1. 属性与方法重载
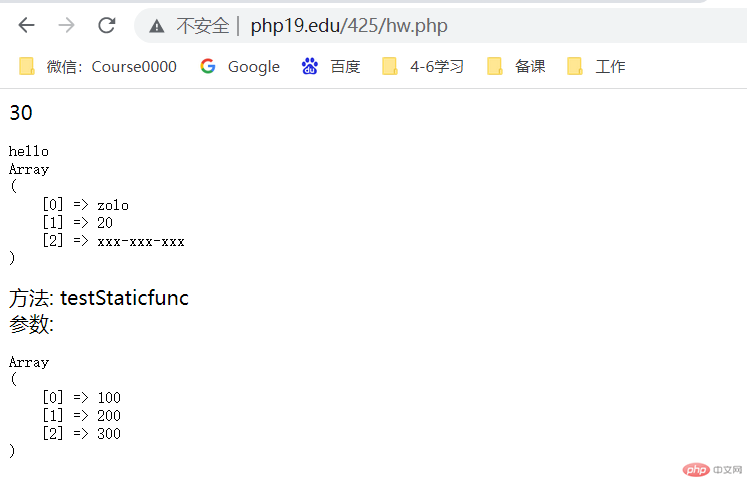
<?php
/**
* 1. 属性重载: __get(), __set()
* 2. 方法属性: 普通__call(), 静态__callStatic()
*/
class User{
// 属性
private $data = [
'age' => '',
'name'=> 'zolo',
'address'=>'',
];
//所有的重载方法都必须被声明为 public
public function __get($arg){
if(array_key_exists($arg,$this->data)){
return $this->data[$arg];
}else{
return "{$arg}不存在";
}
}
//PHP所提供的“重载”(overloading)是指动态地“创建”类属性和方法
//我们是通过魔术方法(magic methods)来实现的
public function __set($arg,$argv)
{
if(array_key_exists($arg,$this->data)){
if($arg === 'age'){
//echo is_numeric($argv);
if($argv > 18){
return $this->data[$arg] = $argv;
}else{
echo "{$arg}不合法";
}
}else{
return $this->data[$arg] = $argv;
}
}else{
echo "参数{$arg}不合法";
}
}
// 方法拦截器
// $name: 方法名, $args: 传给方法的参数
public function __call($funcname,$funcargs){
printf('<pre>%s<br>%s</pre>',$funcname,print_r($funcargs,true));
}
public static function __callStatic($name, $arguments){
printf('方法: %s<br>参数:<pre>%s</pre>', $name, print_r($arguments, true));
}
}
$u = new User;
// echo $u->name.'<br>';
// $u->address = '虹口区北京路555号';
$u->age = 30;
echo $u->age.'<br>';
// echo $u->address.'<br>';
$u->hello('zolo',20,'xxx-xxx-xxx');
User::testStaticfunc(100, 200,300);
2. 命名空间
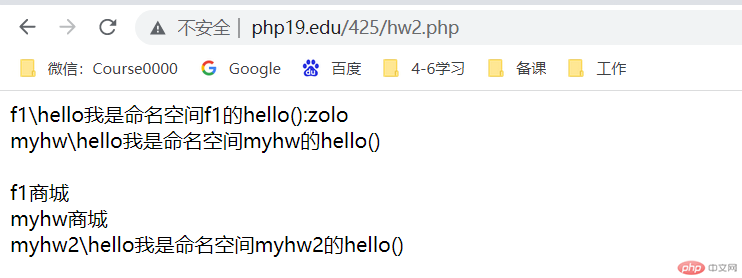
<?php
// 命名空间,使用namespace声明,必须写到第一行
/**
* 命名空间: 解决了全局成员的命名冲突
* 全局成员: 类/接口, 常量 , 函数,不能重复声明
*/
namespace myhw;
require __DIR__.'/inc/f1.php';
const APP = 'myhw商城';
function hello(){
return __FUNCTION__.'我是命名空间myhw的hello()<br>';
}
echo \f1\hello('zolo');
echo hello();
echo '<br>';
echo \f1\APP;//打印商城
echo '<br>';
echo APP;
echo '<br>';
namespace myhw2;
function hello(){
return __FUNCTION__.'我是命名空间myhw2的hello()<br>';
}
echo hello();
3.类自动加载器
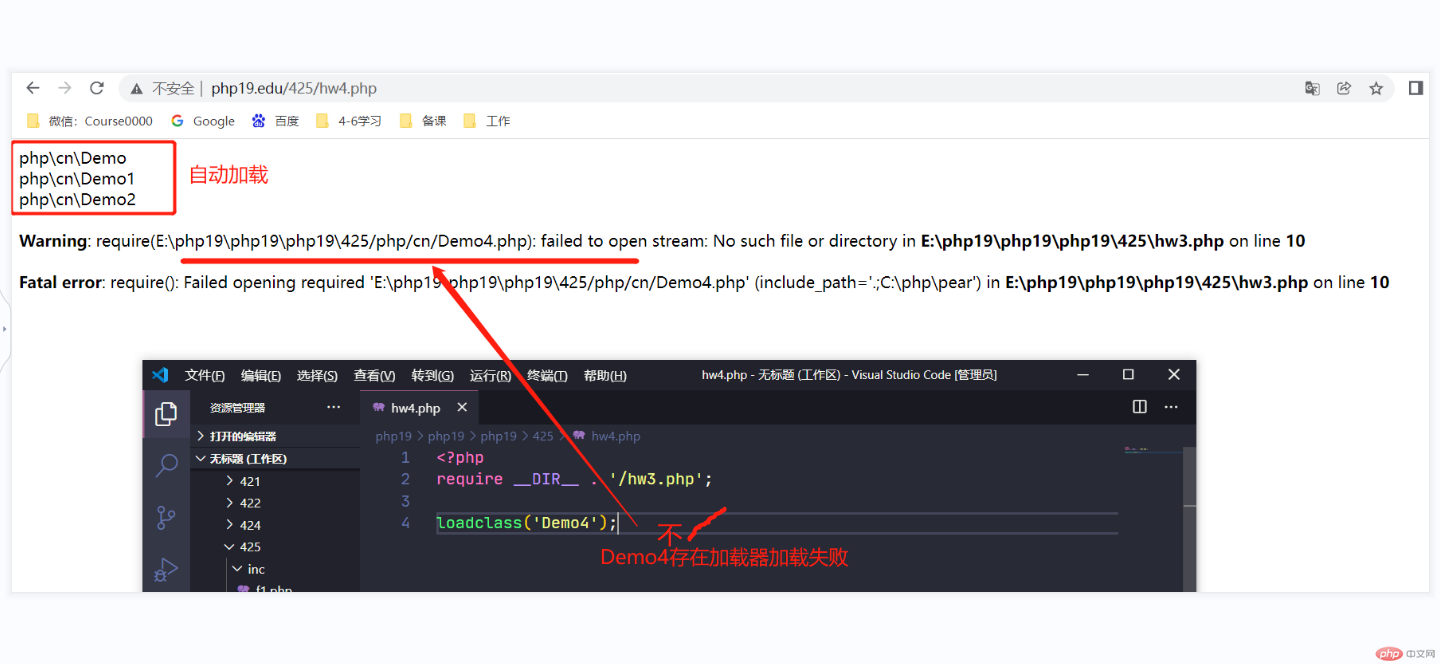
function loadclass($arg){
require __DIR__.'/php/cn/'.$arg.'.php';
}
loadclass('Demo');
loadclass('Demo1');
loadclass('Demo2');
<?php
require __DIR__ . '/hw3.php';
loadclass('Demo4');