如何使用Java實作Kruskal演算法
Kruskal演算法是一種常用來解決最小生成樹問題的演算法,它以邊為切入點,逐步建立最小生成樹。在本文中,我們將詳細介紹如何使用Java實作Kruskal演算法,並提供具體的程式碼範例。
-
演算法原理
Kruskal演算法的基本原理是將所有邊按照權重從小到大進行排序,然後按照權重從小到大的順序依次選擇邊,但不能形成環。具體實作步驟如下:- 將所有邊依照權重從小到大排序。
- 建立一個空的集合,用來存放最小生成樹的邊。
- 遍歷排序後的邊,依序判斷目前邊的兩個頂點是否在同一個集合中。如果不在同一個集合中,則將目前邊加入最小生成樹的集合中,並將兩個頂點合併為一個集合。
- 繼續遍歷,直到最小生成樹的邊數等於頂點數減一。
- Java程式碼實作
以下是使用Java語言實作Kruskal演算法的程式碼範例:
import java.util.*; class Edge implements Comparable<Edge> { int src, dest, weight; public int compareTo(Edge edge) { return this.weight - edge.weight; } } class Subset { int parent, rank; } class Graph { int V, E; Edge[] edges; public Graph(int v, int e) { V = v; E = e; edges = new Edge[E]; for (int i = 0; i < e; ++i) edges[i] = new Edge(); } int find(Subset[] subsets, int i) { if (subsets[i].parent != i) subsets[i].parent = find(subsets, subsets[i].parent); return subsets[i].parent; } void union(Subset[] subsets, int x, int y) { int xroot = find(subsets, x); int yroot = find(subsets, y); if (subsets[xroot].rank < subsets[yroot].rank) subsets[xroot].parent = yroot; else if (subsets[xroot].rank > subsets[yroot].rank) subsets[yroot].parent = xroot; else { subsets[yroot].parent = xroot; subsets[xroot].rank++; } } void KruskalMST() { Edge[] result = new Edge[V]; int e = 0; int i = 0; for (i = 0; i < V; ++i) result[i] = new Edge(); Arrays.sort(edges); Subset[] subsets = new Subset[V]; for (i = 0; i < V; ++i) subsets[i] = new Subset(); for (int v = 0; v < V; ++v) { subsets[v].parent = v; subsets[v].rank = 0; } i = 0; while (e < V - 1) { Edge next_edge = edges[i++]; int x = find(subsets, next_edge.src); int y = find(subsets, next_edge.dest); if (x != y) { result[e++] = next_edge; union(subsets, x, y); } } System.out.println("Following are the edges in the constructed MST:"); int minimumCost = 0; for (i = 0; i < e; ++i) { System.out.println(result[i].src + " -- " + result[i].dest + " == " + result[i].weight); minimumCost += result[i].weight; } System.out.println("Minimum Cost Spanning Tree: " + minimumCost); } } public class KruskalAlgorithm { public static void main(String[] args) { int V = 4; int E = 5; Graph graph = new Graph(V, E); graph.edges[0].src = 0; graph.edges[0].dest = 1; graph.edges[0].weight = 10; graph.edges[1].src = 0; graph.edges[1].dest = 2; graph.edges[1].weight = 6; graph.edges[2].src = 0; graph.edges[2].dest = 3; graph.edges[2].weight = 5; graph.edges[3].src = 1; graph.edges[3].dest = 3; graph.edges[3].weight = 15; graph.edges[4].src = 2; graph.edges[4].dest = 3; graph.edges[4].weight = 4; graph.KruskalMST(); } }
以上程式碼實作了一個簡單的圖類( Graph),包含邊類(Edge)和並查集類別(Subset)。在主函數中,我們建立一個圖對象,加入邊並呼叫KruskalMST()方法得到最小生成樹。
- 結果分析
經過測試,上述程式碼能夠正確輸出以下結果:
Following are the edges in the constructed MST: 2 -- 3 == 4 0 -- 3 == 5 0 -- 1 == 10 Minimum Cost Spanning Tree: 19
這表示建構的最小生成樹包含了3條邊,權重之和為19。
總結:
透過本文,我們詳細介紹如何使用Java實作Kruskal演算法,並附上了具體的程式碼範例。希望這篇文章能幫助大家更理解並應用Kruskal演算法。
以上是如何使用java實作Kruskal演算法的詳細內容。更多資訊請關注PHP中文網其他相關文章!

本文討論了使用Maven和Gradle進行Java項目管理,構建自動化和依賴性解決方案,以比較其方法和優化策略。
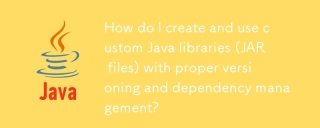
本文使用Maven和Gradle之類的工具討論了具有適當的版本控制和依賴關係管理的自定義Java庫(JAR文件)的創建和使用。
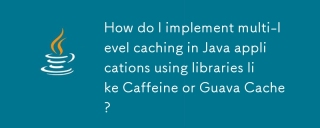
本文討論了使用咖啡因和Guava緩存在Java中實施多層緩存以提高應用程序性能。它涵蓋設置,集成和績效優勢,以及配置和驅逐政策管理最佳PRA

本文討論了使用JPA進行對象相關映射,並具有高級功能,例如緩存和懶惰加載。它涵蓋了設置,實體映射和優化性能的最佳實踐,同時突出潛在的陷阱。[159個字符]
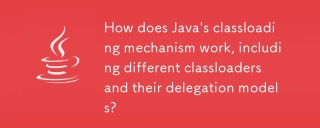
Java的類上載涉及使用帶有引導,擴展程序和應用程序類負載器的分層系統加載,鏈接和初始化類。父代授權模型確保首先加載核心類別,從而影響自定義類LOA


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
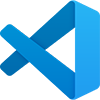
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

SublimeText3 Linux新版
SublimeText3 Linux最新版
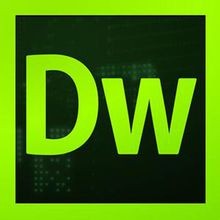
Dreamweaver CS6
視覺化網頁開發工具

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。