1.使用九宫格来演示选择第1个和中间那个
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>选择器:简单选择器</title>
<style>
/* 使用九宫格来演示: grid布局实现 */
.container {
width: 300px;
height: 300px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: lightskyblue;
display: flex;
justify-content: center;
align-items: center;
}
/* 类选择器:对应html标签中的class属性 */
.item {
border: 2px solid #000;
}
/* 多个类复合应用中间没有空格:.item.center */
.item.center {
background-color: lime;
}
/* 层叠样式表,相同元素,后面追加的样式会覆盖前面的样式 */
*#first {
background-color: maroon;
}
/* 元素 < class < id
id,class可以添加到任何元素上,所以可以省略*号 */
/* id 选择器的应用场景目前主要用在:表单元素、锚点 */
</style>
</head>
<body>
<div class="container">
<div class="item" id="first">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item center">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
2.使用九宫格来演示结构伪类选择器(不分组与分组):123为div一组,456789为span另一组
- 先把9个全部选上给同样的颜色
- 再在第二组里选择第3个
- 在第二组里选择前2个
- 在第二组里选择后2个
代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>结构伪类选择器:分组</title>
<style>
/* 使用九宫格来演示: grid布局实现 */
.container {
width: 300px;
height: 300px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: lightskyblue;
display: flex;
justify-content: center;
align-items: center;
}
/* 分组结构伪类:1.元素按类型进行分组;2.在分组中根据索引进行选择 */
/* .container span:last-of-type {
background-color: tomato;
} */
/* 在div分组中选择第3个 */
.container div:nth-of-type(3) {
background-color: steelblue;
}
/* 在span分组中选择第3个 */
.container span:nth-of-type(3) {
background-color: slateblue;
}
/* 拿前2个 */
.container span:nth-of-type(-n + 2) {
background-color: springgreen;
}
/* 最后2个 */
.container span:nth-last-of-type(-n + 2) {
background-color: wheat;
}
</style>
</head>
<body>
<div class="container">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<span class="item">4</span>
<span class="item">5</span>
<span class="item">6</span>
<span class="item">7</span>
<span class="item">8</span>
<span class="item">9</span>
</div>
</body>
</html>
3.用登录和列表来演示:target, :not(), ::before, ::after, :focus
- :target:锚点激活
- :focus:当获取焦点的时候执行
- :not():用于选择不满足条件元素
- ::before:在什么之前生成
- ::after:在什么之后生成
- :focus:当获取焦点的时候执行
" class="reference-link">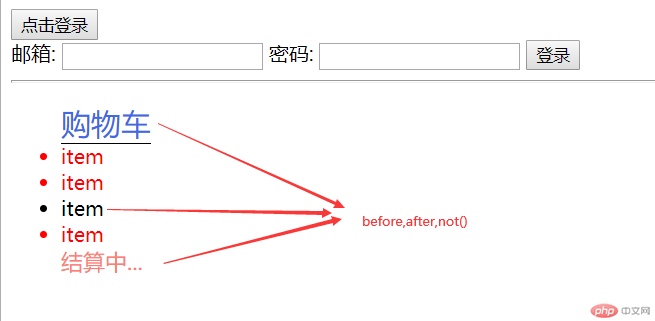
代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>伪类与伪元素</title>
<style>
#login-form {
display: none;
}
/* :target: 必须id配合,实现锚点操作 */
/* 当前#login-form的表单元素被a的锚点激活的时候执行 */
#login-form:target {
display: block;
}
/* :focus:当获取焦点的时候执行 */
input:focus {
background-color: tan;
}
/* ::selection:设置选中文本的前景色与背景色 */
input::selection {
color: thistle;
background-color: tomato;
}
/* :not():用于选择不满足条件元素 */
.list > :not(:nth-of-type(3)) {
color: red;
}
/* ::before:在什么之前生成 */
.list::before {
content: "购物车";
color: royalblue;
font-size: 1.5rem;
border-bottom: 1px solid#000;
}
/* ::after:在什么之后生成 */
.list::after {
content: "结算中...";
color: salmon;
font-size: 1.1rem;
}
</style>
</head>
<body>
<!-- <a href="#login-form">我要登录:</a> -->
<button onclick="location=`#login-form`">点击登录</button>
<form action="" method="post" id="login-form">
<label for="email">邮箱:</label>
<input type="email" name="email" id="email" />
<label for="password">密码:</label>
<input type="password" name="password" id="password" />
<button>登录</button>
</form>
<hr />
<ul class="list">
<li>item</li>
<li>item</li>
<li>item</li>
<li>item</li>
</ul>
</body>
</html>