Controller
<?php
use model\BookModel;
class BookController{
public function listAll(){
$book = new BookModel();
$data = $book->getAll();
require 'view/list.php';
}
public function info($id=1){
$id = isset($_GET['id'])?$_GET['id']:$id;
$book = new BookModel();
$data = $book->info($id);
require 'view/info.php';
}
public function add(){
require 'view/add.php';
}
public function save(){
if ($_POST['id']){
$id = intval($_POST['id']);
$data['book_name'] = trim($_POST['book_name']);
$data['category'] = trim($_POST['category']);
$data['price'] = trim($_POST['price']);
$data['num'] = trim($_POST['num']);
$data['status'] = trim($_POST['status']);
$book = new BookModel();
$data = $book->update($data,$id);
if ($data>0){
echo '<script>alert("编辑保存成功");location.assign("?a=listAll")</script>';
}
}else{
$data['book_name'] = trim($_POST['book_name']);
$data['category'] = trim($_POST['category']);
$data['price'] = trim($_POST['price']);
$data['num'] = trim($_POST['num']);
$data['status'] = trim($_POST['status']);
$book = new BookModel();
$data = $book->insert($data);
if ($data>0){
echo '<script>alert("新增保存成功");location.assign("?a=listAll")</script>';
}
}
}
}
Db类
<?php
namespace model;
class Db{
//数据库默认参数
private $dbConfig = [
'db'=>'mysql',
'host'=>'localhost',
'port'=>'3306',
'user'=>'root',
'pwd'=>'root',
'charset'=>'utf8',
'dbname'=>'demo'
];
//使用单例模式
private static $instance = null;
private $conn = null;
public $num = 0;
public $insertId = null;
//构造方法私有化
private function __construct($params){
$this->dbConfig = array_merge($this->dbConfig,$params);
//连接数据库
$this->connect();
}
//克隆方法私有化
private function __clone(){
// TODO: Implement __clone() method.
}
//自定义类实例化对象
public static function getInstance($params=[]){
if(!self::$instance instanceof self){
self::$instance = new self($params);
}
return self::$instance;
}
private function connect(){
try{
$dsn = "{$this->dbConfig['db']}:host={$this->dbConfig['host']};port={$this->dbConfig['port']};dbname={$this->dbConfig['dbname']}";
$this->conn = new \PDO($dsn,$this->dbConfig['user'],$this->dbConfig['pwd']);
$this->conn->query("SET NAMES {$this->dbConfig['charset']}");
}catch(\PDOException $e){
die('数据库连接失败'.$e->getMessage());
}
}
public function exec($sql){
//受影响的行数
$num = $this->conn->exec($sql);
if ($num>0){
if (null!==$this->conn->lastInsertId()){
$this->insertId = $this->conn->lastInsertId();
}
return $num;
}else{
$error = $this->conn->errorInfo();
print '操作失败'.$error[0].':'.$error[1].':'.$error[2];
}
}
public function fetch($sql){
return $this->conn->query($sql)->fetch(\PDO::FETCH_ASSOC);
}
public function fetchAll($sql){
return $this->conn->query($sql)->fetchAll(\PDO::FETCH_ASSOC);
}
}
Model类
<?php
namespace model;
class Model{
protected $db = null;
public $data = null;
public function __construct(){
$this->init();
}
private function init(){
$dbConfig = [
'user'=>'root',
'pwd'=>'root',
'dbname'=>'demo',
];
$this->db = Db::getInstance($dbConfig);
}
public function getAll(){
$sql = "SELECT * FROM db_book";
return $this->data = $this->db->fetchAll($sql);
}
public function info($id){
$sql = "SELECT * FROM db_book WHERE id={$id}";
return $this->data = $this->db->fetch($sql);
}
public function update($data,$id){
$sql = "UPDATE db_book SET ";
if (is_array($data)){
foreach ($data as $k=>$v){
$sql .= $k.'="'.$v.'", ';
}
}
$sql = rtrim(trim($sql),',');
$sql .= " WHERE id={$id}";
return $this->data = $this->db->exec($sql);
}
public function insert($data){
$sql = "INSERT db_book SET ";
if (is_array($data)){
foreach ($data as $k=>$v){
$sql .= $k.'="'.$v.'", ';
}
}
$sql = rtrim(trim($sql),',');
return $this->data = $this->db->exec($sql);
}
}
View-add
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>图书信息</title>
</head>
<body>
<h2 align="center">图书添加</h2>
<form action="?a=save" method="post">
<table border="1" cellpadding="5" cellspacing="0" align="center" width="50%">
<tr>
<th>图书名称</th>
<td><input type="text" name="book_name" value=""></td>
</tr>
<tr>
<th>图书类别</th>
<td>
<select name="category" id="">
<option value="">请选择</option>
<option value="1">小说</option>
<option value="2">工具书</option>
<option value="3">历史</option>
</select>
</td>
</tr>
<tr>
<th>价格</th>
<td><input type="text" name="price" value=""></td>
</tr>
<tr>
<th>存库数量</th>
<td><input type="number" name="num" value=""></td>
</tr>
<tr>
<th>状态</th>
<td>
<input type="radio" name="status" value="0" checked>启用
<input type="radio" name="status" value="1">禁用
</td>
</tr>
<tr>
<td colspan="2" align="center">
<button>保存</button>
<button type="reset">重置</button>
</td>
</tr>
</table>
</form>
</body>
</html>
View-list
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>图书信息</title>
<style>
</style>
</head>
<body>
<div>
<h2 align="center">图书信息表</h2>
<table border="1" cellpadding="3" cellspacing="0" align="center" width="70%">
<tr bgcolor="#add8e6">
<th>ID</th>
<th>图书名称</th>
<th>图书类别</th>
<th>价格</th>
<th>存库数量</th>
<th>状态</th>
<th>操作</th>
</tr>
<?php foreach($data as $book):?>
<tr align="center">
<td><?php echo $book['id'];?></td>
<td><?php echo $book['book_name'];?></td>
<td>
<?php
switch ($book['category']) {
case '1':
echo '小说';
break;
case '2':
echo '工具书';
break;
case '3':
echo '历史';
}
?>
</td>
<td><?php echo $book['price'];?></td>
<td><?php echo $book['num'];?></td>
<td><?php echo $book['status']?'禁用':'启用'; ?></td>
<td><a href="?a=info&id=<?php echo $book['id'];?>">编辑</a>|<a href="?a=del&id=<?php echo $book['id'];?>">删除</a></td>
</tr>
<?php endforeach;?>
<tr>
<td colspan="2"><button><a href="?a=add">添加图书信息</a></button></td>
<td colspan="5" align="center">合计:<?php echo count($data);?>条记录</td>
</tr>
</table>
</div>
</body>
</html>
View-update
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>图书信息</title>
</head>
<body>
<h2 align="center">图书编辑</h2>
<form action="?a=save" method="post">
<table border="1" cellpadding="5" cellspacing="0" align="center" width="50%">
<input type="hidden" name="id" value="<?php echo $data['id'];?>">
<tr>
<th>图书名称</th>
<td><input type="text" name="book_name" value="<?php echo $data['book_name']?>"></td>
</tr>
<tr>
<th>图书类别</th>
<td>
<select name="category" id="">
<option value="1" <?php if($data['category']=='1'){echo $selected="selected";}?>>小说</option>
<option value="2" <?php if($data['category']=='2'){echo $selected="selected";}?>>工具书</option>
<option value="3" <?php if($data['category']=='3'){echo $selected="selected";}?>>历史</option>
</select>
</td>
</tr>
<tr>
<th>价格</th>
<td><input type="text" name="price" value="<?php echo $data['price']?>"></td>
</tr>
<tr>
<th>存库数量</th>
<td><input type="number" name="num" value="<?php echo $data['num']?>"></td>
</tr>
<tr>
<th>状态</th>
<td>
<input type="radio" name="status" value="0" <?php if($data['status']=='0'){echo $checked="checked";}?>>启用
<input type="radio" name="status" value="1" <?php if($data['status']=='1'){echo $checked="checked";}?>>禁用
</td>
</tr>
<tr>
<td colspan="2" align="center">
<button>保存</button>
<button type="reset">重置</button>
</td>
</tr>
</table>
</form>
</body>
</html>
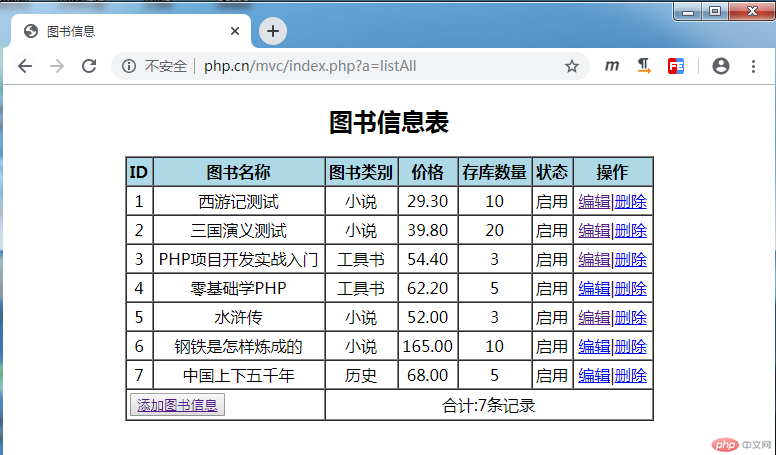