}
return dtb;
}
function generateDtb() {
//Write
var txtName = document.getElementById("txtName").value;
//Create an array
var dtb = new Array();
//Write data into the array through a loop and return
for (var i = 0; i var row = new Object();
row.Name = txtName;
row.fullMoney = firstGroup[i].value;
row.discount = secondGroup[i].value;
dtb.push(row);
}
return dtb;
}
Convert the array into a json string and pass it to the background:
[html]
$(function () {
//Click botton1
$("#lbtnOK").click(function () {
var url = "DiscountManger.aspx?ajax=1";
var dtb = generateDtb();
// var strName = document.getElementById("txtName").value;
if (dtb == null)
{ }
else {
//Serialized object
var postdata = JSON.stringify (dtb);
//Asynchronous request
$.post(url, { json: postdata }, function (json) {
if (json) {
jBox.tip("Added successfully! ", "tip");
location.reload();
}
else {
jBox.tip("Add failed!", "tip");
location.reload( );
}
}, "json")
}
});
});
$(function () {
//Click botton1
$("#lbtnOK").click(function () {
var url = "DiscountManger.aspx?ajax=1";
var dtb = generateDtb();
// var strName = document. getElementById("txtName").value;
if (dtb == null)
{ }
else {
//Serialized object
var postdata = JSON.stringify(dtb);
//Asynchronous request
$.post(url, { json: postdata }, function (json) {
if (json) {
jBox.tip("Added successfully!", "Tip ");
location.reload();
}
else {
jBox.tip("Add failed!", "Tip");
location.reload();
}
}, "json")
}
});
});
Operations in the background:
First determine whether data needs to be transmitted
[html]
if (!IsPostBack)
{
//Determine whether to request asynchronously
if (Request.QueryString["ajax"] == "1")
{
ProcessRequest();
}
if (!IsPostBack)
{
//Determine whether to request asynchronously
if (Request.QueryString["ajax"] == "1")
{
ProcessRequest();
}
Process the data here:
[html]
///
/// Processing asynchronous requests
///
private void ProcessRequest()
{
// Deposit the strategy to be filled in
ArrayList arrDiscount = new ArrayList();
Response.ContentType = "text/html";
string json = Request.Form["json"];
// Deserialize DataTable
if (json == null)
{
return;
}
else
{
DataTable newdtb = Json2Dtb(json);
for (int i = 0; i {
Entity.StrategyDiscount enStrategyDiscount = new Entity.StrategyDiscount();
//Discount plan name
enStrategyDiscount.name = newdtb.Rows[i]["Name"].ToString();
//Shop ID
enStrategyDiscount.shopId = long.Parse(LoginInfo.ShopID);
enStrategyDiscount.fullMoney = Convert.ToDecimal (newdtb.Rows[i]["fullMoney"].ToString());
enStrategyDiscount.discount = Convert.ToDecimal(newdtb.Rows[i]["discount"].ToString());
/ /Write data to the array
arrDiscount.Add(enStrategyDiscount);
}
//Write data to the database
IStrategyBLL strategy = new StrategyBLL();
if (strategy.AddStrategyDiscount( arrDiscount))
{
Response.Write("true");
Response.End();
}
else
{
Response.Write("false" );
Response.End();
}
}
///
/// Handling asynchronous requests
///
private void ProcessRequest()
{
//Save the policy to be filled in
ArrayList arrDiscount = new ArrayList();
Response.ContentType = "text/html";
string json = Request.Form["json"];
//Deserialize DataTable
if (json == null)
{
return;
}
else
{
DataTable newdtb = Json2Dtb(json);
for (int i = 0; i {
Entity.StrategyDiscount enStrategyDiscount = new Entity.StrategyDiscount( );
//Discount plan name
enStrategyDiscount.name = newdtb.Rows[i]["Name"].ToString();
//Store ID
enStrategyDiscount.shopId = long.Parse (LoginInfo.ShopID);
enStrategyDiscount.fullMoney = Convert.ToDecimal(newdtb.Rows[i]["fullMoney"].ToString());
enStrategyDiscount.discount = Convert.ToDecimal(newdtb.Rows[i ]["discount"].ToString());
//Write data to the array
arrDiscount.Add(enStrategyDiscount);
}
//Write data to the database
IStrategyBLL strategy = new StrategyBLL();
if (strategy.AddStrategyDiscount(arrDiscount))
{
Response.Write("true");
Response.End();
}
else
{
Response.Write("false");
Response.End();
}
}
Here, we need to put json Convert to datatable
[html]
///
/// Json to DataTable
///
///
// /
private DataTable Json2Dtb(string json)
{
JavaScriptSerializer jss = new JavaScriptSerializer();
ArrayList dic = jss.Deserialize
DataTable dtb = new DataTable();
if (dic.Count > 0)
{
foreach (Dictionary
{
if (dtb.Columns.Count == 0)
{
foreach (string key in drow.Keys)
{
dtb.Columns.Add(key, drow[key].GetType() );
}
}
DataRow row = dtb.NewRow();
foreach (string key in drow.Keys)
{
row[key] = drow[key] ;
}
dtb.Rows.Add(row);
}
}
return dtb;
}
///
// / Json to DataTable
///
///
///
private DataTable Json2Dtb(string json)
{
JavaScriptSerializer jss = new JavaScriptSerializer();
ArrayList dic = jss.Deserialize
DataTable dtb = new DataTable ();
if (dic.Count > 0)
{
foreach (Dictionary
{
if (dtb.Columns.Count == 0)
{
foreach (string key in drow.Keys)
{
dtb.Columns.Add(key, drow[key].GetType());
}
}
DataRow row = dtb.NewRow();
foreach (string key in drow.Keys)
{
row[key] = drow[key];
}
dtb .Rows.Add(row);
}
}
return dtb;
}
In this way, the data can be written to the database without refreshing.
Of course, if we have a datatable read from the database, what if it is displayed in the foreground through json.
First, we need to convert the datatable into json data
[html]
///
/// DataTable转Json
///
///
///
private string Dtb2Json(DataTable dtb)
{
JavaScriptSerializer jss = new JavaScriptSerializer();
ArrayList dic = new ArrayList();
foreach (DataRow row in dtb.Rows)
{
Dictionary
foreach (DataColumn col in dtb.Columns)
{
drow.Add(col.ColumnName, row[col.ColumnName]);
}
dic.Add(drow);
}
return jss.Serialize(dic);
}
///
/// DataTable转Json
///
///
///
private string Dtb2Json(DataTable dtb)
{
JavaScriptSerializer jss = new JavaScriptSerializer();
ArrayList dic = new ArrayList();
foreach (DataRow row in dtb.Rows)
{
Dictionary
foreach (DataColumn col in dtb.Columns)
{
drow.Add(col.ColumnName, row[col.ColumnName]);
}
dic.Add(drow);
}
return jss.Serialize(dic);
}
然后写回到前台
[html]
///
/// 处理异步请求
///
private void ProcessRequest()
{
Response.ContentType = "text/html";
string json = Request.Form["json"];
//反序列化DataTable
DataTable newdtb = Json2Dtb(json);
//序列化DataTable为JSON
string back = Dtb2Json(newdtb);
Response.Write(back);
Response.End();
}
///
/// 处理异步请求
///
private void ProcessRequest()
{
Response.ContentType = "text/html";
string json = Request.Form["json"];
//反序列化DataTable
DataTable newdtb = Json2Dtb(json);
//序列化DataTable为JSON
string back = Dtb2Json(newdtb);
Response.Write(back);
Response.End();
}
在前台接受显示:
[html]
$(function() {
//点击botton1
$("#botton1").click(function() {
createTable(json);
});
});
//显示Json中的数据
function createTable(json) {
var table = $("
for (var i = 0; i o1 = json[i];
var row = $("
for (key in o1) {
var td = $("
td.text(o1[key].toString());
td.appendTo(row);
}
row.appendTo(table);
}
table.appendTo($("#back"));
}
$(function() {
//点击botton1
$("#botton1").click(function() {
createTable(json);
});
});
//显示Json中的数据
function createTable(json) {
var table = $("
for (var i = 0; i o1 = json[i];
var row = $("
for (key in o1) {
var td = $("
td.text(o1[key].toString());
td.appendTo(row);
}
row.appendTo(table);
}
table.appendTo($("#back"));
}
这样,就完成了json向后台传输数据和显示后台数据了,当然,这种传输方式只是传输的一种,如果是简单的字符串也可以用get和post进行传输,但是,javascript本身具有不安全性和不稳定行,对于一些比较重要的数据,建议还是寻找一些更可靠的方法。
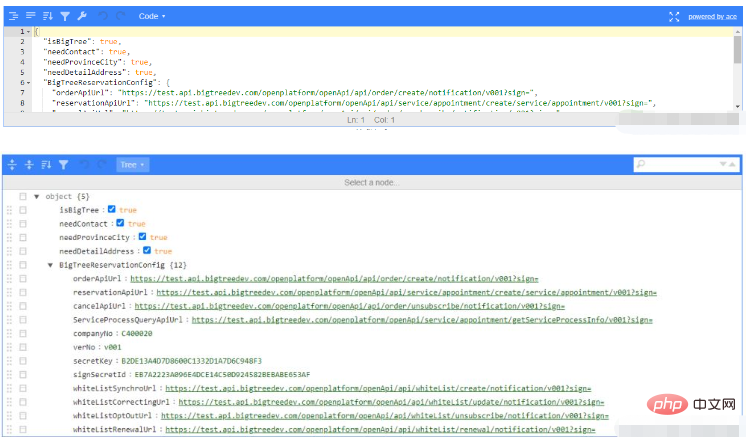
1、先看看效果图,可以自行选择展示效果2、这是我在vue3项目中使用的JSON编辑器,首先引入第三方插件npminstalljson-editor-vue3yarnaddjson-editor-vue33、引入到项目中//导入模块importJsonEditorVuefrom'json-editor-vue3'//注册组件components:{JsonEditorVue},4、一般后端返回的是会将JSON转为String形式我们传给后端也是通过这种形式,就可以通
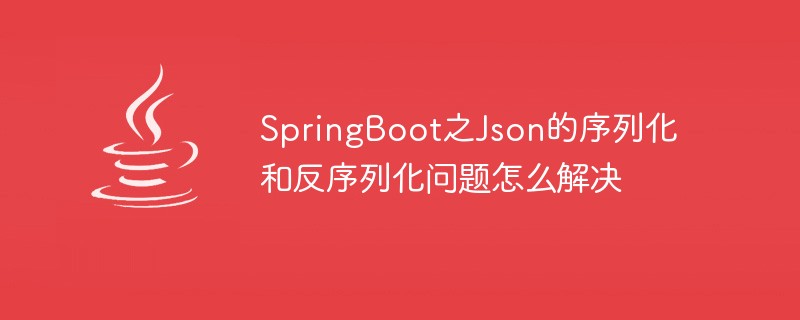
控制json序列化/反序列化1.@JsonIgnoreProperties的用法@JsonIgnoreProperties(value={"prop1","prop2"})用来修饰Pojo类,在序列化和反序列化的时候忽略指定的属性,可以忽略一个或多个属性.@JsonIgnoreProperties(ignoreUnknown=true)用来修饰Pojo类,在反序列化的时候忽略那些无法被设置的属性,包括无法在构造子设置和没有对应的setter方法.2.@Js
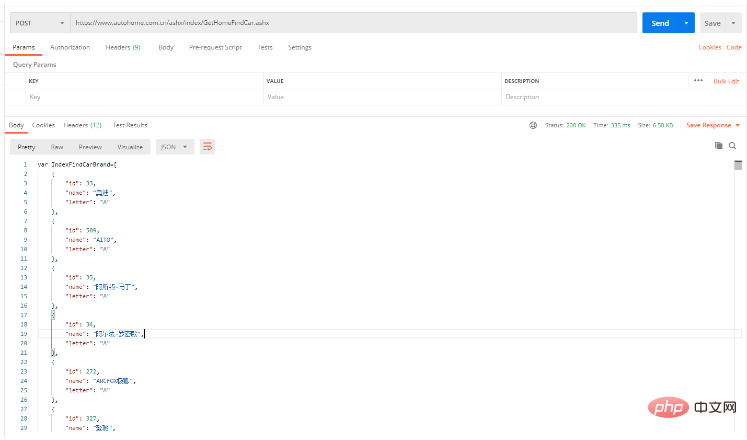
Java调用接口获取json数据保存到数据库1.在yml文件中配置自己定义的接口URL//自己定义的JSON接口URLblacklist_data_url:接口URL2.在Controller中添加请求方法和路径/***@Title:查询*@Description:查询车辆的记录*@Author:半度纳*@Date:2022/9/2717:33*/@GetMapping("/Blacklist")publicvoidselectBlacklist(){booleana=imB
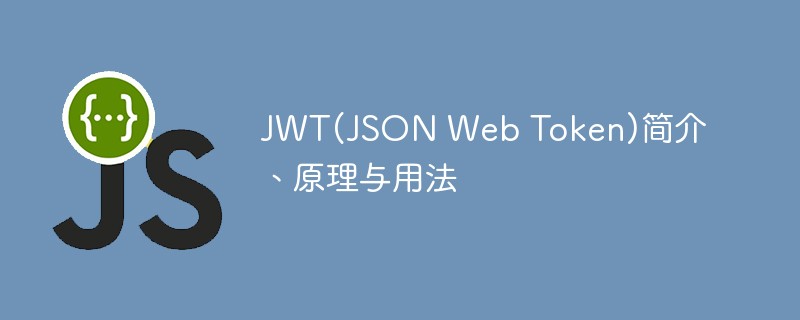
本篇文章给大家带来了关于JWT的相关知识,其中主要介绍了什么是JWT?JWT的原理以及用法是什么?感兴趣的朋友,下面一起来看一下吧,希望对大家有帮助。
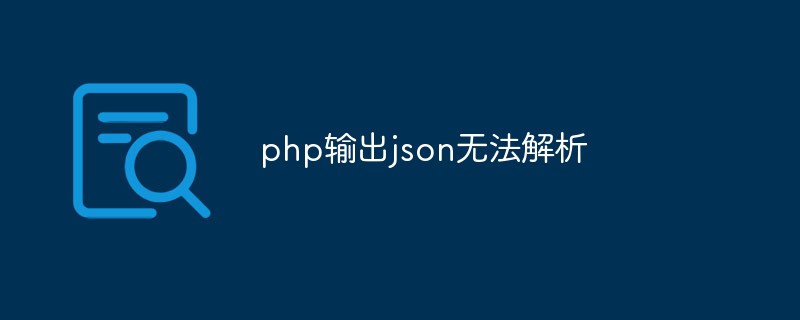
PHP作为一种常见的编程语言,在web开发中使用广泛,其与前端交互的方式也多种多样。其中,输出Json数据是一种常见的交互方式,但有时候会碰到Json无法解析的问题。为什么会出现无法解析的情况呢?下面列举了几个可能的原因。
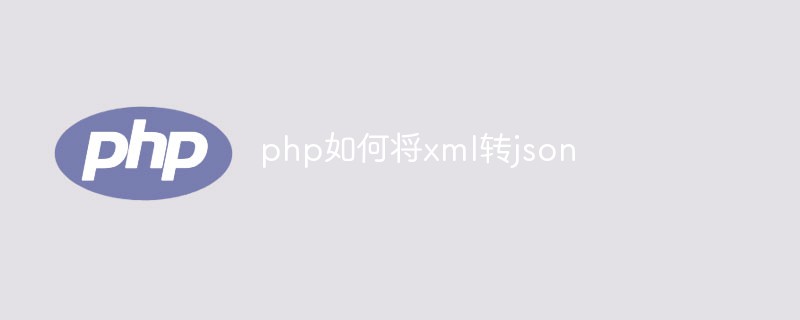
当我们处理数据时经常会遇到将XML格式转换为JSON格式的需求。PHP有许多内置函数可以帮助我们执行这个操作。在本文中,我们将讨论将XML格式转换为JSON格式的不同方法。
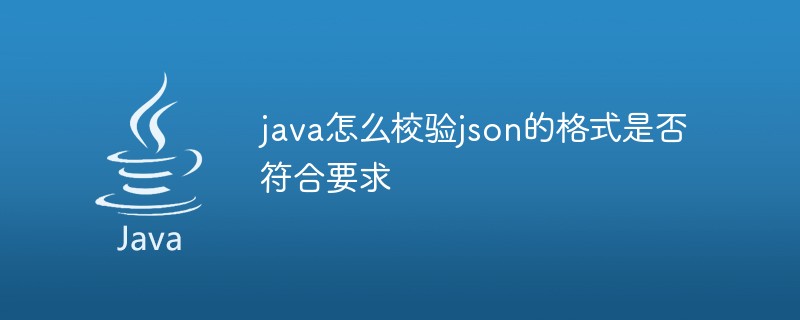
JSONSchemaJSONSchema是用于验证JSON数据结构的强大工具,Schema可以理解为模式或者规则。JsonSchema定义了一套词汇和规则,这套词汇和规则用来定义Json元数据,且元数据也是通过Json数据形式表达的。Json元数据定义了Json数据需要满足的规范,规范包括成员、结构、类型、约束等。JSONSchema就是json的格式描述、定义、模板,有了他就可以生成任何符合要求的json数据json-schema-validator在java中,对json数据格式的校验,使用
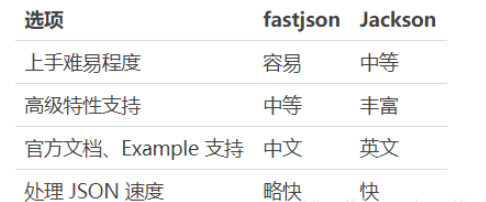
一、@RestController注解在SpringBoot中的Controller中使用@RestController注解即可返回JSON格式的数据。@RestController注解包含了@Controller和@ResponseBody注解。@ResponseBody注解是将返回的数据结构转换为JSON格式。@Target({ElementType.TYPE})@Retention(RetentionPolicy.RUNTIME)@Documented@Controller@Respons


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
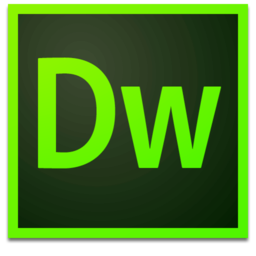
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
