HTML部分
<script>
// 1.函数参数
// 1-1. 参数不足: 默认参数
// 参数不足的情况下,给形参加上默认值 = 0
console.log(`-----------参数不足分割线-----------`);
function fn(x, y = 0) {
console.log(x - y);
}
console.log(fn(9));
console.log(`----------参数过多分割线------------`);
// 1-22. 参数过多: ...剩余参数
let x = (a, b, ...c) => console.log(a, b, c);
console.log(x(9, 8, 7, 6, 5));
// 获取函数中的数组,离散值
let arr = [9, 8, 7, 6, 5];
console.log(...arr);
// 可以将实参里放入数组
console.log(x(...arr));
//将数组放在形参中,以回调的方式,输出多个数据
x = (...arr) => arr.reduce((a, c) => a + c);
console.log(x(14, 64, 478, 417, 174, 147, 64571, 1));
console.log(`----------返回值分割线------------`);
//2.返回值
//2.1单个返回值
let fc = (a, b) => console.log(a + b);
console.log(fc(996, 007));
console.log(`----------返回一个引用类型的复合值--数组------------`);
// 2.2返回一个引用类型的复合值
// 数组
let fd = () => [1, 2, 3, 9, 8, 7];
let arrfd = fd();
console.log(arrfd);
console.log(`----------返回一个引用类型的复合值--对象------------`);
// 对象
let fg = () => ({
id: 996,
admin: '隔壁老王',
userName: '你王叔',
});
addfd = fg();
console.log(addfd);
// 4.模板字面量
// 4-1字面量
console.log(`----------模板字面量------------`);
let name = '---马化腾';
console.log(`充八万你会更强! ${name}`);
console.log(`----------模板函数------------`);
// 4-2模板函数
mlt` 麻辣烫份量:${10}} 价格¥:${6}`;
function mlt(strings, ...args) {
console.log(strings);
console.log(args);
console.log(args[0] * args[1]);
}
// 模板函数的函数名就是以模板字面量为值的标识符
// 模板函数形参里的第一个参数是模板字面量,中的字符串
// 模板函数形参里的第二个参数是模板字面量中的'插值',组成的数组
</script>
展示
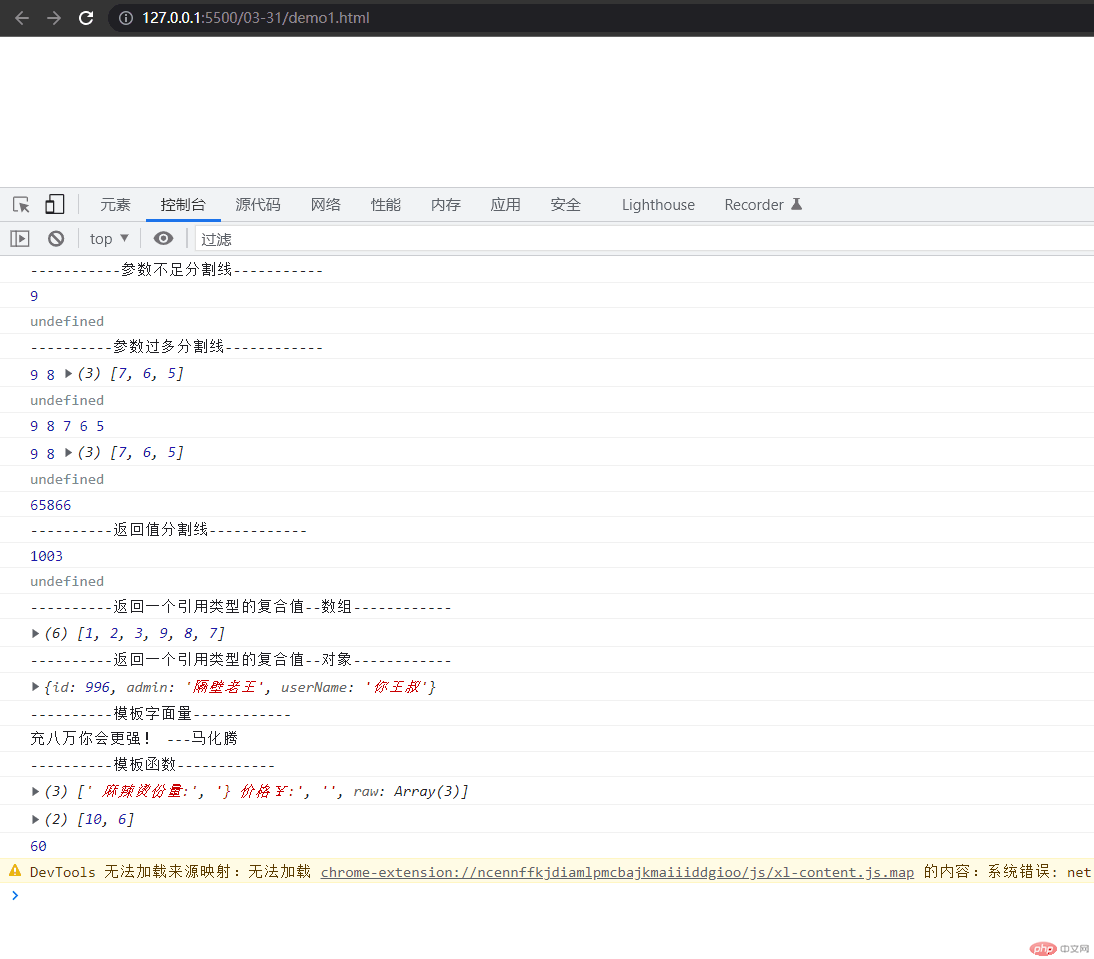