In-depth understanding of what JavaScript closures are_Basic knowledge
1. Simple example
Let’s start with a classic mistake. There are several divs on the page. We want to bind an onclick method to them, so we have the following code
/span> 2 3
;
;/span> 1 2 3
>
Copy code
alert(i) ;
;
Copy code
The code is as follows:
2. Internal functions
Let’s start with some basic knowledge and first understand internal functions. An internal function is a function defined within another function. For example:
Copy code
function outerFn () {
functioninnerFn ( ) {}}
The code is as follows:
document.write( "Outer function
"); function innerFn() {
document.write("Inner function
");
However, if innerFn is called inside outerFn, it can run successfully:
function outerFn() {
document.write( "Outer function
");
function innerFn() {
document.write("Inner function
");
}
innerFn();
}
outerFn();
2.1 The Great Escape
JavaScript allows developers to pass functions like any type of data, that is, internal functions in JavaScript are able to escape the external functions that define them.
There are many ways to escape. For example, you can assign an internal function to a global variable:
var globalVar;
function outerFn() {
document.write("Outer function
");
function innerFn() {
document .write("Inner function
");
.
When calling outerFn, the global variable globalVar will be modified. At this time, its reference becomes innerFn. After that, calling globalVar is the same as calling innerFn. At this time, calling innerFn directly outside outerFn will still cause an error. This is because although the inner function escapes by saving the reference in a global variable, the name of this function still only exists in the scope of outerFn.
You can also get the internal function reference through the return value of the parent function
Copy code
"); function innerFn() {
");
return innerFn;
}
var fnRef = outerFn();
fnRef();
The global variable is not modified inside outerFn, but a reference to innerFn is returned from outerFn. This reference can be obtained by calling outerFn, and this reference can be saved in a variable.
The fact that internal functions can still be called by reference even after leaving the function scope means that JavaScript needs to retain the referenced function as long as there is a possibility of calling the internal function. Moreover, the JavaScript runtime needs to track all variables that reference this internal function until the last variable is discarded before the JavaScript garbage collector can release the corresponding memory space (the red part is the key to understanding closures).
After talking for a long time, I finally have something to do with closures. Closures refer to functions that have permission to access variables in the scope of another function. The common way to create closures is to create another function inside a function. It is the internal function we mentioned above, so what I just said is not nonsense, it is also related to closures^_^
Inner functions can also have their own variables, which are restricted to the scope of the inner function:
Copy code
function outerFn() {
document.write("Outer function
"); innerVar;
document.write("Inner functiont"); 🎜> }
var fnRef = outerFn();
var fnRef2 = outerFn();
fnRef();
fnRef2();
Whenever this inner function is called by reference or other means, a new innerVar variable is created, then incremented by 1, and finally displayed
Copy code
The code is as follows:
Copy code
The code is as follows:
"); ‐‐‐‐‐‐‐‐‐‐ f(); var fnRef2 = outerFn(); var fnRef2 = outerFn(); fnRef2(); Value of variable:
Copy code
The code is as follows:
Outer function
Inner function globalVar = 1
Inner function globalVar = 2
Outer function
Inner function globalVar = 3
Inner function globalVar = 4
function outerFn() {
var outerVar = 0;
document.write("Outer function
");
innerFn() function {
outerVar ;
document.write("Inner functiont"); 🎜> }
var fnRef = outerFn();
var fnRef2 = outerFn();
fnRef();
fnRef2();
The result this time is very interesting, perhaps beyond our expectations
Copy code
What we see is the combined effect of the previous two situations, through Each reference call to innerFn increments outerVar independently. That is to say, the second call to outerFn does not continue to use the value of outerVar, but creates and binds new outerVar instances in the scope of the second function call. The two counters are completely unrelated.
When an inner function is referenced outside the scope in which it is defined, a closure of the inner function is created. In this case, we call variables that are neither local variables of the internal function nor its parameters free variables, and call the calling environment of the external function a closed closure environment. Essentially, if an inner function references a variable located in an outer function, it authorizes that variable to be deferred. Therefore, when the external function call completes, the memory of these variables is not released (the last value is saved), and the closure still needs to use them.
3. Interaction between closures
When there are multiple internal functions, unexpected closures are likely to occur. We define an increasing function, the increment of this function is 2
Copy code
function outerFn() {
var outerVar = 0;
document.write("Outer function
");
function innerFn1() {
outerVar ;
document.write("Inner function 1t");
document.write("outerVar = " outerVar "
");
}
function innerFn2() {
outerVar = 2;
document.write("Inner function 2t");
document.write("outerVar = " outerVar "
");
}
return { "fn1": innerFn1, "fn2": innerFn2 };
}
var fnRef = outerFn();
fnRef.fn1();
fnRef.fn2();
fnRef.fn1();
var fnRef2 = outerFn();
fnRef2.fn1();
fnRef2.fn2();
fnRef2.fn1();
我们映射返回两个内部函数的引用,可以通过返回的引用调用任一个内部函数,结果:
Outer function
Inner function 1 outerVar = 1
Inner function 2 outerVar = 3
Inner function 1 outerVar = 4
Outer function
Inner function 1 outerVar = 1
Inner function 2 outerVar = 3
Inner function 1 outerVar = 4
innerFn1和innerFn2引用了同一个局部变量,因此他们共享一个封闭环境。当innerFn1为outerVar递增一时,久违innerFn2设置了outerVar的新的起点值,反之亦然。我们也看到对outerFn的后续调用还会创建这些闭包的新实例,同时也会创建新的封闭环境,本质上是创建了一个新对象,自由变量就是这个对象的实例变量,而闭包就是这个对象的实例方法,而且这些变量也是私有的,因为不能在封装它们的作用域外部直接引用这些变量,从而确保了了面向对象数据的专有性。
4.解惑
现在我们可以回头看看开头写的例子就很容易明白为什么第一种写法每次都会alert 4了。
for (var i = 0; i spans[i].onclick = function() {
alert(i);
}
}
The above code will be executed after the page is loaded. When the value of i is 4, the judgment condition is not established and the for loop is executed. However, because the onclick method of each span is an internal function at this time, i is closed. Reference, memory cannot be destroyed, the value of i will remain 4, and will not be recycled until the program changes it or all onclick functions are destroyed (actively assign the function to null or the page is unloaded). In this way, every time we click span, the onclick function will look up the value of i (the scope chain is a reference method), and if it is equal to 4, it will alert us. The second method is to use an immediately executed function to create a layer of closure. The function declaration is placed in parentheses and becomes an expression. After adding parentheses and parentheses, it is called. At this time, i is used as a parameter. Passed in, the function is executed immediately, and num saves the value of i each time.
After reading this, everyone must have some understanding of closures like me. Of course, if you fully understand it, you need to understand the execution environment and scope chain of the function ^_^
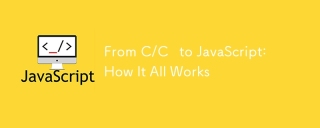
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
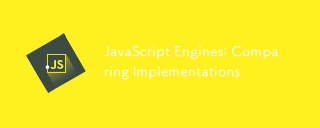
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
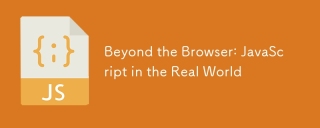
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
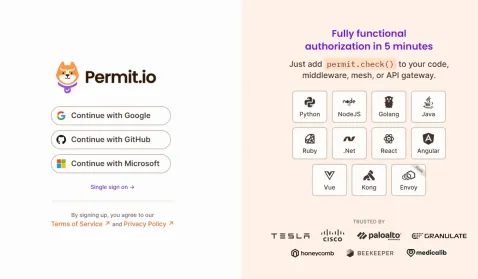
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
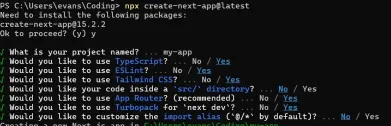
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
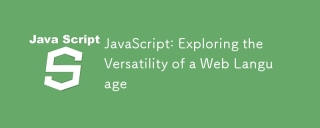
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
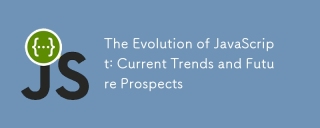
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
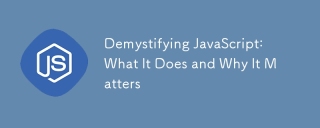
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
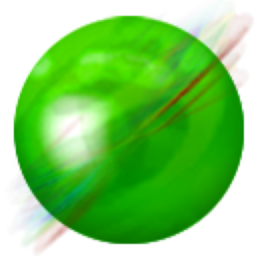
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
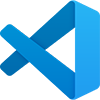
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment