这是我们关于如何使用 HTMX 和 Django 构建 Todo 应用程序系列的第二篇文章。单击此处查看第 1 部分。
在第 2 部分中,我们将创建 Todo 模型并通过单元测试实现其基本功能。
创建 Todo 模型
在 models.py 中,我们创建 Todo 模型及其基本属性。我们希望将 Todo 项目与 UserProfile 相关联,以便用户只能看到自己的项目。待办事项还将有一个标题和一个布尔属性 is_completed。我们对 Todo 模型有很多未来的想法,例如除了已完成或未开始之外还可以将任务设置为“正在进行”,以及截止日期,但那是以后的事了。现在让我们保持简单,尽快在屏幕上显示一些内容。
注意:在现实世界的应用程序中,我们可能应该考虑使用 UUID 作为 UserProfile 和 Todo 模型的主键,但我们现在会保持简单。
# core/models.py from django.contrib.auth.models import AbstractUser from django.db import models # <p>让我们运行新模型的迁移:<br> </p> <pre class="brush:php;toolbar:false">❯ uv run python manage.py makemigrations Migrations for 'core': core/migrations/0002_todo.py + Create model Todo ❯ uv run python manage.py migrate Operations to perform: Apply all migrations: admin, auth, contenttypes, core, sessions Running migrations: Applying core.0002_todo... OK
编写我们的第一个测试
让我们为我们的项目编写第一个测试。我们希望确保用户只能看到自己的待办事项,而看不到其他用户的项目。
为了帮助我们编写测试,我们将向我们的项目 model-bakery 添加新的开发依赖项,这简化了创建虚拟 Django 模型实例的过程。我们还将添加 pytest-django。
❯ uv add model-bakery pytest-django --dev Resolved 27 packages in 425ms Installed 2 packagez in 12ms + model-bakery==1.20.0 + pytest-django==4.9.0
在 pyproject.toml 中,我们需要通过在文件末尾添加一些行来配置 pytest:
# pyproject.toml # NEW [tool.pytest.ini_options] DJANGO_SETTINGS_MODULE = "todomx.settings" python_files = ["test_*.py", "*_test.py", "testing/python/*.py"]
现在让我们编写第一个测试,以确保用户只能访问自己的待办事项。
# core/tests/test_todo_model.py import pytest @pytest.mark.django_db class TestTodoModel: def test_todo_items_are_associated_to_users(self, make_todo, make_user): [user1, user2] = make_user(_quantity=2) for i in range(3): make_todo(user=user1, title=f"user1 todo {i}") make_todo(user=user2, title="user2 todo") assert {todo.title for todo in user1.todos.all()} == { "user1 todo 0", "user1 todo 1", "user1 todo 2", } assert {todo.title for todo in user2.todos.all()} == {"user2 todo"}
我们使用 pytest 中的 conftest.py 文件来存放我们计划在测试中使用的所有装置。 model_bakery 库使得使用最少的样板文件创建 UserProfile 和 Todo 的实例变得简单。
#core/tests/conftest.py import pytest from model_bakery import baker @pytest.fixture def make_user(django_user_model): def _make_user(**kwargs): return baker.make("core.UserProfile", **kwargs) return _make_user @pytest.fixture def make_todo(make_user): def _make_todo(user=None, **kwargs): return baker.make("core.Todo", user=user or make_user(), **kwargs) return _make_todo
让我们运行测试!
❯ uv run pytest Test session starts (platform: darwin, Python 3.12.8, pytest 8.3.4, pytest-sugar 1.0.0) django: version: 5.1.4, settings: todomx.settings (from ini) configfile: pyproject.toml plugins: sugar-1.0.0, django-4.9.0 collected 1 item core/tests/test_todo_model.py ✓ 100% ██████████ Results (0.25s): 1 passed
注册 Todos 的管理页面
最后,我们可以为其注册一个管理页面:
# core/admin.py from django.contrib import admin from django.contrib.auth.admin import UserAdmin from .models import Todo, UserProfile # <p>我们现在可以从管理员添加一些待办事项!</p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/173594553634650.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="Creating a To-Do app with Django and HTMX - Part Adding the Todo model with TDD"></p> <p>如果你想查看第 2 部分结束之前的整个代码,可以在 Github 的第 02 部分分支上查看。</p>
以上是使用 Django 和 HTMX 创建 To-Do 应用程序 - 使用 TDD 添加 Todo 模型部分的详细内容。更多信息请关注PHP中文网其他相关文章!
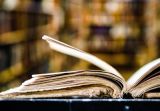
本教程演示如何使用Python处理Zipf定律这一统计概念,并展示Python在处理该定律时读取和排序大型文本文件的效率。 您可能想知道Zipf分布这个术语是什么意思。要理解这个术语,我们首先需要定义Zipf定律。别担心,我会尽量简化说明。 Zipf定律 Zipf定律简单来说就是:在一个大型自然语言语料库中,最频繁出现的词的出现频率大约是第二频繁词的两倍,是第三频繁词的三倍,是第四频繁词的四倍,以此类推。 让我们来看一个例子。如果您查看美国英语的Brown语料库,您会注意到最频繁出现的词是“th
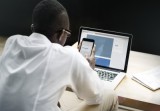
Python 提供多种从互联网下载文件的方法,可以使用 urllib 包或 requests 库通过 HTTP 进行下载。本教程将介绍如何使用这些库通过 Python 从 URL 下载文件。 requests 库 requests 是 Python 中最流行的库之一。它允许发送 HTTP/1.1 请求,无需手动将查询字符串添加到 URL 或对 POST 数据进行表单编码。 requests 库可以执行许多功能,包括: 添加表单数据 添加多部分文件 访问 Python 的响应数据 发出请求 首
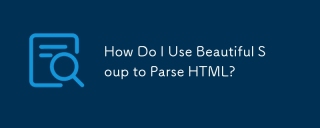
本文解释了如何使用美丽的汤库来解析html。 它详细介绍了常见方法,例如find(),find_all(),select()和get_text(),以用于数据提取,处理不同的HTML结构和错误以及替代方案(SEL)
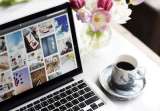
处理嘈杂的图像是一个常见的问题,尤其是手机或低分辨率摄像头照片。 本教程使用OpenCV探索Python中的图像过滤技术来解决此问题。 图像过滤:功能强大的工具 图像过滤器
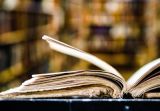
PDF 文件因其跨平台兼容性而广受欢迎,内容和布局在不同操作系统、阅读设备和软件上保持一致。然而,与 Python 处理纯文本文件不同,PDF 文件是二进制文件,结构更复杂,包含字体、颜色和图像等元素。 幸运的是,借助 Python 的外部模块,处理 PDF 文件并非难事。本文将使用 PyPDF2 模块演示如何打开 PDF 文件、打印页面和提取文本。关于 PDF 文件的创建和编辑,请参考我的另一篇教程。 准备工作 核心在于使用外部模块 PyPDF2。首先,使用 pip 安装它: pip 是 P

本教程演示了如何利用Redis缓存以提高Python应用程序的性能,特别是在Django框架内。 我们将介绍REDIS安装,Django配置和性能比较,以突出显示BENE
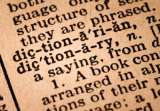
自然语言处理(NLP)是人类语言的自动或半自动处理。 NLP与语言学密切相关,并与认知科学,心理学,生理学和数学的研究有联系。在计算机科学
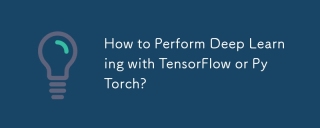
本文比较了Tensorflow和Pytorch的深度学习。 它详细介绍了所涉及的步骤:数据准备,模型构建,培训,评估和部署。 框架之间的关键差异,特别是关于计算刻度的


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

SublimeText3汉化版
中文版,非常好用
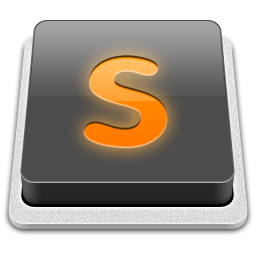
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

螳螂BT
Mantis是一个易于部署的基于Web的缺陷跟踪工具,用于帮助产品缺陷跟踪。它需要PHP、MySQL和一个Web服务器。请查看我们的演示和托管服务。
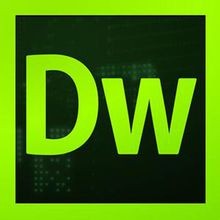
Dreamweaver CS6
视觉化网页开发工具

DVWA
Damn Vulnerable Web App (DVWA) 是一个PHP/MySQL的Web应用程序,非常容易受到攻击。它的主要目标是成为安全专业人员在合法环境中测试自己的技能和工具的辅助工具,帮助Web开发人员更好地理解保护Web应用程序的过程,并帮助教师/学生在课堂环境中教授/学习Web应用程序安全。DVWA的目标是通过简单直接的界面练习一些最常见的Web漏洞,难度各不相同。请注意,该软件中