Django creates the first project
In this chapter we will introduce Django management tools and how to use Django to create projects. For the first project, we use HelloWorld to command the project.
Django Administration Tools
After installing Django, you should now have the administration tool django-admin.py available. We can use django-admin.py to create a project:
We can take a look at the command introduction of django-admin.py:
[root@solar ~]# django-admin.py Usage: django-admin.py subcommand [options] [args] Options: -v VERBOSITY, --verbosity=VERBOSITY Verbosity level; 0=minimal output, 1=normal output, 2=verbose output, 3=very verbose output --settings=SETTINGS The Python path to a settings module, e.g. "myproject.settings.main". If this isn't provided, the DJANGO_SETTINGS_MODULE environment variable will be used. --pythonpath=PYTHONPATH A directory to add to the Python path, e.g. "/home/djangoprojects/myproject". --traceback Raise on exception --version show program's version number and exit -h, --help show this help message and exit Type 'django-admin.py help <subcommand>' for help on a specific subcommand. Available subcommands: [django] check cleanup compilemessages createcachetable ……省略部分……
Create the first project
Use django-admin.py to create the HelloWorld project:
django-admin.py startproject HelloWorld
After the creation is completed, we can view the directory structure of the project:
[root@solar ~]# cd HelloWorld/ [root@solar HelloWorld]# tree . |-- HelloWorld | |-- __init__.py | |-- settings.py | |-- urls.py | `-- wsgi.py `-- manage.py
Directory description:
HelloWorld: The container of the project.
manage.py: A useful command line tool that allows you to interact with this Django project in various ways.
HelloWorld/__init__.py: An empty file that tells Python that the directory is a Python package.
HelloWorld/settings.py: Settings/configuration for this Django project.
HelloWorld/urls.py: The URL declaration for this Django project; a "directory" of websites powered by Django.
HelloWorld/wsgi.py: Entrance to a WSGI-compatible web server to run your project.
Next we enter the HelloWorld directory and enter the following command to start the server:
python manage.py runserver 0.0.0.0:8000
0.0.0.0 allows other computers to connect to the development server, and 8000 is the port number. If not specified, the port number defaults to 8000.
Enter your server's IP and port number in the browser. If it starts normally, the output will be as follows:
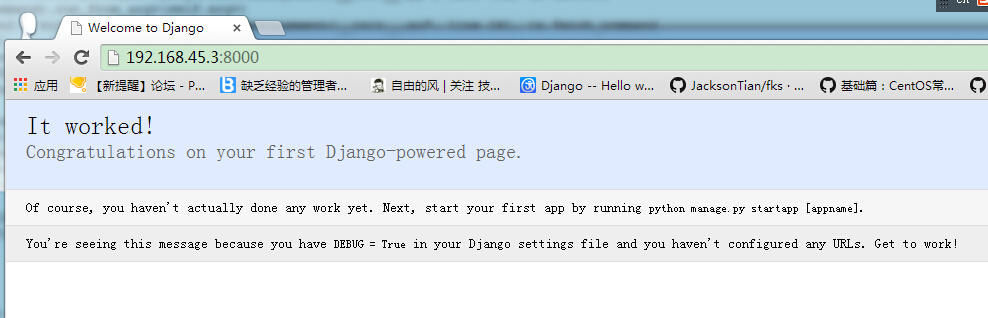
View and URL configuration
In the previously created HelloWorld Create a new view.py file in the HelloWorld directory and enter the code:
from django.http import HttpResponse def hello(request): return HttpResponse("Hello world ! ")
Next, bind the URL and the view function. Open the urls.py file, delete the original code, and copy and paste the following code into the urls.py file:
from django.conf.urls import * from HelloWorld.view import hello urlpatterns = patterns("", ('^hello/$', hello), )
The entire directory structure is as follows:
[root@solar HelloWorld]# tree . |-- HelloWorld | |-- __init__.py | |-- __init__.pyc | |-- settings.py | |-- settings.pyc | |-- urls.py # url 配置 | |-- urls.pyc | |-- view.py # 添加的视图文件 | |-- view.pyc # 编译后的视图文件 | |-- wsgi.py | `-- wsgi.pyc `-- manage.py
After completion, start the Django development server, and Open the browser and visit:
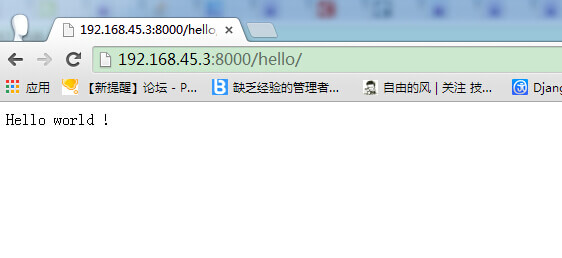
Note: If there are changes to the code in the project, the server will automatically monitor the code changes and automatically reload, so if you If the server has already been started, there is no need to restart it manually.