在机器学习领域,评估模型的性能至关重要。此评估有助于我们了解模型预测或分类数据的效果。在众多可用指标中,平均绝对误差 (MAE)、均方误差 (MSE) 和均方根误差 (RMSE) 是最常用的三个指标。但我们为什么要使用它们呢?是什么让它们如此重要?
1. 平均绝对误差(MAE)
MAE是什么?
平均绝对误差测量一组预测中误差的平均大小,而不考虑其方向。它是预测值和实际值之间的绝对差的平均值。
为什么使用 MAE?
- 可解释性: MAE 提供了对平均误差的清晰、直接的解释。如果 MAE 为 5,则模型的预测平均与实际值相差 5 个单位。
- **稳健性:**MAE 与 MSE 和 RMSE 相比对异常值不太敏感,因为它不平方误差项。
何时使用 MAE?
当您想要直接了解平均误差而不夸大大误差的影响时,MAE 是首选。当数据集存在异常值或错误成本呈线性时,它特别有用。
2. 均方误差(MSE)
什么是 MSE?
均方误差是预测值与实际值之间的平方差的平均值。
为什么使用 MSE?
- 误差放大:通过对误差进行平方,MSE 为较大误差赋予更多权重,当大误差特别不受欢迎时,MSE 成为一个很好的指标。
- 数学属性: MSE 是可微分的,并且经常在梯度下降等优化算法中用作损失函数,因为它的导数很容易计算。 何时使用 MSE?
当大错误比小错误更成问题,以及当您希望指标对大偏差进行更严厉的惩罚时,通常会使用 MSE。它在模型训练过程中也很常用,因为它计算方便。
3.均方根误差(RMSE)
什么是 RMSE?
均方根误差是 MSE 的平方根。它将指标恢复到数据的原始规模,比 MSE 更容易解释。
为什么使用 RMSE?
尺度上的可解释性:RMSE 与 MSE 不同,它与原始数据处于同一尺度,使其更具可解释性。
对大误差敏感:与 MSE 一样,RMSE 也会惩罚大误差,但由于它是在原始尺度上,因此可以提供更直观的误差大小测量。
何时使用 RMSE?
当您想要一个惩罚大错误的指标但仍需要结果与原始数据采用相同单位时,首选 RMSE。它广泛用于误差幅度分布很重要以及与数据处于相同规模至关重要的环境中。
选择正确的指标
- MAE 对异常值更加稳健,并给出与数据相同单位的平均误差,使其易于解释。
- MSE 放大较大的误差,当较大的误差代价特别昂贵时,它非常有用,并且它经常在模型训练中用作损失函数。
- RMSE 结合了 MSE 和 MAE 的优点,提供了一种错误度量,可以惩罚大错误并保持可解释性。
在实践中,MAE、MSE 和 RMSE 之间的选择取决于当前问题的具体要求。如果您的应用程序需要简单、可解释的指标,MAE 可能是最佳选择。如果您需要更严厉地惩罚较大的错误,MSE 或 RMSE 可能更合适。
图形表示
1. 建立和回归模型
以下是我们如何使用回归模型生成 MAE、MSE 和 RMSE 的图形表示:
import numpy as np import matplotlib.pyplot as plt from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_absolute_error, mean_squared_error # Generate some synthetic data for demonstration np.random.seed(42) X = 2 * np.random.rand(100, 1) y = 4 + 3 * X + np.random.randn(100, 1) # Train a simple linear regression model model = LinearRegression() model.fit(X, y) y_pred = model.predict(X) # Calculate MAE, MSE, and RMSE mae = mean_absolute_error(y, y_pred) mse = mean_squared_error(y, y_pred) rmse = np.sqrt(mse) # Plotting the regression line with errors plt.figure(figsize=(12, 8)) # Scatter plot of actual data points plt.scatter(X, y, color='blue', label='Actual Data') # Regression line plt.plot(X, y_pred, color='red', label='Regression Line') # Highlighting errors (residuals) for i in range(len(X)): plt.vlines(X[i], y[i], y_pred[i], color='gray', linestyle='dashed') # Adding annotations for MAE, MSE, RMSE plt.text(0.5, 8, f'MAE: {mae:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5)) plt.text(0.5, 7.5, f'MSE: {mse:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5)) plt.text(0.5, 7, f'RMSE: {rmse:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5)) # Titles and labels plt.title('Linear Regression with MAE, MSE, and RMSE') plt.xlabel('X') plt.ylabel('y') plt.legend() plt.show()
2. 剧情说明
- **蓝点:**这些代表实际数据点。
- 红线:这是代表模型预测值的回归线。
- 灰线:这些虚线代表每个数据点的残差或误差。这些线的长度对应于误差大小。
- MAE、MSE、RMSE: 在图中进行注释,显示这些值可帮助可视化如何评估模型的性能。
3. 解释
- MAE:给出与数据相同单位的平均误差,显示数据点与回归线的平均距离。
- MSE:对误差进行平方,更加强调较大的误差,经常在回归模型的训练过程中使用。
- RMSE:提供与原始数据相同规模的指标,使其比 MSE 更易于解释,同时仍然惩罚较大的错误。
训练机器学习模型
在训练机器学习模型时,尤其是在回归任务中,选择正确的误差度量至关重要,因为它会影响模型的学习方式及其性能评估方式。我们来分解一下MAE、MSE、RMSE在模型训练中的意义:
1.MAE(平均绝对误差)
定义:MAE 是预测值与实际值之间绝对差的平均值。
在模型训练中的意义:
- 对异常值的鲁棒性:与 MSE 和 RMSE 相比,MAE 对异常值不太敏感,因为它平等地对待所有错误,而不对它们进行平方。这意味着在训练过程中,模型的目标是最小化平均误差,而不会过分关注较大的误差。
- 线性惩罚:MAE 的线性性质意味着每个错误对模型学习过程的影响与该错误的大小成正比。
- 可解释性:MAE 与原始数据的单位相同,更容易解释。如果 MAE 为 5,则意味着模型的预测平均偏差 5 个单位。
2.MSE(均方误差)
定义:MSE 是预测值和实际值之间的平方差的平均值。
Significance in Model Training:
- Sensitivity to Outliers: MSE is sensitive to outliers because it squares the error, making larger errors much more significant in the calculation. This causes the model to prioritize reducing large errors during training.
- Punishing Large Errors: The squaring effect means that the model will penalize larger errors more severely, which can lead to a better fit for most data points but might overfit to outliers.
- Smooth Gradient: MSE is widely used in optimization algorithms like gradient descent because it provides a smooth gradient, making it easier for the model to converge during training.
- Model’s Focus on Large Errors: Since large errors have a bigger impact, the model might focus on reducing these at the cost of slightly increasing smaller errors, which can be beneficial if large errors are particularly undesirable in the application.
3. RMSE (Root Mean Squared Error)
Definition: RMSE is the square root of the average of the squared differences between the predicted and actual values.
Significance in Model Training:
- Balance between MAE and MSE: RMSE retains the sensitivity to outliers like MSE but brings the error metric back to the original scale of the data, making it more interpretable than MSE.
- Penalizes Large Errors: Similar to MSE, RMSE also penalizes larger errors more due to the squaring process, but because it takes the square root, it doesn’t exaggerate them as much as MSE does.
- Interpretable Units: Since RMSE is on the same scale as the original data, it’s easier to understand in the context of the problem. For instance, an RMSE of 5 means that on average, the model’s prediction errors are about 5 units away from the actual values.
- Optimization in Complex Models: RMSE is often used in models where the distribution of errors is important, such as in complex regression models or neural networks.
Visual Example to Show Significance in Model Training:
Let’s consider a graphical representation that shows how these metrics affect the model’s training process.
- MAE Focuses on Reducing Average Error: Imagine the model adjusting the regression line to minimize the average height of the gray dashed lines (errors) equally for all points.
- MSE Prioritizes Reducing Large Errors: The model might adjust the line more drastically to reduce the longer dashed lines (larger errors), even if it means increasing some smaller ones.
- RMSE Balances Both: The model will make adjustments that reduce large errors but will not overemphasize them to the extent of distorting the overall fit.
import numpy as np import matplotlib.pyplot as plt from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_absolute_error, mean_squared_error # Generate synthetic data with an outlier np.random.seed(42) X = 2 * np.random.rand(100, 1) y = 4 + 3 * X + np.random.randn(100, 1) y[98] = 30 # Adding an outlier # Train a simple linear regression model model = LinearRegression() model.fit(X, y) y_pred = model.predict(X) # Calculate MAE, MSE, and RMSE mae = mean_absolute_error(y, y_pred) mse = mean_squared_error(y, y_pred) rmse = np.sqrt(mse) # Plotting the regression line with errors plt.figure(figsize=(12, 8)) # Scatter plot of actual data points plt.scatter(X, y, color='blue', label='Actual Data') # Regression line plt.plot(X, y_pred, color='red', label='Regression Line') # Highlighting errors (residuals) for i in range(len(X)): plt.vlines(X[i], y[i], y_pred[i], color='gray', linestyle='dashed') # Annotating one of the residual lines plt.text(X[0] + 0.1, (y[0] + y_pred[0]) / 2, 'Error (Residual)', color='gray') # Adding annotations for MAE, MSE, RMSE plt.text(0.5, 20, f'MAE: {mae:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5)) plt.text(0.5, 18, f'MSE: {mse:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5)) plt.text(0.5, 16, f'RMSE: {rmse:.2f}', fontsize=12, bbox=dict(facecolor='white', alpha=0.5)) # Titles and labels plt.title('Linear Regression with MAE, MSE, and RMSE - Impact on Model Training') plt.xlabel('X') plt.ylabel('y') plt.legend() plt.show()
Explanation:
Outlier Impact: Notice how the model tries to adjust for the outlier in the upper region, which affects MSE and RMSE more significantly.
Model Training Implications:
- With MAE: The model may place less emphasis on the outlier, leading to a fit that is more balanced but less sensitive to extreme deviations.
- With MSE and RMSE: The model might adjust more aggressively to minimize the impact of the outlier, which can lead to a more distorted fit if outliers are rare.
Choosing the right approach for training a model depends on the specific problem you’re trying to solve, the nature of your data, and the goals of your model. Here’s a guide to help you decide which metric (MAE, MSE, RMSE) to focus on, along with considerations for training your model:
1. Nature of the Data
Presence of Outliers:
- MAE: If your data contains outliers, and you don’t want these outliers to disproportionately affect your model, MAE is a good choice. It treats all errors equally, so a few large errors won’t dominate the metric.
- MSE/RMSE: If outliers are expected and meaningful (e.g., extreme but valid cases), and you want your model to account for them strongly, MSE or RMSE might be more appropriate.
Homogeneous Data:
If your data is relatively homogeneous, without significant outliers, MSE or RMSE can help capture the overall performance, focusing more on the general fit of the model.
2. 模型的目标
可解释性:
- MAE:提供更容易的解释,因为它与目标变量的单位相同。如果原始单位的可解释性很重要,并且您想用简单的术语理解平均误差,那么 MAE 是更好的选择。
- RMSE:也可以用相同的单位解释,但重点是更多地惩罚较大的错误。
关注较大的错误:
- MSE/RMSE:如果您更关心较大的误差,因为它们在您的应用中成本特别高或风险很大(例如,预测医疗剂量、财务预测),那么 MSE 或 RMSE 应该是您的重点。这些指标对较大的错误惩罚更多,这可以指导模型优先考虑减少重大偏差。
- MAE:如果您的应用程序平等对待所有错误并且您不希望模型过度关注大偏差,那么 MAE 是更好的选择。
3. 模型类型和复杂度
简单线性模型:
- MAE:适用于简单的线性模型,其目标是最小化平均偏差,而不必过多担心异常值。
- MSE/RMSE:也可以使用,特别是如果模型需要考虑所有数据点,包括极端情况。
复杂模型(例如神经网络、集成方法):
- MSE/RMSE:这些通常用于更复杂的模型,因为它们提供更平滑的梯度,这对于梯度下降等优化技术至关重要。对较大错误的惩罚也有助于微调复杂模型。
4. 优化与收敛
梯度下降和优化:
- MSE/RMSE:在优化算法中通常是首选,因为它们提供平滑且连续的误差表面,这对于梯度下降等方法的有效收敛至关重要。
- MAE:可能不太平滑,这可能会使优化稍微更具挑战性,尤其是在大型模型中。然而,现代优化技术在许多情况下可以解决这个问题。
5. 背景考虑
应用特定要求:
- MAE:非常适合需要避免异常值影响或错误成本呈线性时的应用,例如估计交付时间或预测分数。
- MSE/RMSE:最适合特别不希望出现大错误以及应用程序要求对这些错误进行更高惩罚的情况,例如在高风险的财务预测、安全关键系统中或在优化模型时竞争环境。
结论:采取哪种方法
- 如果异常值不是主要问题:使用 MSE 或 RMSE。它们帮助模型注意较大的错误,这在许多应用中至关重要。
- 如果您想要平衡的方法:RMSE 通常是一个很好的折衷方案,因为它以与目标变量相同的单位提供度量,同时仍然比较小的误差对较大的误差进行更多的惩罚。
- 如果您需要对异常值的鲁棒性:使用 MAE。它确保异常值不会对模型产生不成比例的影响,使其适合您想要更平衡模型的情况。
- 对于原始单位的可解释性:MAE 或 RMSE 更容易解释,因为它们与目标变量的单位相同。这在您需要向非技术利益相关者解释结果的领域尤其重要。
在实践中,您可以根据这些考虑因素从一个指标开始,然后进行实验,看看您的模型在每个指标上的表现如何。在训练期间监控多个指标以全面了解模型的性能也很常见。
Medium 文章 - 了解 MAE、MSE 和 RMSE:机器学习中的关键指标
@mondalsabbha
以上是了解 MAE、MSE 和 RMSE:机器学习中的关键指标的详细内容。更多信息请关注PHP中文网其他相关文章!
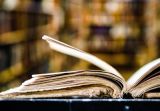
本教程演示如何使用Python处理Zipf定律这一统计概念,并展示Python在处理该定律时读取和排序大型文本文件的效率。 您可能想知道Zipf分布这个术语是什么意思。要理解这个术语,我们首先需要定义Zipf定律。别担心,我会尽量简化说明。 Zipf定律 Zipf定律简单来说就是:在一个大型自然语言语料库中,最频繁出现的词的出现频率大约是第二频繁词的两倍,是第三频繁词的三倍,是第四频繁词的四倍,以此类推。 让我们来看一个例子。如果您查看美国英语的Brown语料库,您会注意到最频繁出现的词是“th
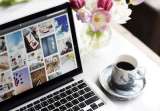
处理嘈杂的图像是一个常见的问题,尤其是手机或低分辨率摄像头照片。 本教程使用OpenCV探索Python中的图像过滤技术来解决此问题。 图像过滤:功能强大的工具 图像过滤器
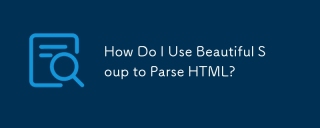
本文解释了如何使用美丽的汤库来解析html。 它详细介绍了常见方法,例如find(),find_all(),select()和get_text(),以用于数据提取,处理不同的HTML结构和错误以及替代方案(SEL)
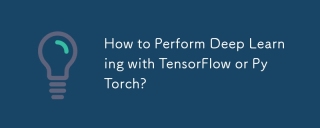
本文比较了Tensorflow和Pytorch的深度学习。 它详细介绍了所涉及的步骤:数据准备,模型构建,培训,评估和部署。 框架之间的关键差异,特别是关于计算刻度的
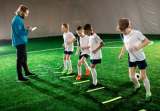
Python是数据科学和处理的最爱,为高性能计算提供了丰富的生态系统。但是,Python中的并行编程提出了独特的挑战。本教程探讨了这些挑战,重点是全球解释
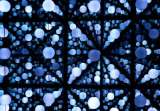
本教程演示了在Python 3中创建自定义管道数据结构,利用类和操作员超载以增强功能。 管道的灵活性在于它能够将一系列函数应用于数据集的能力,GE
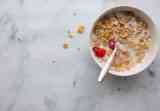
Python 对象的序列化和反序列化是任何非平凡程序的关键方面。如果您将某些内容保存到 Python 文件中,如果您读取配置文件,或者如果您响应 HTTP 请求,您都会进行对象序列化和反序列化。 从某种意义上说,序列化和反序列化是世界上最无聊的事情。谁会在乎所有这些格式和协议?您想持久化或流式传输一些 Python 对象,并在以后完整地取回它们。 这是一种在概念层面上看待世界的好方法。但是,在实际层面上,您选择的序列化方案、格式或协议可能会决定程序运行的速度、安全性、维护状态的自由度以及与其他系
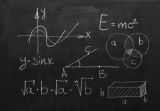
Python的statistics模块提供强大的数据统计分析功能,帮助我们快速理解数据整体特征,例如生物统计学和商业分析等领域。无需逐个查看数据点,只需查看均值或方差等统计量,即可发现原始数据中可能被忽略的趋势和特征,并更轻松、有效地比较大型数据集。 本教程将介绍如何计算平均值和衡量数据集的离散程度。除非另有说明,本模块中的所有函数都支持使用mean()函数计算平均值,而非简单的求和平均。 也可使用浮点数。 import random import statistics from fracti


热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

安全考试浏览器
Safe Exam Browser是一个安全的浏览器环境,用于安全地进行在线考试。该软件将任何计算机变成一个安全的工作站。它控制对任何实用工具的访问,并防止学生使用未经授权的资源。

SublimeText3 Linux新版
SublimeText3 Linux最新版

SublimeText3汉化版
中文版,非常好用

记事本++7.3.1
好用且免费的代码编辑器
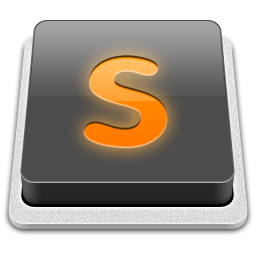
SublimeText3 Mac版
神级代码编辑软件(SublimeText3)