作业内容:
1. 实例演示fetch api, async,await的使用
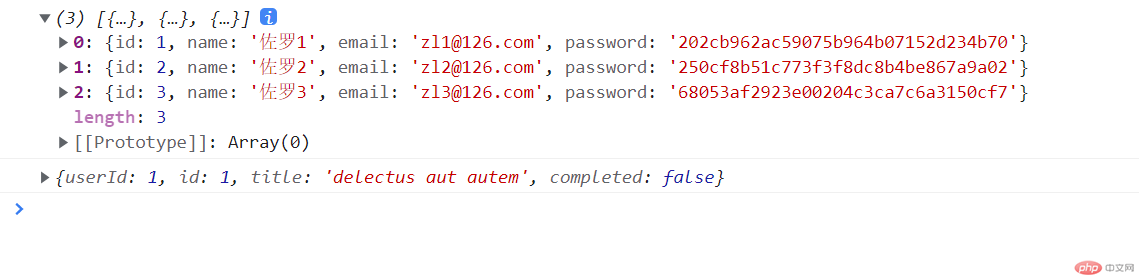
// cps :尾部调用, 让用户产生一个错觉, 代码是同步执行,并不异步
// 异步操作:定时器, 文件操作, 网络操作
function createdFile(success, failure) {
success();
failure();
}
function success(msg) {
return "success" + msg;
}
function failure(msg) {
return "error" + msg;
}
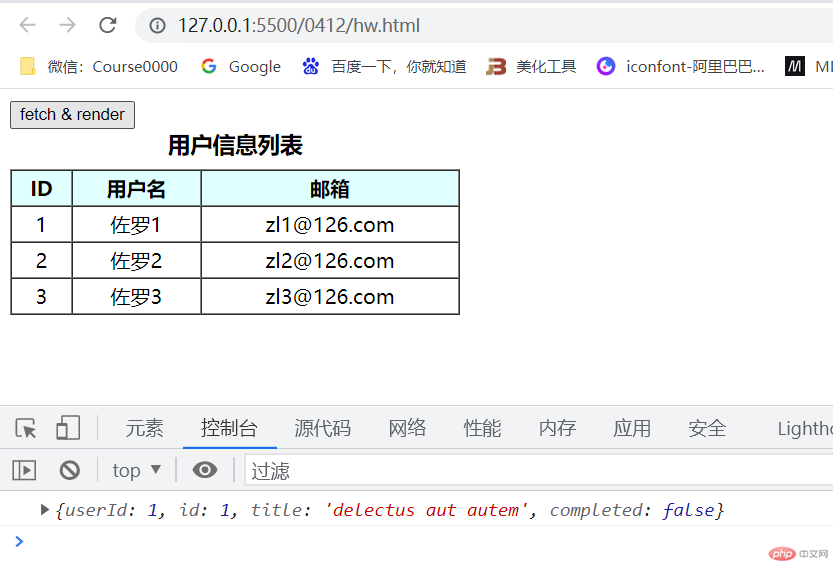
//createdFile().then(success).then(json).catch(failure)
fetch("https://jsonplaceholder.typicode.com/todos/1")
.then((response) => response.json())
.then((json) => console.log(json));
url = "http://tptest.com/users.php";
async function getInfo(btn,url) {
response = await fetch(url)
result = await response.json();
render(result, btn)
}
//模块
<script type="module">
// 模块: 就是一个外部的js
// 传统js,引入外部js,共享一个全局空间
// 模块有自己的独立作用域
// 导入模块
// import 成员列表 from 模块路径
import * as m from "./1.js";
console.log(m.email);
console.log(new m.Demo().show());
</script>
2. npm 安装与删除包的常用操作
"axios": "^0.26.1"
0: 大版本
26:小版本
1: 补丁
^: 仅允许小版本更新
*: 允许大版本更新
~: 仅允许修复补丁
npm i axios -S: 生产依赖
npm i axios -D:开发依赖
npm root -g: 查看全局模块的路径
Windows PowerShell
PS E:\php19\0413> node 2.js
admin@php.cn
PS E:\php19\0413> npm -v
PS E:\php19\0413> npm init -y
Wrote to E:\php19\0413\package.json:
{
"name": "0413",
"version": "1.0.0",
"description": "",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
PS E:\php19\0413> npm install axios
added 2 packages, and audited 3 packages in 7s
1 package is looking for funding
run `npm fund` for details
found 0 vulnerabilities
PS E:\php19\0413> npm uninstall axios
removed 2 packages, and audited 1 package in 603ms
found 0 vulnerabilities
3. node中的模块声明,导出与导入
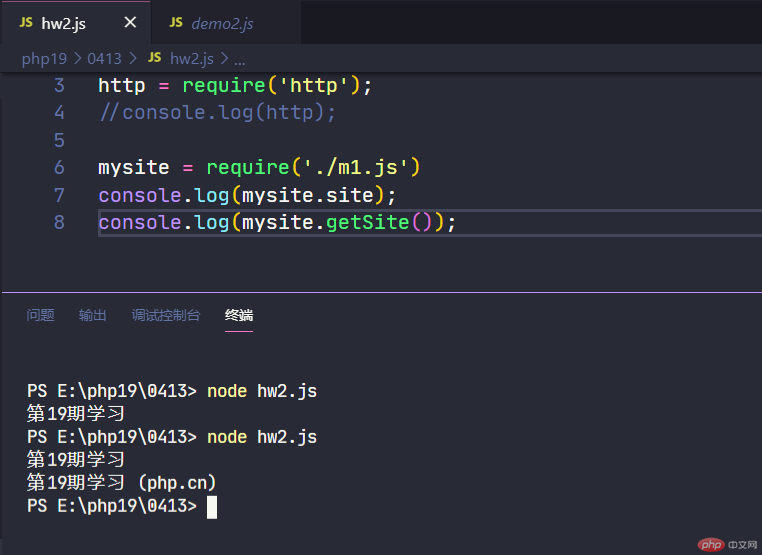
const { site } = require('./m1');
//require导入
http = require('http');
//console.log(http);
mysite = require('./m1.js')
console.log(mysite.site);
console.log(mysite.getSite());
//常用导出
module.exports = {
site: '第19期学习',
getSite() {
return this.site + ' (php.cn)';
},
};
//文件模块
fs = require('fs')
fs.readFile('./test.txt', function (err, data) {
if (err) {
return false
} else {
console.log(String(data));
console.log(data.toString());
}
})