一.手机端通用布局
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>手机端通用布局</title> <link rel="stylesheet" href="1.css"> </head> <body> <header>不甜的淡月</header> <main>内容</main> <footer> <a href="">消息</a> <a href="">联系人</a> <a href="">动态</a> </footer> </body> </html>
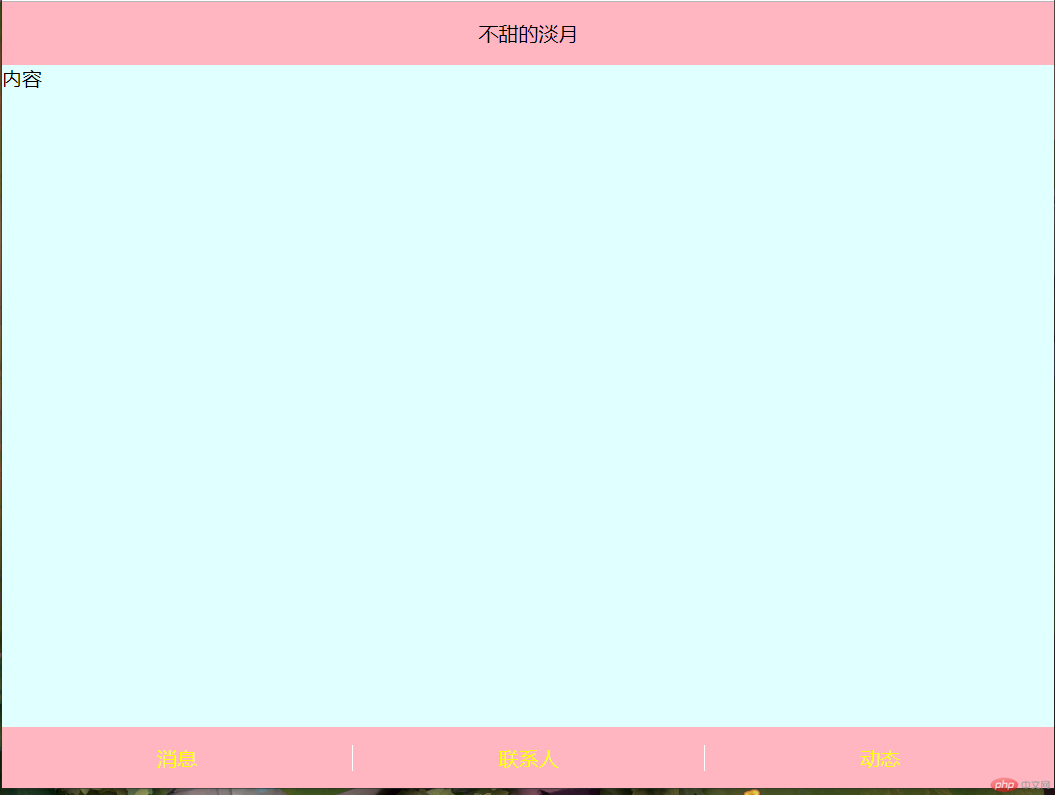
/*最简单的样式重置*/ *{ margin: 0; padding: 0; } /*链接重置*/ a{ /*去掉下划线*/ text-decoration: none; color: yellow; } body{ /*px:像素*/ /*rem:相对于html*/ /*vh:视口(可视窗口)高度百分值*/ height: 100vh; display: flex; /*主轴垂直不换行*/ flex-flow: column nowrap; } /*页眉/页脚设置样式*/ header, footer{ box-sizing: border-box; background-color: lightpink; height: 50px; display: flex; /*水平垂直居中*/ justify-content: center; align-items: center; } main{ box-sizing: border-box; background-color: lightcyan; /*将全部剩余空间分配给主体*/ flex: 1; } footer > a{ border-right: 1px solid white; flex: 1; display: flex; justify-content: center; align-items: center; } footer > a:last-of-type{ border-right: none; }
二.flex实现圣杯布局
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>flex实现圣杯布局</title> <link rel="stylesheet" href="2.css"> </head> <body> <header>不甜的淡月</header> <main> <article>主体</article> <aside>左侧</aside> <aside>右侧</aside> </main> <footer> 底部 </footer> </body> </html>
![1573123678142408.png NKF`J55MN556]{85LC`{(K8.png](https://img.php.cn/upload/image/735/444/384/1573123678142408.png)
/*最简单的样式重置*/ *{ margin: 0; padding: 0; } body{ /*px:像素*/ /*rem:相对于html*/ /*vh:视口(可视窗口)高度百分值*/ height: 100vh; /*view-height:vh*/ /*view-width:vw*/ display: flex; /*主轴垂直不换行*/ flex-flow: column nowrap; } /*页眉/页脚设置样式*/ header, footer{ box-sizing: border-box; background-color: lightgray; height: 50px; } main{ box-sizing: border-box; background-color: lightcyan; /*将全部剩余空间分配给主体*/ flex: 1; display: flex; } /*两边边栏的样式*/ main > aside{ box-sizing: border-box; width: 200px; } main > aside:first-of-type{ background-color: wheat; order: -1; } main > aside:last-of-type{ background-color: lightgreen; } main > article{ box-sizing: border-box; flex: 1; }
三.弹性布局实现登录表单
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>弹性布局实现登录表单</title> <link rel="stylesheet" href="3.css"> </head> <body> <div class="container"> <h3>管理员登录</h3> <form action=""> <div> <label for="email">账号:</label> <input type="email"id="email" name="email" placeholder="example@email.com"> </div> <div> <label for="password">密码:</label> <input type="password" id="password" name="password" placeholder="不少于六位"> </div> <div> <button>Enter</button> </div> </form> </div> </body> </html>
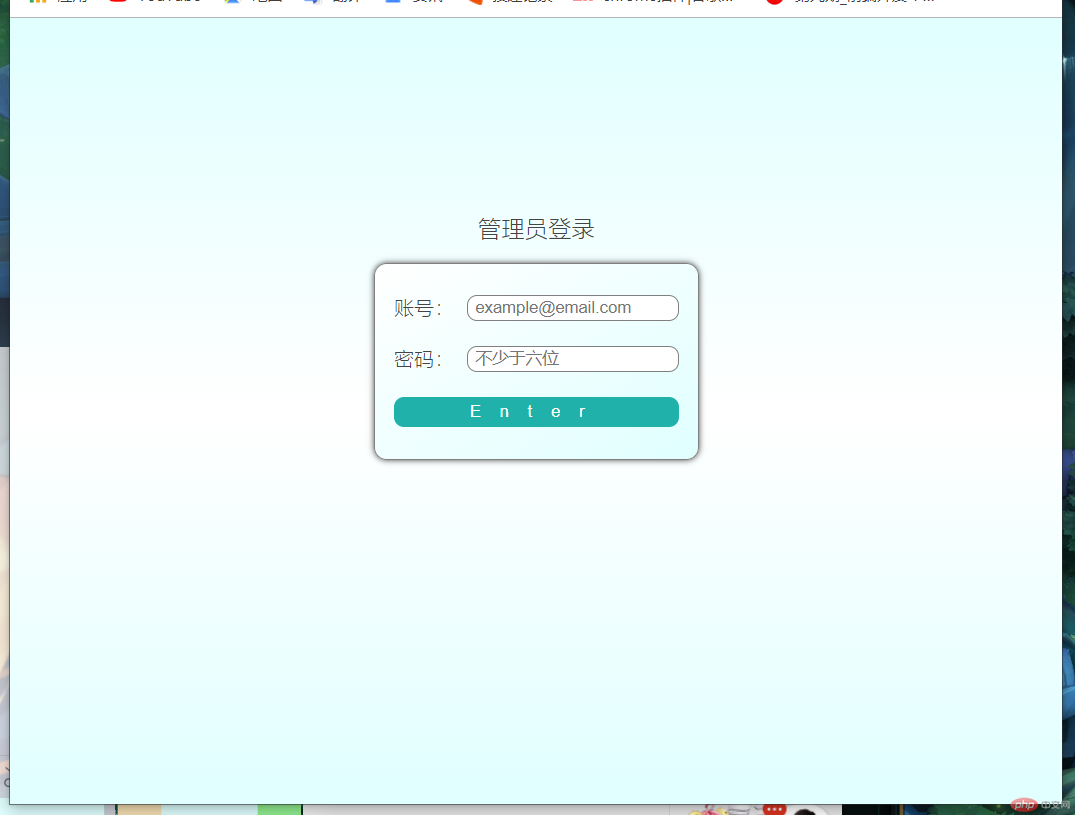
/*最简单的样式重置*/ *{ margin: 0; padding: 0; /*参考轮廓线*/ /*outline: 1px dashed red;*/ } body { /*px:像素*/ /*rem:相对于html*/ /*vh:视口(可视窗口)高度百分值*/ height: 100vh; /*view-height:vh*/ /*view-width:vw*/ display: flex; /*主轴垂直不换行*/ flex-flow: column nowrap; justify-content: center; align-items: center; color: #444; font-weight: lighter; background: linear-gradient(to top,lightcyan,white,lightcyan); } .container{ box-sizing: border-box; width: 300px; padding: 20px; /*相对定位*/ position: relative; top: -60px; } .container > h3{ text-align: center; margin-bottom: 15px; font-weight: lighter; } .container > form{ display: flex; flex-flow: column nowrap; padding: 15px; border: 1px solid gray; border-radius: 10px; background: linear-gradient(to right bottom,lightblue,white); } .container > form:hover{ background: linear-gradient(to left top,lightcyan,white); box-shadow: 0 0 5px ; } .container > form > div{ margin: 10px 0; display: flex; } .container > form > div > input{ flex: 1; margin-left: 10px; padding-left: 6px; border: 1px solid gray; border-radius: 8px; } .container > form > div > button{ flex: 1; background-color: lightseagreen; color: white; height: 24px; border: none; border-radius: 8px; letter-spacing: 15px; } .container > form > div > button:hover{ background-color: lightcoral; box-shadow: 0 0 5px #888; }
四.网站后台
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>网站后台</title> <link rel="stylesheet" href="4.css"> </head> <body> <header> <p>淡月的网站后台</p> <nav> <div><a href="https://space.bilibili.com/19157130">管理员信息</a></div> <span><a href="https://passport.bilibili.com/login">管理员登录</a></span> </nav> </header> <main> <article> <h3>欢迎进入淡月的网站后台系统</h3> <img src="aaa.jpg" alt=""> <img src="234.jpg" alt=""> </article> <aside> <div><a href="https://www.bilibili.com/">主站信息</a></div> <div><a href="https://game.bilibili.com/">游戏中心</a></div> <div><a href="https://live.bilibili.com/">直播区</a></div> <div><a href="https://www.bilibili.com/blackroom/ban?spm_id_from=333.334.b_7072696d6172795f6d656e75.111">小黑屋</a></div> </aside> </main> <footer> <p> Copyright © 2018 - 2021 php中文网 (不甜的淡月,2019.11.07) </p> </footer> </body> </html>
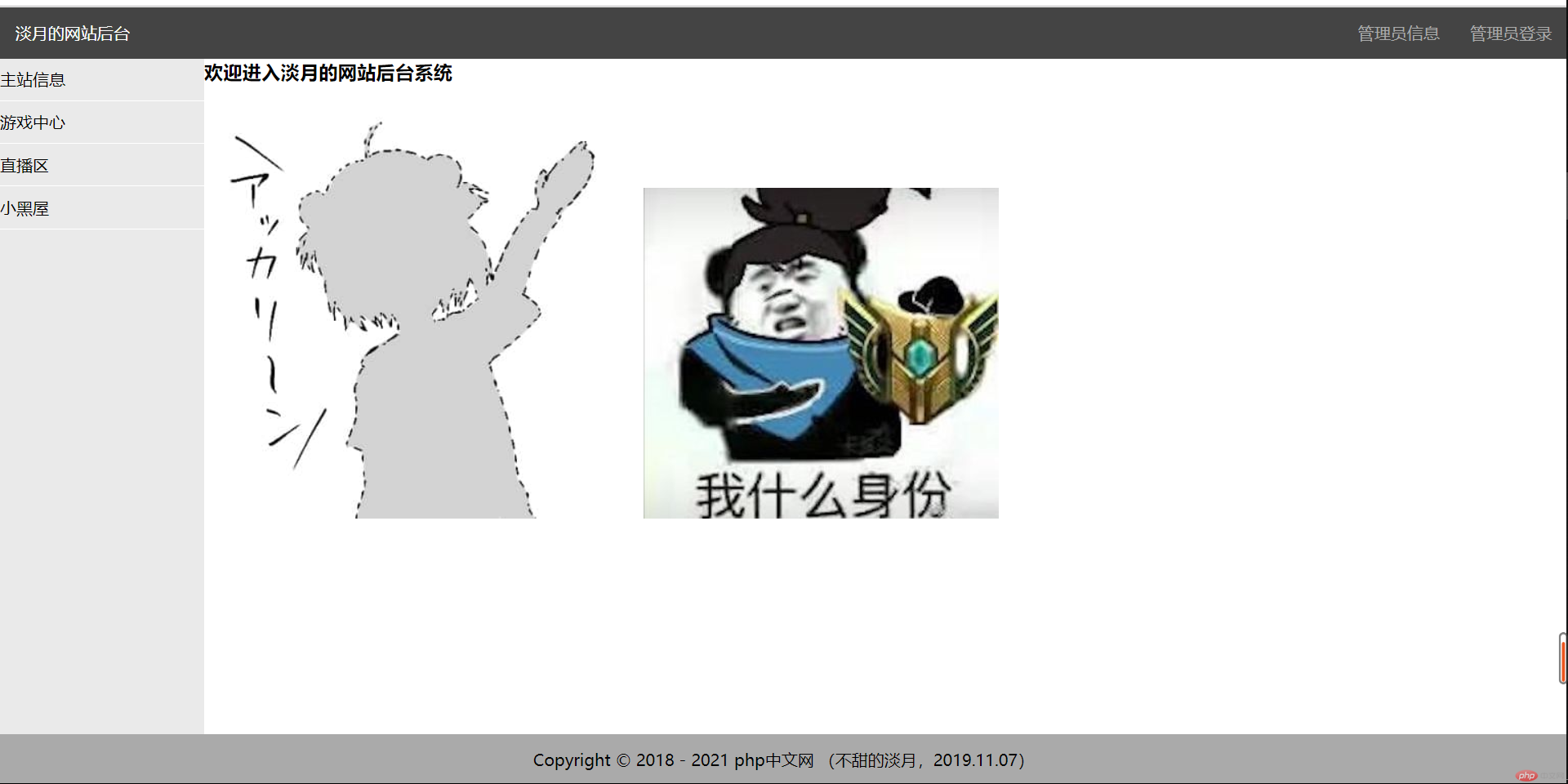
*{ margin: 0; padding: 0; /*outline: 1px dashed;*/ text-decoration: none; } body{ height: 100vh; display: flex; flex-flow: column nowrap; } header { height: 50px; background-color: #444; display: flex; justify-content: space-between; color: white; } header > p { display: flex; justify-content: center; align-items: center; padding-left: 15px; } header > nav{ display: flex; justify-content: center; align-items: center; } header > nav > div > a{ color: #aaa; } header > nav > span > a{ color: #aaa; } header > nav > *{ padding: 0 15px; } main{ display: flex; flex: 1; } main > article { flex: 1; } main > aside { width: 200px; background-color: #eaeaea; order: -1; } main > aside > div { padding: 10px 0; border-bottom: 1px solid white; } main > aside > div > a{ color: black; } footer{ height: 50px; background-color: #aaa; display: flex; justify-content: center; align-items: center; }