1.表格的学习,以购物车为例,详细如下:
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>购物车</title>
<style type="text/css">
/* 浏览器默认外边距8像素,全部初始化0 */
* {
padding: 0;
margin: 0;
}
.table {
width: 70%;
/* 表格边框线实心,去掉间隙 */
border-collapse: collapse;
/* 表格文字水平居中对齐 */
text-align: center;
/* 表格水平居中对齐 */
margin: auto;
}
.table caption {
/* 标题上下外边距10像素,左右自动,也就是水平居中 */
margin: 10px auto;
font-size: 20px;
}
.table tr th,
.table tr td {
border-bottom: 1px solid #efef;
/* 字体显示更细 */
font-weight: lighter;
/* 表格内容内边距10像素 */
padding: 10px;
}
/* 表格头部第一个设置背景颜色 */
.table thead tr:first-child {
background: lightgreen;
}
/* 表格内容主题,分组后偶数行显示背景颜色,也就是表格隔行换色 */
.table tbody tr:nth-of-type(even) {
background: #eeeeee;
}
/* 光标移到表格行上后,表格背景颜色改变 */
.table tbody tr:hover {
background: palegoldenrod;
}
.table tfoot {
font-size: 18px;
color: coral;
}
/* body内容分组后,第一个div设置宽度和外边距 */
body div:first-of-type {
width: 70%;
/* 外边距:上10像素,下0像素,左右边距自动 */
margin: 10px auto 0px;
}
button {
width: 90px;
height: 35px;
/* 这个是圆角样式 */
border-radius: 4px;
color: #ffffff;
background: darkcyan;
/* 这个是去掉点击后的外边框虚线 */
outline: none;
float: right;
}
/* 光标移上去样式 */
button:hover {
/* 光标移上去后,改变光标形状,pointer表示小手形状 */
cursor: pointer;
background: darkolivegreen;
font-size: 18px;
}
</style>
</head>
<body>
<table class="table">
<caption>
购物车
</caption>
<thead>
<tr>
<th>商品编码</th>
<th>商品名称</th>
<th>数量</th>
<th>单价/元</th>
<th>金额</th>
</tr>
</thead>
<tbody>
<tr>
<td>NO2020061801</td>
<td>小米笔记本</td>
<td>1</td>
<td>7899.00</td>
<td>7899.00</td>
</tr>
<tr>
<td>NO2020061802</td>
<td>鼠标垫</td>
<td>2</td>
<td>8.00</td>
<td>16.00</td>
</tr>
<tr>
<td>NO2020061803</td>
<td>毛峰茶</td>
<td>1</td>
<td>20.00</td>
<td>20.00</td>
</tr>
<tr>
<td>NO2020061804</td>
<td>农夫山泉</td>
<td>1</td>
<td>2.00</td>
<td>2.00</td>
</tr>
</tbody>
<tfoot>
<tr>
<!-- colspan表示横向合并单元格,rowspan表示纵向合并单元格,后面跟上合并单元格数量,并且删除对应数量的单元格 -->
<td colspan="2">合计:</td>
<td>5</td>
<td> </td>
<td>7937.00</td>
</tr>
</tfoot>
</table>
<div>
<button>结算</button>
</div>
</body>
</html>
运行结果截图:
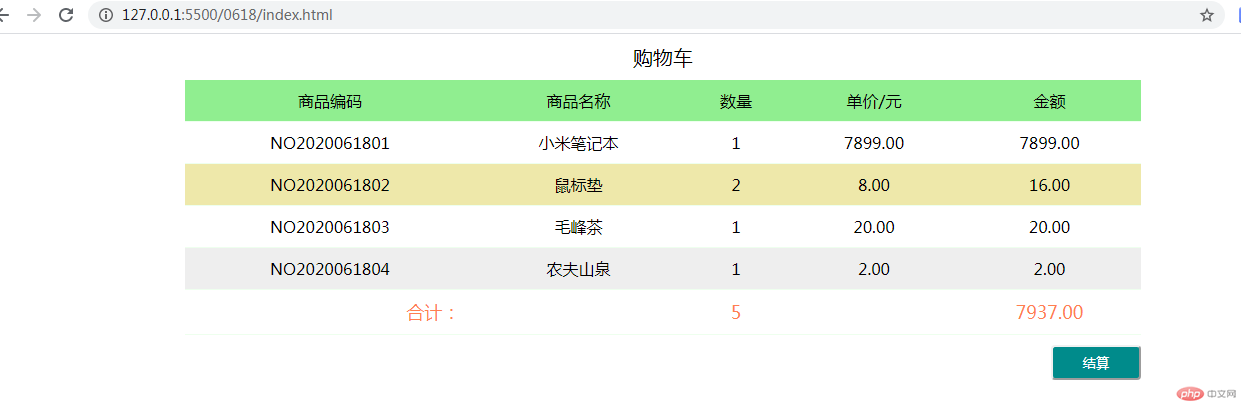
2.表单学习:
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>提交表单</title>
<style type="text/css">
/* 浏览器默认外边距8像素,全部初始化0 */
* {
padding: 0;
margin: 0;
}
input,
textarea {
outline: none;
padding: 0 10px;
border-radius: 5px;
border: none;
}
textarea {
line-height: 25px;
}
input[type="text"],
input[type="password"],
input[type="email"] {
height: 25px;
line-height: 25px;
}
input[type="radio"],
input[type="checkbox"] {
margin-left: 10px;
margin-right: 5px;
}
input[type="button"] {
background: mediumspringgreen;
color: navy;
padding: 5px;
cursor: pointer;
}
.regbox {
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
font-weight: lighter;
}
.applyBox {
width: 500px;
color: #666;
}
.applyBox .title {
text-align: center;
font-size: 20px;
margin: 10px 0px;
}
.forminfo fieldset {
font-size: 14px;
padding: 10px;
margin-bottom: 10px;
border-radius: 10px;
background: linen;
}
.forminfo fieldset:last-of-type {
margin-bottom: 0px;
}
.forminfo fieldset div:not(:last-of-type) {
margin-bottom: 10px;
}
.forminfo fieldset div label:first-of-type {
width: 80px;
display: inline-block;
}
.applyBox .btnBox {
text-align: center;
}
.btnBox .submitBtn {
width: 90px;
height: 35px;
/* 这个是圆角样式 */
border-radius: 4px;
color: #ffffff;
background: darkcyan;
/* 这个是去掉点击后的外边框虚线 */
outline: none;
margin-top: 10px;
}
/* 光标移上去样式 */
.btnBox .submitBtn:hover {
/* 光标移上去后,改变光标形状,pointer表示小手形状 */
cursor: pointer;
background: darkolivegreen;
font-size: 18px;
}
</style>
</head>
<body>
<div class="regbox">
<form action="">
<div class="applyBox">
<div class="title">用户注册</div>
<div class="forminfo">
<fieldset>
<legend>基本信息(必填)</legend>
<div>
<!-- for的值要与input的id对应,这样点击lable也能直接点中input -->
<!-- required只在submit提交表单时生效,可以提示必录 -->
<!-- autofocus默认光标选中 -->
<label for="userName">用户名称:</label>
<input
type="text"
id="userName"
name="userName"
placeholder="请输入用户名"
required
autofocus
/>
</div>
<div>
<label for="email">邮箱号:</label>
<input
type="email"
id="email"
name="email"
placeholder="请输入邮箱号"
required
/>
</div>
<div>
<label for="password">密码:</label>
<input
type="password"
id="password"
name="password"
placeholder="******"
required
/>
<input type="button" data-showid="password" value="显示密码" />
</div>
<div>
<label for="confitmPwd">确认密码:</label>
<input
type="password"
id="confitmPwd"
name="confitmPwd"
placeholder="******"
required
/>
<input
type="button"
data-showid="confitmPwd"
value="显示密码"
/>
</div>
</fieldset>
<fieldset>
<legend>扩展信息(选填)</legend>
<div>
<label for="birthday">生日:</label>
<input type="date" id="birthday" name="birthday" />
</div>
<div>
<label for="gender">性别:</label>
<input type="radio" id="male" name="sex" value="0" /><label
for="male"
>男</label
>
<input type="radio" id="female" name="sex" value="1" /><label
for="female"
>女</label
>
<input type="radio" id="gender" name="sex" value="2" /><label
for="gender"
>保密</label
>
</div>
<div>
<label for="read">爱好:</label>
<input type="checkbox" id="read" name="like" value="0" />
<label for="read">阅读</label>
<input type="checkbox" id="friends" name="like" value="1" />
<label for="friends">交友</label>
<input type="checkbox" id="game" name="like" value="2" />
<label for="game">游戏</label>
</div>
<div>
<label for="phone">手机号:</label>
<input
type="text"
id="phone"
name="phone"
placeholder="请输入11位手机号"
/>
</div>
</fieldset>
<fieldset>
<legend>其他</legend>
<div>
<label for="userimg">用户头像:</label>
<input type="file" id="userimg" name="userimg" />
</div>
<div>
<label for="introduce" style="vertical-align: top;"
>个人介绍:</label
>
<textarea
name="introduce"
id="introduce"
cols="30"
rows="3"
placeholder="请输入个人介绍"
></textarea>
</div>
</fieldset>
</div>
<div class="btnBox">
<button type="submit" class="submitBtn">提交</button>
</div>
</div>
</form>
</div>
</body>
<script>
//给显示密码按钮添加事件
document
.querySelectorAll('input[type="button"]')[0]
.addEventListener("click", showPwd);
document
.querySelectorAll('input[type="button"]')[1]
.addEventListener("click", showPwd);
function showPwd(e) {
let id = e.target.dataset.showid;
let objtype = document.getElementById(id).type;
if (objtype === "text") {
document.getElementById(id).type = "password";
e.target.value = "显示密码";
} else {
document.getElementById(id).type = "text";
e.target.value = "隐藏密码";
}
}
</script>
</html>
预览:
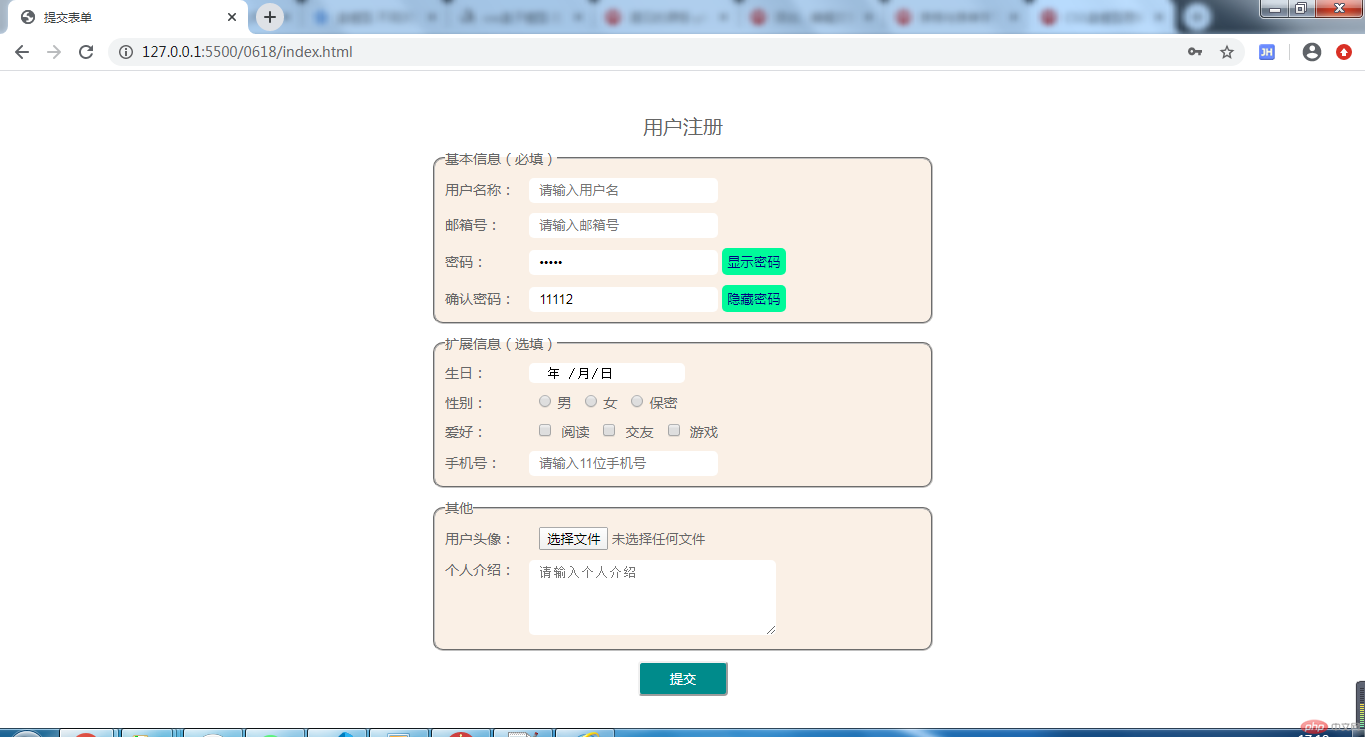