這篇文章帶大家深入了解一下Angular中常用的錯誤處理方式,希望對大家有幫助!
錯誤處理是編寫程式碼經常遇見的並且必須處理的需求,很多時候處理異常的邏輯是為了避免程式的崩潰,本文將簡單介紹Angular
處理異常的方式。 【相關教學推薦:《angular教學》】
什麼是Angular
Angualr
是一款來自Google的開源的web 前端框架,誕生於2009 年,由Misko Hevery 等人創建,後來由Google 所收購。是一款優秀的前端 JS 框架,已經被用於 Google 的多款產品當中。
AngularJS 是基於聲明式程式設計模式是使用者可以基於業務邏輯進行開發. 該框架基於HTML的內容填充並做了雙向資料綁定從而完成了自動資料同步機制. 最後, AngularJS強化的DOM操作增強了可測試性.
try/catch
最熟悉的方式,就是在程式碼中加入try/ catch
區塊,在try
中發生錯誤,就會被捕獲並且讓腳本繼續執行。然而,隨著應用程式規模的擴大,這種方式將變得無法管理。
ErrorHandler
Angular
提供了一個預設的ErrorHandler
,可以將錯誤訊息列印到控制台,因此可以攔截這個預設行為來添加自訂的處理邏輯,下面嘗試編寫錯誤處理類別:
import { ErrorHandler, Injectable } from "@angular/core"; import { HttpErrorResponse } from "@angular/common/http"; @Injectable() export class ErrorsHandler implements ErrorHandler { handleError(error: Error | HttpErrorResponse) { if (!navigator.onLine) { console.error("Browser Offline!"); } else { if (error instanceof HttpErrorResponse) { if (!navigator.onLine) { console.error("Browser Offline!"); } else { // Handle Http Error (4xx, 5xx, ect.) console.error("Http Error!"); } } else { // Handle Client Error (Angular Error, ReferenceError...) console.error("Client Error!"); } console.error(error); } } }
#通常在
app
#下建立一個共享目錄shared
,並將此檔案放在providers
資料夾中
現在,需要更改應用程式的預設行為,以使用我們自訂的類別而不是ErrorHandler
。修改app.module.ts
文件,從@angular/core
導入ErrorHandler
,並將providers
添加到@NgModule
模組,程式碼如下:
import { NgModule, ErrorHandler } from "@angular/core"; import { BrowserModule } from "@angular/platform-browser"; import { FormsModule } from "@angular/forms"; // Providers import { ErrorsHandler } from "./shared/providers/error-handler"; import { AppComponent } from "./app.component"; @NgModule({ imports: [BrowserModule, FormsModule], declarations: [AppComponent], providers: [{ provide: ErrorHandler, useClass: ErrorsHandler }], bootstrap: [AppComponent] }) export class AppModule {}
HttpInterceptor
#HttpInterceptor
提供了一種攔截HTTP請求/回應的方法,就可以在傳遞它們之前處理。例如,可以在拋出錯誤之前重試幾次HTTP請求。這樣,就可以優雅地處理超時,而不必拋出錯誤。
還可以在拋出錯誤之前檢查錯誤的狀態,使用攔截器,可以檢查401狀態錯誤碼,將使用者重新導向到登入頁面。
import { Injectable } from "@angular/core"; import { HttpEvent, HttpRequest, HttpHandler, HttpInterceptor, HttpErrorResponse } from "@angular/common/http"; import { Observable, throwError } from "rxjs"; import { retry, catchError } from "rxjs/operators"; @Injectable() export class HttpsInterceptor implements HttpInterceptor { intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> { return next.handle(request).pipe( retry(1), catchError((error: HttpErrorResponse) => { if (error.status === 401) { // 跳转到登录页面 } else { return throwError(error); } }) ); } }
同樣需要添加到app.module.ts
中
import { NgModule, ErrorHandler } from "@angular/core"; import { HTTP_INTERCEPTORS } from "@angular/common/http"; import { BrowserModule } from "@angular/platform-browser"; import { FormsModule } from "@angular/forms"; // Providers import { ErrorsHandler } from "./shared/providers/error-handler"; import { HttpsInterceptor } from "./shared/providers/http-interceptor"; import { AppComponent } from "./app.component"; @NgModule({ imports: [BrowserModule, FormsModule], declarations: [AppComponent], providers: [ { provide: ErrorHandler, useClass: ErrorsHandler }, { provide: HTTP_INTERCEPTORS, useClass: HttpsInterceptor, multi: true } ], bootstrap: [AppComponent] }) export class AppModule {}
多提供者用於建立可擴展服務,其中系統帶有一些預設提供者,也可以註冊其他提供者。預設提供者和其他提供者的組合將用於驅動系統的行為。
Notifications
在控制台列印錯誤日誌對於開發者來說非常友好,但是對於使用者來說則需要一種更加友好的方式來告訴這些錯誤何時從GUI發生。根據錯誤類型,推薦兩個元件:Snackbar
和Dialog
#Snackbar
:推薦用於簡單的提示,例如表單缺少必填欄位或通知使用者可預見的錯誤(無效電子郵件、使用者名稱太長等)。Dialog
:當存在未知的伺服器端或客戶端錯誤時,建議使用這種方式;透過這種方式,可以顯示更多的描述,甚至call-to-action
,例如允許用戶輸入他們的電子郵件來追蹤錯誤。
在shared
資料夾中新增一個服務來處理所有通知,新建services
資料夾,建立檔案:notification. service.ts
,程式碼如下:
import { Injectable } from "@angular/core"; import { MatSnackBar } from "@angular/material/snack-bar"; @Injectable({ providedIn: "root" }) export class NotificationService { constructor(public snackBar: MatSnackBar) {} showError(message: string) { this.snackBar.open(message, "Close", { panelClass: ["error"] }); } }
更新error-handler.ts
,新增NotificationService
:
import { ErrorHandler, Injectable, Injector } from "@angular/core"; import { HttpErrorResponse } from "@angular/common/http"; // Services import { NotificationService } from "../services/notification.service"; @Injectable() export class ErrorsHandler implements ErrorHandler { //Error handling需要先加载,使用Injector手动注入服务 constructor(private injector: Injector) {} handleError(error: Error | HttpErrorResponse) { const notifier = this.injector.get(NotificationService); if (!navigator.onLine) { //console.error("Browser Offline!"); notifier.showError("Browser Offline!"); } else { if (error instanceof HttpErrorResponse) { if (!navigator.onLine) { //console.error("Browser Offline!"); notifier.showError(error.message); } else { // Handle Http Error (4xx, 5xx, ect.) // console.error("Http Error!"); notifier.showError("Http Error: " + error.message); } } else { // Handle Client Error (Angular Error, ReferenceError...) // console.error("Client Error!"); notifier.showError(error.message); } console.error(error); } } }
如果在一個元件中拋出一個錯誤,可以看到一個很好的snackbar
訊息:
#日誌和錯誤追蹤
當然不能期望使用者向報告每個bug
,一旦部署到生產環境中,就不能看到任何控制台日誌。因此就需要能夠記錄錯誤的後端服務與自訂邏輯寫入資料庫或使用現有的解決方案,如Rollbar
、Sentry
、Bugsnag
。
接下來建立一個簡單的錯誤追蹤服務,建立logging.service.ts
:
import { Injectable } from "@angular/core"; import { HttpErrorResponse } from "@angular/common/http"; @Injectable({ providedIn: "root" }) export class LoggingService { constructor() {} logError(error: Error | HttpErrorResponse) { // This will be replaced with logging to either Rollbar, Sentry, Bugsnag, ect. if (error instanceof HttpErrorResponse) { console.error(error); } else { console.error(error); } } }
將服務加入error-handler.ts
中:
import { ErrorHandler, Injectable, Injector } from "@angular/core"; import { HttpErrorResponse } from "@angular/common/http"; // Services import { NotificationService } from "../services/notification.service"; import { LoggingService } from "../services/logging.service"; @Injectable() export class ErrorsHandler implements ErrorHandler { //Error handling需要先加载,使用Injector手动注入服务 constructor(private injector: Injector) {} handleError(error: Error | HttpErrorResponse) { const notifier = this.injector.get(NotificationService); const logger = this.injector.get(LoggingService); if (!navigator.onLine) { //console.error("Browser Offline!"); notifier.showError("Browser Offline!"); } else { if (error instanceof HttpErrorResponse) { if (!navigator.onLine) { //console.error("Browser Offline!"); notifier.showError(error.message); } else { // Handle Http Error (4xx, 5xx, ect.) // console.error("Http Error!"); notifier.showError("Http Error: " + error.message); } } else { // Handle Client Error (Angular Error, ReferenceError...) // console.error("Client Error!"); notifier.showError(error.message); } // console.error(error); logger.logError(error); } } }
至此,整個錯誤處理的機制已經介紹完了,基本上跟其他框架或語言開發的專案處理方式類似。
更多程式相關知識,請造訪:程式設計影片! !
以上是聊聊Angular中常用的錯誤處理方式的詳細內容。更多資訊請關注PHP中文網其他相關文章!
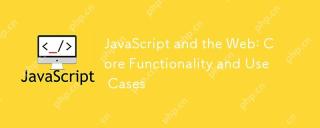
JavaScript在Web開發中的主要用途包括客戶端交互、表單驗證和異步通信。 1)通過DOM操作實現動態內容更新和用戶交互;2)在用戶提交數據前進行客戶端驗證,提高用戶體驗;3)通過AJAX技術實現與服務器的無刷新通信。
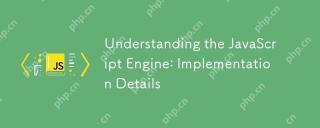
理解JavaScript引擎內部工作原理對開發者重要,因為它能幫助編寫更高效的代碼並理解性能瓶頸和優化策略。 1)引擎的工作流程包括解析、編譯和執行三個階段;2)執行過程中,引擎會進行動態優化,如內聯緩存和隱藏類;3)最佳實踐包括避免全局變量、優化循環、使用const和let,以及避免過度使用閉包。

Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
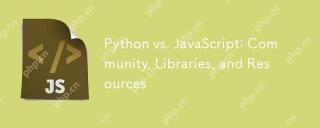
Python和JavaScript在社區、庫和資源方面的對比各有優劣。 1)Python社區友好,適合初學者,但前端開發資源不如JavaScript豐富。 2)Python在數據科學和機器學習庫方面強大,JavaScript則在前端開發庫和框架上更勝一籌。 3)兩者的學習資源都豐富,但Python適合從官方文檔開始,JavaScript則以MDNWebDocs為佳。選擇應基於項目需求和個人興趣。
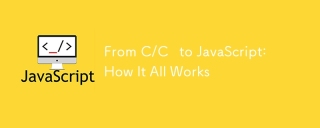
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
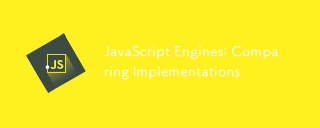
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
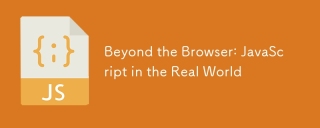
JavaScript在現實世界中的應用包括服務器端編程、移動應用開發和物聯網控制:1.通過Node.js實現服務器端編程,適用於高並發請求處理。 2.通過ReactNative進行移動應用開發,支持跨平台部署。 3.通過Johnny-Five庫用於物聯網設備控制,適用於硬件交互。
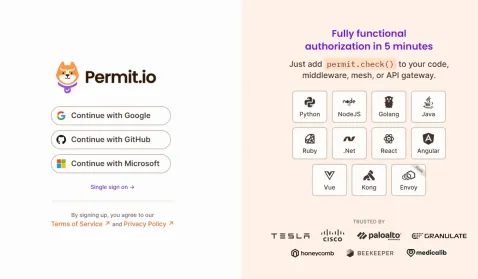
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
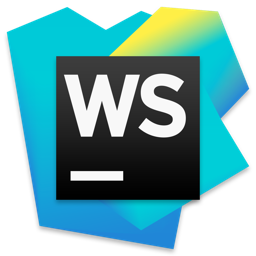
WebStorm Mac版
好用的JavaScript開發工具
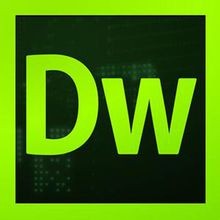
Dreamweaver CS6
視覺化網頁開發工具

Atom編輯器mac版下載
最受歡迎的的開源編輯器

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。