Warning information about message entitiesWarn: 199 Miscellaneous warning | | 2.Response header of HTTP response:Also gives the picture in the previous section:
PS: The first line is: protocol version number, status code 302 means it is not available here, but it is available in another place, and it is redirected through the Location page 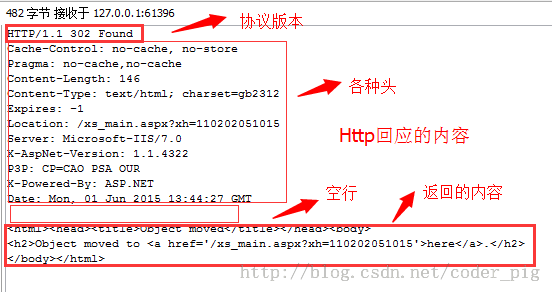
HTTP Responses Header Response header information comparison table: Header | Explanation | Example |
---|
Accept-Ranges | Indicates whether the server supports specified range requests and which type of segmented requests | Accept -Ranges: bytes | Age | Estimated time (in seconds, non-negative) from origin server to proxy cache formation | Age: 12 | Allow | A valid request behavior for a certain network resource. If it is not allowed, 405 | # will be returned. ##Allow: GET, HEAD | ##Cache-ControlTell all caching mechanisms whether they can be cached and what type | Cache-Control: no-cache | | Content-EncodingThe returned content compression encoding type supported by the web server | Content-Encoding: gzip | | ##Content-LanguageThe language of the response body | Content-Language: en,zh | | Content-LengthThe length of the response body | Content-Length: 348 | | Content-LocationRequest an alternative address for the resource | Content-Location: /index.htm | | Content-MD5Returns the MD5 check value of the resource | Content-MD5: Q2hlY2sgSW50ZWdyaXR5IQ== | | Content-RangeThe byte position of this part in the entire return body | Content-Range: bytes 21010-47021/47022 | | Content-TypeReturn the MIME type of the content | Content-Type: text/html; charset=utf-8 | | DateTime when the original server message was sent | Date: Tue, 15 Nov 2010 08:12:31 GMT | | ETagThe current value of the entity tag of the request variable | ETag: "737060cd8c284d8af7ad3082f209582d" | | Expires Response expiration date and time | Expires: Thu, 01 Dec 2010 16:00:00 GMT | | Last-ModifiedThe last modified time of the requested resource | Last-Modified: Tue, 15 Nov 2010 12:45:26 GMT | | LocationUsed to redirect the recipient to the location of the non-requested URL to complete the request or identify a new resource | Location: http://blog.csdn.net/coder_pig | Pragma | Includes implementation-specific directives that can be applied to any receiver on the response chain | Pragma: no-cache | Proxy-Authenticate | It indicates the authentication scheme and parameters that can be applied to the proxy on that URL | Proxy-Authenticate: Basic |
3. The role of the code verification response header: Okay, after reading so many concepts, how can I do it without taking action? Right, then let's write some simple code to verify some
Let’s take a look at the functions of commonly used response headers in order to deepen our understanding. Here, the server will use the simplest Servlet to implement it. If not
Java Web friends only need to copy the code, configure web.xml, and add the Servlet class name, such as:
FirstServletcom.jay.server.FirstServletFirstServlet/FirstServlet to the corresponding class name!
1) Implement page redirection through LocationImplementation code: package com.jay.http.test;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ServletOne extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//告诉浏览器响应码,以及重定向页面
resp.setStatus(302);
resp.setHeader("Location", "http://www.baidu.com");
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
this.doGet(req, resp);
}
} Running result: When we visit: http://localhost:8080/HttpTest/ServletOne, we will find that the page jumps to Baidu,
Then we use FireFox’s developer tools: you can see the content of the HTTP we sent: 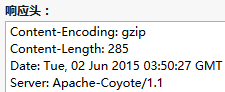
2) Tell the browser the compression format of the data through Content-Encoding Implementation code: package com.jay.http.test;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.zip.GZIPOutputStream;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ServletTwo extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
String data = "Fresh air and sunshine can have an amazing effect on our feelings. "
+ "Sometimes when we are feeling down, all that we need to do is simply to go "
+ "outside and breathe. Movement and exercise is also a fantastic way to feel better. "
+ "Positive emotions can be generated by motion. So if we start to feel down,"
+ " take some deep breathes, go outside, feel the fresh air, "
+ "let the sun hit our face, go for a hike, a walk, a bike ride, "
+ "a swim, a run, whatever. We will feel better if we do this.";
System.out.println("原始数据长度:" + data.getBytes().length);
// 对数据进行压缩:
ByteArrayOutputStream bout = new ByteArrayOutputStream();
GZIPOutputStream gout = new GZIPOutputStream(bout);
gout.write(data.getBytes());
gout.close();
// 得到压缩后的数据
byte gdata[] = bout.toByteArray();
resp.setHeader("Content-Encoding", "gzip");
resp.setHeader("Content-Length", gdata.length + "");
resp.getOutputStream().write(gdata);
}
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doGet(req, resp);
};
} Running result:  ##Console output: 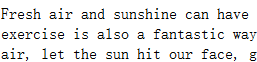 Browser output: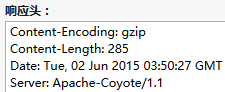 This gzip compressed string is not efficient for simple string compression. For example, Xiaozhu originally wrote a string of poems about a quiet night.
Later, I found that the compressed size was even larger than the original one =-=... 3) Set the returned data type through content-type What the server returns may sometimes be a text/html, sometimes it may be an image/jpeg, or a video/avi
The browser can display the data in different ways according to the corresponding data type! Okay, here we get a implementation code for reading PDF: package com.jay.http.test;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ServletThree extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
resp.setHeader("content-type", "application/pdf");
InputStream in = this.getServletContext().getResourceAsStream("/file/android编码规范.pdf");
byte buffer[] = new byte[1024];
int len = 0;
OutputStream out = resp.getOutputStream();
while((len = in.read(buffer)) > 0)
{
out.write(buffer,0,len);
}
}
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws
ServletException ,IOException
{
doGet(req, resp);
};
} Running result: Enter on the browser: http://localhost:8080/HttpTest/ServletThreeOkay, you can indeed read the PDF. By the way, I have lost this PDF in the file directory of the webroot, otherwise it will Report a null pointer~:Of course, you can also try to play a piece of music or video, just modify the content-type parameterThe following is an HTTP Content- Type comparison table: HTTP Content-type comparison table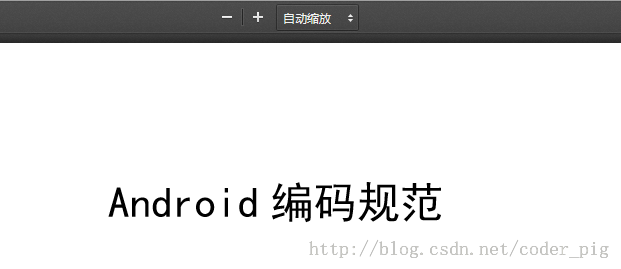
4) Use the refresh response header to let the browser jump to another page after a few seconds Well, generally we may have such a need, such as every few seconds Refresh the page every second, or a few seconds after loading a page
Jump to another page, then refresh can meet your needs~ Implementation code: package com.jay.http.test;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ServletFour extends HttpServlet {
public int second = 0;
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//1.浏览器每隔2秒定时刷新页面
// resp.setHeader("refresh", "2");
// resp.getWriter().write(++second + "");
// System.out.println("doGet方法被调用~");
//2.进入页面5s后,然页跳到百度~
resp.setHeader("refresh", "5;url='http://www.baidu.com'");
resp.getWriter().write("HE HE DA~");
}
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException ,IOException
{
doGet(req, resp);
};
} Running result: - 1 If the page is refreshed every 2 seconds, we can see that the displayed number is increasing, and the doGet method is also called all the time.
The description page is really refreshed! If
- 2, 5 seconds after entering the page, you will jump to Baidu~
##5) Let the browser download the file through the content-dispostion response header This is very simple. We only need to remove the line that sets Content-type in ③, and then add: resp.setHeader("content-disposition", "attachment;filename=Android .pdf"); Implementation code: package com.jay.http.test;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class ServletFive extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
resp.setHeader("content-disposition", "attachment;filename=Android.pdf");
InputStream in = this.getServletContext().getResourceAsStream("/file/android编码规范.pdf");
byte buffer[] = new byte[1024];
int len = 0;
OutputStream out = resp.getOutputStream();
while((len = in.read(buffer)) > 0)
{
out.write(buffer,0,len);
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doGet(req, resp);
}
} Running result: 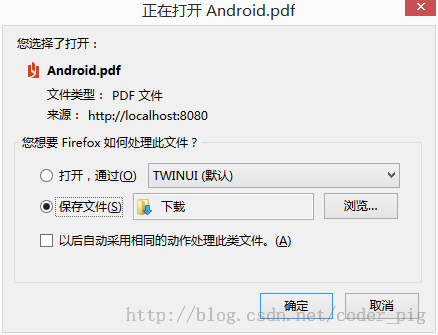
Summary of this section: This section introduces you to the request headers and response headers in Http, and also writes a few tips about adjusting the browser using response headers. some examples,
I believe that after this chapter, everyone has a better understanding of the Http protocol. In the next section, we will learn about the Http provided by Android.
Request method: HttpURLConnection! Okay, that’s it for this section, thank you~
By the way, download the demo of this section: Download HttpTest.zip |