


Discussion on the use of typeof and instanceof operators in JavaScript_Basic knowledge
When writing JavaScript code, the two operators typeof and instanceof are used from time to time and are must-use. but! It is always difficult to get the desired results directly when using them. It is generally said that "these two operators are perhaps the biggest design flaw in JavaScript, because it is almost impossible to get the desired results from them"
typeof
Explanation: typeof returns a string of the data type of an expression, and the return result is the basic data type of js, including number, boolean, string, object, undefined, function.
Judging from the description, there seems to be no problem.
The following code writes a numerical variable, and the result after typeof is "number".
var a = 1;
console.log( typeof(a)); //=>number
If you use the constructor new of type Number to create a variable, the result after typeof is "object".
var a = new Number(1);
console.log(typeof(a)); //=>object
The above two output results seem to have no problem, which seems to be a matter of course from the book. Because javascript is designed this way.
But! The problem is that since typeof is called, it should accurately return the type of a variable, whether it is created directly with a value or with a type constructor, otherwise! What else should I use you for?
So for:
var a = 1;
var b = new Number(1);
Accurately speaking, the types of a and b variables should be Number to get the desired result.
The accurate type information is stored in the value of the variable's internal property [[Class]], which is obtained by using the method toString defined on Object.prototype.
Get type information:
var a = 1;
var b = new Number(1);
console.log(Object.prototype.toString.call(a));
console.log(Object.prototype.toString.call(b ));
Output:
[object Number]
[object Number]
Isn’t it already very straightforward? Let’s do a little processing and get the direct result:
var a = 1;
var b = new Number(1);
console.log( Object.prototype.toString.call(a).slice(8,-1));
console.log(Object.prototype.toString.call(b).slice(8,-1));
Output:
Number
Number
This is the desired result.
For better use, we encapsulate a method to determine whether a variable is of a certain type:
function is(obj,type) {
var clas = Object.prototype.toString.call(obj).slice(8, -1);
return obj !== undefined && obj !== null && clas === type;
}
I have defined some variables and tested them. Let’s take a look at their typeof output first:
var a1=1;
var a2=Number(1 );
var b1="hello";
var b2=new String("hello");
var c1=[1,2,3];
var c2=new Array(1 ,2,3);
console.log("a1's typeof:" typeof(a1));
console.log("a2's typeof:" typeof(a2));
console.log(" b1's typeof:" typeof(b1));
console.log("b2's typeof:" typeof(b2));
console.log("c1's typeof:" typeof(c1));
console .log("c2's typeof:" typeof(c2));
Output:
a1's typeof:number
a2's typeof:object
b1's typeof:string
b2's typeof:object
c1's typeof:object
c2's typeof:object
使用する新しく作成した関数は次のとおりです:
console.log("a1 は番号:" is(a1,"Number"));
console.log("a2 は番号:" is(a2,"Number")); .log( "b1 は文字列です:" is(b1,"String"));
console.log("b2 は文字列です:" is(b2,"String")); c1 は配列 :" is(c1,"Array"));
console.log("c2 は配列:" is(c2,"Array"));
出力:
a1 は数値: true
a2 は Number:true
b1 は String:true
b2 は String:true
c1 は Array:true
c2 は Array:true
注: typeof 実際の用途は、変数が定義されているか、値が割り当てられているかを検出することです。
instanceof
説明: オブジェクトが特定のデータ型であるかどうか、または変数がオブジェクトのインスタンスであるかどうかを判断します。 instanceof 演算子も、2 つの組み込み型変数の比較に使用される場合には無力であり、結果にも満足できません。
console.log(new String("abc") インスタンスオブ String); // true
console.log( new String( "abc")instanceof Object); // true
カスタム オブジェクトを比較する場合にのみ関係を正確に反映します。
Man.prototype = new Person();
console.log(new Man()instanceofMan) // true
console.log(new Man()instanceofMan); ; // 真
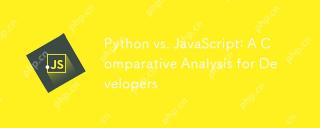
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
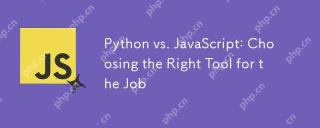
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
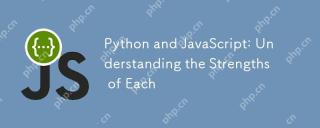
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
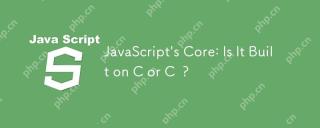
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
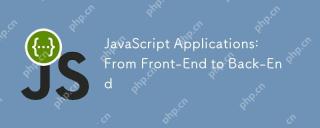
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
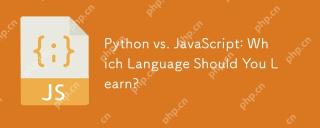
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
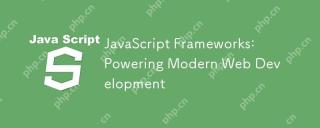
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
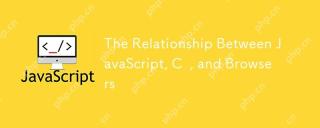
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
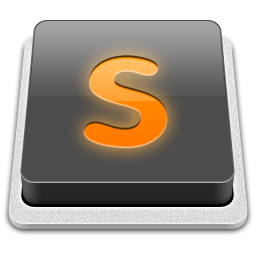
SublimeText3 Mac version
God-level code editing software (SublimeText3)
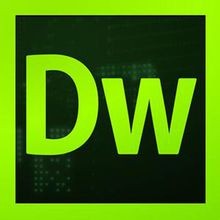
Dreamweaver CS6
Visual web development tools
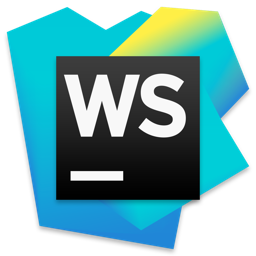
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
