Java開發:如何使用多執行緒實作並發任務處理
引言:
在現代軟體開發中,高效的並發任務處理是至關重要的。在Java中,多執行緒是一種常見且強大的方式來實現並發任務處理。本文將向您介紹如何使用多執行緒來實現並發任務處理,並附帶具體的程式碼範例。
- 建立執行緒的基本方式
在Java中,可以透過繼承Thread類別或實作Runnable介面來建立執行緒。以下是兩種方式的範例程式碼:
方式一:繼承Thread類別
public class MyThread extends Thread { public void run() { // 在这里写入线程运行时需要执行的代码 } } // 创建并启动线程 MyThread myThread = new MyThread(); myThread.start();
方式二:實作Runnable介面
public class MyRunnable implements Runnable { public void run() { // 在这里写入线程运行时需要执行的代码 } } // 创建并启动线程 Thread thread = new Thread(new MyRunnable()); thread.start();
- 使用執行緒池管理執行緒
在實際應用中,直接建立執行緒可能會導致系統資源的浪費。為了更好地管理線程,可以使用線程池。 Java提供了ThreadPoolExecutor類,可以輕鬆建立線程池並管理其中的線程。下面是一個使用執行緒池的範例程式碼:
ExecutorService executorService = Executors.newFixedThreadPool(5); // 创建线程池,指定线程数量为5 for (int i = 0; i < 10; i++) { executorService.execute(new MyRunnable()); // 提交任务给线程池执行 } executorService.shutdown(); // 关闭线程池
在上述範例程式碼中,我們建立了一個固定大小為5的執行緒池。然後,我們循環提交10個任務給執行緒池執行。最後,我們呼叫shutdown()方法關閉執行緒池。
- 實作並發任務間的通訊
在執行緒之間實作通訊常常是非常重要的。 Java提供了多種方式來實作執行緒之間的通信,其中最常用的方式是使用共享變數和使用wait()、notify()方法。
使用共享變數:
public class SharedData { private int count; public synchronized void increment() { count++; } public synchronized int getCount() { return count; } } SharedData sharedData = new SharedData(); // 创建并启动多个线程 for (int i = 0; i < 10; i++) { Thread thread = new Thread(() -> { sharedData.increment(); }); thread.start(); } // 等待所有线程执行完毕 Thread.sleep(1000); System.out.println(sharedData.getCount()); // 输出结果应为10
使用wait()、notify()方法:
public class Message { private String content; private boolean isEmpty = true; public synchronized String take() { while (isEmpty) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } isEmpty = true; notifyAll(); return content; } public synchronized void put(String content) { while (!isEmpty) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } isEmpty = false; this.content = content; notifyAll(); } } Message message = new Message(); // 创建并启动多个线程 Thread producerThread = new Thread(() -> { for (int i = 0; i < 10; i++) { message.put("Message " + i); Thread.sleep(1000); } }); Thread consumerThread = new Thread(() -> { for (int i = 0; i < 10; i++) { System.out.println(message.take()); Thread.sleep(1000); } }); producerThread.start(); consumerThread.start();
- 執行緒間的同步控制
多執行緒並發執行可能會導致執行緒安全性問題,為了避免問題的發生,我們可以使用synchronized關鍵字、Lock介面等方式來對關鍵程式碼進行同步控制。以下是使用synchronized關鍵字的範例:
public class Counter { private int count = 0; public synchronized void increment() { count++; } public synchronized void decrement() { count--; } public synchronized int getCount() { return count; } } Counter counter = new Counter(); // 创建并启动多个线程 Thread incrementThread = new Thread(() -> { for (int i = 0; i < 1000; i++) { counter.increment(); } }); Thread decrementThread = new Thread(() -> { for (int i = 0; i < 1000; i++) { counter.decrement(); } }); incrementThread.start(); decrementThread.start(); incrementThread.join(); decrementThread.join(); System.out.println(counter.getCount()); // 输出结果应为0
結束語:
使用多執行緒可以有效地實現並發任務處理。在本文中,我們介紹瞭如何建立線程、使用線程池、實現線程間的通訊以及線程間的同步控制,並提供了具體的程式碼範例。希望這些內容對您在Java開發中使用多執行緒實作並發任務處理有所幫助!
以上是Java開發:如何使用多執行緒實作並發任務處理的詳細內容。更多資訊請關注PHP中文網其他相關文章!
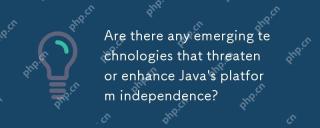
新興技術對Java的平台獨立性既有威脅也有增強。 1)雲計算和容器化技術如Docker增強了Java的平台獨立性,但需要優化以適應不同雲環境。 2)WebAssembly通過GraalVM編譯Java代碼,擴展了其平台獨立性,但需與其他語言競爭性能。
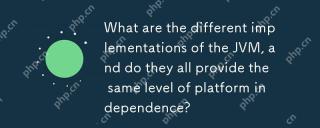
不同JVM實現都能提供平台獨立性,但表現略有不同。 1.OracleHotSpot和OpenJDKJVM在平台獨立性上表現相似,但OpenJDK可能需額外配置。 2.IBMJ9JVM在特定操作系統上表現優化。 3.GraalVM支持多語言,需額外配置。 4.AzulZingJVM需特定平台調整。
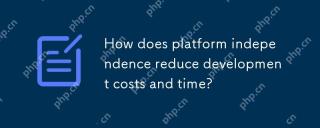
平台獨立性通過在多種操作系統上運行同一套代碼,降低開發成本和縮短開發時間。具體表現為:1.減少開發時間,只需維護一套代碼;2.降低維護成本,統一測試流程;3.快速迭代和團隊協作,簡化部署過程。
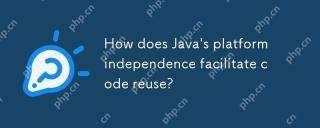
Java'splatformindependencefacilitatescodereusebyallowingbytecodetorunonanyplatformwithaJVM.1)Developerscanwritecodeonceforconsistentbehavioracrossplatforms.2)Maintenanceisreducedascodedoesn'tneedrewriting.3)Librariesandframeworkscanbesharedacrossproj
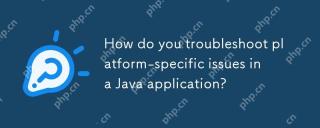
要解決Java應用程序中的平台特定問題,可以採取以下步驟:1.使用Java的System類查看系統屬性以了解運行環境。 2.利用File類或java.nio.file包處理文件路徑。 3.根據操作系統條件加載本地庫。 4.使用VisualVM或JProfiler優化跨平台性能。 5.通過Docker容器化確保測試環境與生產環境一致。 6.利用GitHubActions在多個平台上進行自動化測試。這些方法有助於有效地解決Java應用程序中的平台特定問題。
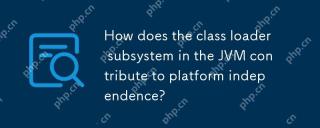
類加載器通過統一的類文件格式、動態加載、雙親委派模型和平台無關的字節碼,確保Java程序在不同平台上的一致性和兼容性,實現平台獨立性。
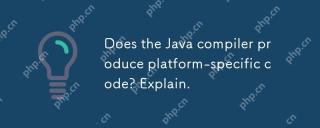
Java編譯器生成的代碼是平台無關的,但最終執行的代碼是平台特定的。 1.Java源代碼編譯成平台無關的字節碼。 2.JVM將字節碼轉換為特定平台的機器碼,確保跨平台運行但性能可能不同。
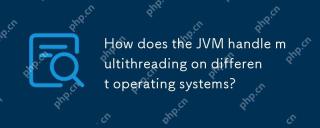
多線程在現代編程中重要,因為它能提高程序的響應性和資源利用率,並處理複雜的並發任務。 JVM通過線程映射、調度機制和同步鎖機制,在不同操作系統上確保多線程的一致性和高效性。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),
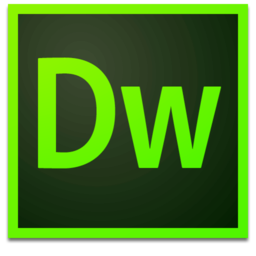
Dreamweaver Mac版
視覺化網頁開發工具

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。