“编程有时感觉就像一部悬疑电影 - 充满了意想不到的曲折。但是,如果您可以使代码像精心设计的场景一样流畅,该怎么办?欢迎来到回调、Promise 和异步/等待的世界!”
1. 回调
回调 是一个函数,您可以将其作为参数传递给另一个函数,然后在该函数完成后执行该函数。可以这样想:您在线订购电影票,并告诉他们在票准备好后向您发送电子邮件(回调函数)。
示例:
想象一下您正在网上订购电影《蜘蛛侠 4》的门票:
function orderTicket(movie, callback) { console.log(`Ordering ticket for ${movie}...`); // Simulate a delay with setTimeout setTimeout(() => { console.log('Ticket ordered successfully!'); callback(); // Notify when the ticket is ready }, 2000); } function notifyUser() { console.log('Your ticket is ready! Enjoy the movie!'); } orderTicket('Spider-Man 4', notifyUser);
输出:
Ordering ticket for Spider-Man 4... Ticket ordered successfully! Your ticket is ready! Enjoy the movie!
这里的notifyUser是订票后调用的回调函数。
2. 回调地狱
回调地狱 当多个回调相互嵌套时就会发生,导致代码难以阅读和维护。它看起来像金字塔或楼梯,很难跟上正在发生的事情。
示例:
现在想象一下您想要做多项事情,比如订票、买爆米花和找到座位,所有这些都按顺序进行:
function orderTicket(movie, callback) { console.log(`Ordering ticket for ${movie}...`); setTimeout(() => { console.log('Ticket ordered successfully!'); callback(); }, 2000); } function getPopcorn(callback) { console.log('Getting popcorn...'); setTimeout(() => { console.log('Popcorn ready!'); callback(); }, 1000); } function findSeat(callback) { console.log('Finding your seat...'); setTimeout(() => { console.log('Seat found!'); callback(); }, 1500); } orderTicket('Spider-Man 4', function() { getPopcorn(function() { findSeat(function() { console.log('All set! Enjoy the movie!'); }); }); });
输出:
Ordering ticket for Spider-Man 4... Ticket ordered successfully! Getting popcorn... Popcorn ready! Finding your seat... Seat found! All set! Enjoy the movie!
这是回调地狱:您可以看到代码如何变得更加嵌套且更难以阅读。
3.承诺
promise 是一个表示异步操作最终完成(或失败)的对象。它允许您编写更清晰的代码并更轻松地处理异步任务,而不会陷入回调地狱。
示例:
让我们使用 Promise
来简化上面的例子
function orderTicket(movie) { return new Promise((resolve) => { console.log(`Ordering ticket for ${movie}...`); setTimeout(() => { console.log('Ticket ordered successfully!'); resolve(); }, 2000); }); } function getPopcorn() { return new Promise((resolve) => { console.log('Getting popcorn...'); setTimeout(() => { console.log('Popcorn ready!'); resolve(); }, 1000); }); } function findSeat() { return new Promise((resolve) => { console.log('Finding your seat...'); setTimeout(() => { console.log('Seat found!'); resolve(); }, 1500); }); } orderTicket('Spider-Man 4') .then(getPopcorn) .then(findSeat) .then(() => { console.log('All set! Enjoy the movie!'); });
输出:
Ordering ticket for Spider-Man 4... Ticket ordered successfully! Getting popcorn... Popcorn ready! Finding your seat... Seat found! All set! Enjoy the movie!
Promise 通过链接 .then() 方法使代码更易于阅读,避免嵌套问题。
4. 异步/等待
Async/await 是一种现代语法,允许您编写外观和行为类似于同步代码的异步代码。它建立在 Promise 之上,使代码更加清晰、更容易理解。
示例:
让我们使用 async/await 来处理相同的场景
function orderTicket(movie) { return new Promise((resolve) => { console.log(`Ordering ticket for ${movie}...`); setTimeout(() => { console.log('Ticket ordered successfully!'); resolve(); }, 2000); }); } function getPopcorn() { return new Promise((resolve) => { console.log('Getting popcorn...'); setTimeout(() => { console.log('Popcorn ready!'); resolve(); }, 1000); }); } function findSeat() { return new Promise((resolve) => { console.log('Finding your seat...'); setTimeout(() => { console.log('Seat found!'); resolve(); }, 1500); }); } async function watchMovie() { await orderTicket('Spider-Man 4'); await getPopcorn(); await findSeat(); console.log('All set! Enjoy the movie!'); } watchMovie();
输出:
Ordering ticket for Spider-Man 4... Ticket ordered successfully! Getting popcorn... Popcorn ready! Finding your seat... Seat found! All set! Enjoy the movie!
使用 async/await,代码更加简单,类似于您在现实生活中描述过程的方式。 wait 关键字会暂停执行,直到 Promise 得到解决,从而使操作流程易于遵循。
概括 :
- 回调:另一个函数完成其工作后执行的函数。
- 回调地狱:嵌套回调导致代码难以阅读。
- Promise: 一种更简洁的方式来处理异步任务,避免回调地狱。
- 异步/等待:基于 Promise 构建的现代、易于阅读的语法,用于处理异步代码。
“通过掌握回调、Promise 和异步/等待,您可以将复杂的代码变成无缝的体验。告别回调地狱,迎接更干净、更高效的 JavaScript。快乐编码!”
以上是回调、回调地狱、Promise、异步/等待的详细内容。更多信息请关注PHP中文网其他相关文章!
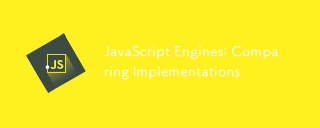
不同JavaScript引擎在解析和执行JavaScript代码时,效果会有所不同,因为每个引擎的实现原理和优化策略各有差异。1.词法分析:将源码转换为词法单元。2.语法分析:生成抽象语法树。3.优化和编译:通过JIT编译器生成机器码。4.执行:运行机器码。V8引擎通过即时编译和隐藏类优化,SpiderMonkey使用类型推断系统,导致在相同代码上的性能表现不同。
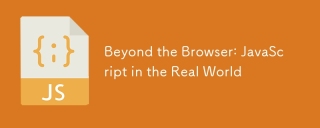
JavaScript在现实世界中的应用包括服务器端编程、移动应用开发和物联网控制:1.通过Node.js实现服务器端编程,适用于高并发请求处理。2.通过ReactNative进行移动应用开发,支持跨平台部署。3.通过Johnny-Five库用于物联网设备控制,适用于硬件交互。
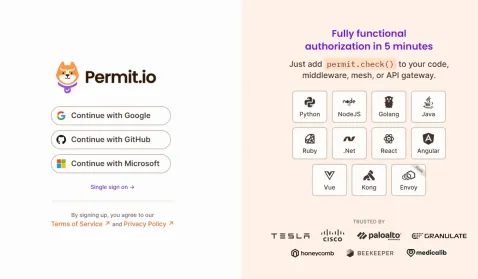
我使用您的日常技术工具构建了功能性的多租户SaaS应用程序(一个Edtech应用程序),您可以做同样的事情。 首先,什么是多租户SaaS应用程序? 多租户SaaS应用程序可让您从唱歌中为多个客户提供服务
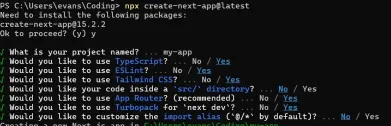
本文展示了与许可证确保的后端的前端集成,并使用Next.js构建功能性Edtech SaaS应用程序。 前端获取用户权限以控制UI的可见性并确保API要求遵守角色库
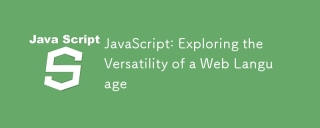
JavaScript是现代Web开发的核心语言,因其多样性和灵活性而广泛应用。1)前端开发:通过DOM操作和现代框架(如React、Vue.js、Angular)构建动态网页和单页面应用。2)服务器端开发:Node.js利用非阻塞I/O模型处理高并发和实时应用。3)移动和桌面应用开发:通过ReactNative和Electron实现跨平台开发,提高开发效率。
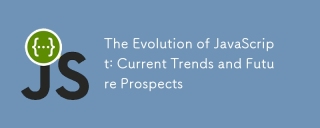
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
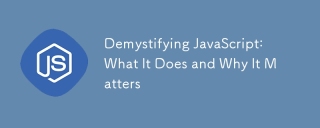
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
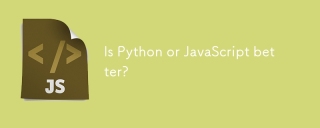
Python更适合数据科学和机器学习,JavaScript更适合前端和全栈开发。 1.Python以简洁语法和丰富库生态着称,适用于数据分析和Web开发。 2.JavaScript是前端开发核心,Node.js支持服务器端编程,适用于全栈开发。


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具
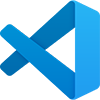
VSCode Windows 64位 下载
微软推出的免费、功能强大的一款IDE编辑器

SublimeText3 Linux新版
SublimeText3 Linux最新版
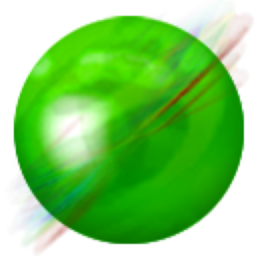
ZendStudio 13.5.1 Mac
功能强大的PHP集成开发环境

SublimeText3 英文版
推荐:为Win版本,支持代码提示!

Atom编辑器mac版下载
最流行的的开源编辑器