总长DR
- 闭包就像函数随身携带的背包,包含它们创建时的数据
- React 组件使用闭包来记住它们的状态和 props
- 当状态更新无法按预期工作时,过时的闭包可能会导致错误
- 功能更新为使用最新状态提供了可靠的解决方案
介绍
你有没有想过为什么有时你的 React 状态更新不能正常工作?或者为什么快速单击按钮多次不会按预期更新计数器?答案在于理解闭包以及 React 如何处理状态更新。在本文中,我们将使用简单的示例来阐明这些概念,让一切变得简单。
什么是闭包?
将闭包视为一个保留其诞生位置的微小记忆的函数。它就像创建函数时存在的所有变量的宝丽来快照。让我们用一个简单的计数器来看看它的实际效果:
function createPhotoAlbum() { let photoCount = 0; // This is our "snapshot" variable function addPhoto() { photoCount += 1; // This function "remembers" photoCount console.log(`Photos in album: ${photoCount}`); } function getPhotoCount() { console.log(`Current photos: ${photoCount}`); } return { addPhoto, getPhotoCount }; } const myAlbum = createPhotoAlbum(); myAlbum.addPhoto(); // "Photos in album: 1" myAlbum.addPhoto(); // "Photos in album: 2" myAlbum.getPhotoCount() // "Current photos: 2"
在此示例中,addPhoto 和 getPhotoCount 函数都会记住 photoCount 变量,即使在 createPhotoAlbum 执行完毕后也是如此。这是一个正在执行的闭包 - 函数会记住它们的出生地!
为什么闭包在 React 中很重要
在 React 中,闭包在组件如何记住其状态方面发挥着至关重要的作用。这是一个简单的计数器组件:
function Counter() { const [count, setCount] = useState(0); const increment = () => { // This function closes over 'count' setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onclick="{increment}">Add One</button> </div> ); }
increment 函数在 count 状态变量周围形成一个闭包。这就是它在单击按钮时“记住”要添加到哪个数字的方式。
问题:过时的闭包
这就是事情变得有趣的地方。让我们创建一个关闭可能导致意外行为的情况:
function BuggyCounter() { const [count, setCount] = useState(0); const incrementThreeTimes = () => { // All these updates see the same 'count' value! setCount(count + 1); // count is 0 setCount(count + 1); // count is still 0 setCount(count + 1); // count is still 0! }; return ( <div> <p>Count: {count}</p> <button onclick="{incrementThreeTimes}">Add Three</button> </div> ); }
如果单击此按钮,您可能会预期计数会增加 3。但令人惊讶的是!它只增加了 1。这是因为“过时的闭包”——我们的函数在创建时一直在查看 count 的原始值。
可以将其想象为拍摄三张显示数字 0 的白板照片,然后尝试为每张照片添加 1。每张照片中仍然有 0!
解决方案:功能更新
React 为这个问题提供了一个优雅的解决方案——功能更新:
function FixedCounter() { const [count, setCount] = useState(0); const incrementThreeTimes = () => { // Each update builds on the previous one setCount(current => current + 1); // 0 -> 1 setCount(current => current + 1); // 1 -> 2 setCount(current => current + 1); // 2 -> 3 }; return ( <div> <p>Count: {count}</p> <button onclick="{incrementThreeTimes}">Add Three</button> </div> ); }
我们不再使用闭包中的值,而是告诉 React“获取当前值并加一”。这就像有一个乐于助人的助手,在添加之前总是先查看白板上的当前数字!
现实世界示例:社交媒体点赞按钮
让我们看看这如何应用于现实场景 - 社交媒体帖子的点赞按钮:
function createPhotoAlbum() { let photoCount = 0; // This is our "snapshot" variable function addPhoto() { photoCount += 1; // This function "remembers" photoCount console.log(`Photos in album: ${photoCount}`); } function getPhotoCount() { console.log(`Current photos: ${photoCount}`); } return { addPhoto, getPhotoCount }; } const myAlbum = createPhotoAlbum(); myAlbum.addPhoto(); // "Photos in album: 1" myAlbum.addPhoto(); // "Photos in album: 2" myAlbum.getPhotoCount() // "Current photos: 2"
结论
要点
- 闭包是记住变量创建位置的函数 - 就像具有记忆功能的函数一样。
- 过时闭包当你的函数使用内存中的过时值而不是当前值时就会发生。
- React 中的功能更新 (setCount(count => count 1)) 确保您始终使用最新状态。
记住:当根据之前的值更新状态时,更喜欢功能更新。这就像有一个可靠的助手,在进行更改之前总是检查当前值,而不是凭记忆工作!
最佳实践
- 当新状态依赖于先前状态时使用功能更新
- 要特别小心异步操作和事件处理程序中的闭包
- 如有疑问,console.log 您的值以检查是否有过时的闭包
- 考虑使用 React DevTools 来调试状态更新
掌握了这些概念,您就可以像专业人士一样处理 React 中的状态更新!快乐编码! ?
以上是陷入闭包:理解 React 状态管理中的怪癖的详细内容。更多信息请关注PHP中文网其他相关文章!
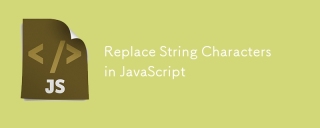
JavaScript字符串替换方法详解及常见问题解答 本文将探讨两种在JavaScript中替换字符串字符的方法:在JavaScript代码内部替换和在网页HTML内部替换。 在JavaScript代码内部替换字符串 最直接的方法是使用replace()方法: str = str.replace("find","replace"); 该方法仅替换第一个匹配项。要替换所有匹配项,需使用正则表达式并添加全局标志g: str = str.replace(/fi
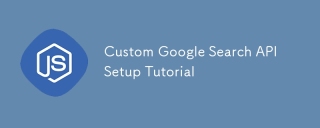
本教程向您展示了如何将自定义的Google搜索API集成到您的博客或网站中,提供了比标准WordPress主题搜索功能更精致的搜索体验。 令人惊讶的是简单!您将能够将搜索限制为Y
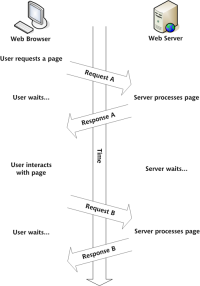
因此,在这里,您准备好了解所有称为Ajax的东西。但是,到底是什么? AJAX一词是指用于创建动态,交互式Web内容的一系列宽松的技术。 Ajax一词,最初由Jesse J创造
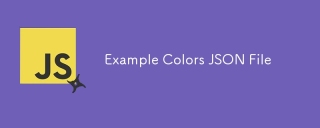
本文系列在2017年中期进行了最新信息和新示例。 在此JSON示例中,我们将研究如何使用JSON格式将简单值存储在文件中。 使用键值对符号,我们可以存储任何类型的
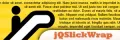
利用轻松的网页布局:8个基本插件 jQuery大大简化了网页布局。 本文重点介绍了简化该过程的八个功能强大的JQuery插件,对于手动网站创建特别有用
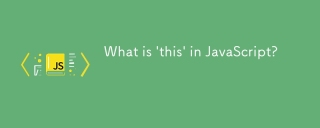
核心要点 JavaScript 中的 this 通常指代“拥有”该方法的对象,但具体取决于函数的调用方式。 没有当前对象时,this 指代全局对象。在 Web 浏览器中,它由 window 表示。 调用函数时,this 保持全局对象;但调用对象构造函数或其任何方法时,this 指代对象的实例。 可以使用 call()、apply() 和 bind() 等方法更改 this 的上下文。这些方法使用给定的 this 值和参数调用函数。 JavaScript 是一门优秀的编程语言。几年前,这句话可

jQuery是一个很棒的JavaScript框架。但是,与任何图书馆一样,有时有必要在引擎盖下发现发生了什么。也许是因为您正在追踪一个错误,或者只是对jQuery如何实现特定UI感到好奇
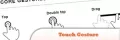
该帖子编写了有用的作弊表,参考指南,快速食谱以及用于Android,BlackBerry和iPhone应用程序开发的代码片段。 没有开发人员应该没有他们! 触摸手势参考指南(PDF) Desig的宝贵资源


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具
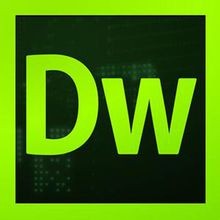
Dreamweaver CS6
视觉化网页开发工具

禅工作室 13.0.1
功能强大的PHP集成开发环境
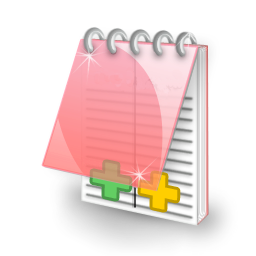
EditPlus 中文破解版
体积小,语法高亮,不支持代码提示功能

SublimeText3 英文版
推荐:为Win版本,支持代码提示!
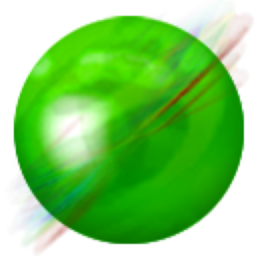
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境