Django template
In the previous chapter we used django.http.HttpResponse() to output "Hello World!". This method mixes data and views, which does not conform to Django's MVC idea.
In this chapter, we will introduce the application of Django templates in detail. A template is a text that is used to separate the presentation form and content of a document.
Template application example
Following the project in the previous chapter, we will create the templates directory under the HelloWorld directory and create the hello.html file. The entire directory structure is as follows:
HelloWorld/ |-- HelloWorld | |-- __init__.py | |-- __init__.pyc | |-- settings.py | |-- settings.pyc | |-- urls.py | |-- urls.pyc | |-- view.py | |-- view.pyc | |-- wsgi.py | `-- wsgi.pyc |-- manage.py `-- templates `-- hello.html
hello.html file code is as follows:
<h1>{{ hello }}</h1>
From the template we know that variables use double brackets.
Next we need to explain the path of the template file to Django, modify HelloWorld/settings.py, and modify the DIRS in TEMPLATES to [BASE_DIR+"/templates",], as shown below:
TEMPLATES = [ { 'BACKEND': 'django.template.backends.django.DjangoTemplates', 'DIRS': [BASE_DIR+"/templates",], 'APP_DIRS': True, 'OPTIONS': { 'context_processors': [ 'django.template.context_processors.debug', 'django.template.context_processors.request', 'django.contrib.auth.context_processors.auth', 'django.contrib.messages.context_processors.messages', ], }, }, ]
We now modify view.py and add a new object for submitting data to the template:
# -*- coding: utf-8 -*- #from django.http import HttpResponse from django.shortcuts import render def hello(request): context = {} context['hello'] = 'Hello World!' return render(request, 'hello.html', context)
As you can see, we use render here to replace the previously used HttpResponse. render also uses a dictionary context as a parameter.
context The key value "hello" of the element in the dictionary corresponds to the variable "{{ hello }}" in the template.
Visit http://192.168.45.3:8000/hello/ again, you can see the page:
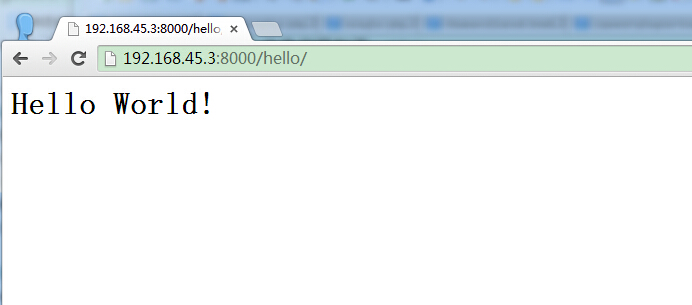
In this way, we have completed using the template to output data, thereby realizing the data Detached from the view.
Next we will introduce in detail the grammar rules commonly used in templates.
Django template tag
if/else tag
The basic syntax format is as follows:
{% if condition %} ... display {% endif %}
or:
{% if condition1 %} ... display 1 {% elif condiiton2 %} ... display 2 {% else %} ... display 3 {% endif %}
according to Conditional judgment whether to output. if/else supports nesting.
{% if %} tag accepts and, or or not keywords to judge multiple variables, or to invert variables (not), for example:
{% if athlete_list and coach_list %} athletes 和 coaches 变量都是可用的。 {% endif %}
for tag
{% for %} allows us to iterate over a sequence.
Similar to Python's for statement, the loop syntax is for X in Y , where Y is the sequence to be iterated and X is the variable name used in each specific loop.
In each loop, the template system will render all content between {% for %} and {% endfor %}.
For example, given a list of athletes in the athlete_list variable, we can use the following code to display the list:<ul> {% for athlete in athlete_list %} <li>{{ athlete.name }}</li> {% endfor %} </ul>
Add a reversed to the label so that the list is iterated in reverse:
{% for athlete in athlete_list reversed %} ... {% endfor %}
You can nest the {% for %} tag:
{% for athlete in athlete_list %} <h1>{{ athlete.name }}</h1> <ul> {% for sport in athlete.sports_played %} <li>{{ sport }}</li> {% endfor %} </ul> {% endfor %}
ifequal/ifnotequal tag
{% ifequal %} tag compares two values when they are equal , displays all values between {% ifequal %} and {% endifequal %}.
The following example compares two template variables user and currentuser:
{% ifequal user currentuser %} <h1>Welcome!</h1> {% endifequal %}
Similar to {% if %}, {% ifequal %} supports the optional {% else%} tag: 8
{% ifequal section 'sitenews' %} <h1>Site News</h1> {% else %} <h1>No News Here</h1> {% endifequal %}
Comment tag
Django comments use {# #}.
{# 这是一个注释 #}
Filter
The template filter can modify the variable before it is displayed. The filter uses the pipe character, as follows:
{{ name|lower }}
{{ name }} After the variable is processed by the filter lower, the document uppercase conversion text is lowercase.
Filter pipes can be *socketed*, that is to say, the output of one filter pipe can also be used as the input of the next pipe:
{{ my_list|first|upper }}
The above example will first element and Convert to uppercase.
Some filters have parameters. Filter arguments follow a colon and are always enclosed in double quotes. For example:
{{ bio|truncatewords:"30" }}
This will display the first 30 words of the variable bio.
Other filters:
addslashes : Adds a backslash before any backslash, single quote, or double quote.
date: Format the date or datetime object according to the specified format string parameter, example:
{{ pub_date|date:"F j, Y" }}
length: Return the length of the variable.
include tag
{% include %} tag allows the content of other templates to be included in the template.
Both of the following two examples include the nav.html template:
{% include "nav.html" %}
Template inheritance
Templates can be reused through inheritance.
Next, we first create the base.html file in the templates directory of the previous project. The code is as follows:
<html> <head> <title>Hello World!</title> </head> <body> <h1>Hello World!</h1> {% block mainbody %} <p>original</p> {% endblock %} </body> </html>
In the above code, the block tag named mainbody can be replaced by successors. fallen part.
All {% block %} tags tell the template engine that subtemplates can override these parts.
hello.html inherits base.html and replaces specific blocks. The modified code of hello.html is as follows:
{% extends "base.html" %} {% block mainbody %} <p>继承了 base.html 文件</p> {% endblock %}
The first line of code indicates that hello.html inherits the base.html file . As you can see, the block tag with the same name here is used to replace the corresponding block in base.html.
Revisit the address http://192.168.45.3:8000/hello/, the output result is as follows:
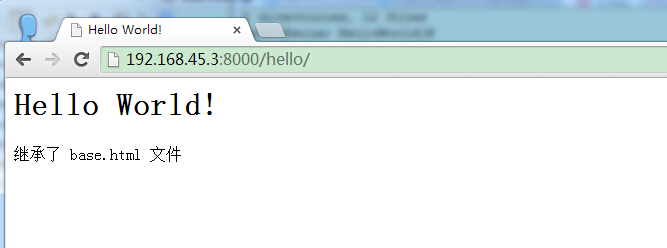