Node.js Express Framework
Express Introduction
Express is a simple and flexible node.js web application framework that provides a series of powerful features to help you create various web applications and rich HTTP tools. .
Use Express to quickly build a fully functional website.
Express framework core features:
You can set up middleware to respond to HTTP requests.
Defines the routing table for performing different HTTP request actions.
You can dynamically render HTML pages by passing parameters to the template.
Install Express
Install Express and save it to the dependency list:
$ npm install express --save
The above command will install the Express framework in the current period In the node_modules directory of the directory, the express directory will be automatically created under the node_modules directory. The following important modules need to be installed together with the express framework:
body-parser - node.js middleware for processing JSON, Raw, Text and URL encoded data.
cookie-parser - This is a tool for parsing cookies. Through req.cookies, you can get the passed cookies and convert them into objects.
multer - node.js middleware, used to process form data with enctype="multipart/form-data" (setting the MIME encoding of the form).
$ npm install body-parser --save $ npm install cookie-parser --save $ npm install multer --save
The first Express framework instance
Next we use the Express framework to output "Hello World".
In the following example, we introduce the express module and respond with the "Hello World" string after the client initiates a request.
Create the express_demo.js file, the code is as follows:
//express_demo.js 文件var express = require('express');var app = express();app.get('/', function (req, res) { res.send('Hello World');})var server = app.listen(8081, function () { var host = server.address().address var port = server.address().port console.log("应用实例,访问地址为 http://%s:%s", host, port)})
Execute the above code:
$ node express_demo.js 应用实例,访问地址为 http://0.0.0.0:8081
Visit http://127.0.0.1:8081 in the browser, The result is shown below:

Request and response
Express application uses the parameters of the callback function: request and response Object to handle request and response data.
app.get('/', function (req, res) { // --})
request and response Detailed introduction of objects:
Request object - The request object represents an HTTP request, including Request query string, parameters, content, HTTP headers and other attributes. Common attributes are:
req.app: When the callback is an external file, use req.app to access the express instance
- ##req.baseUrl : Get the URL path of the route currently installed
- req.body / req.cookies : Get the "request body" / Cookies
- req.fresh / req.stale: Determine whether the request is still "fresh" ##req.hostname / req.ip: Get the host name and IP address
- req .originalUrl: Get the original request URL
- req.params: Get the routing parameters
- req.path: Get the request path
- req.protocol: Get the protocol type
- req.query: Get the query parameter string of the URL
req.route: Get the current matching route
req.subdomains: Get the subdomain name
req .accpets(): Check the request type of the Accept header of the request
req.acceptsCharsets/req.acceptsEncodings/req.acceptsLanguages
req. get(): Get the specified HTTP request header
req.is(): Determine the MIME type of the request header Content-Type
Response Object - The response object represents the HTTP response, that is, the HTTP response data sent to the client when a request is received. Common attributes are:
res.app: Same as req.app
res.append(): Append the specified HTTP header
res.set() after res.append() will reset the previously set header
res.cookie(name, value [, option ]): Set Cookie
opition: domain/expires/httpOnly/maxAge/path/secure/signed
res.clearCookie(): Clear Cookie
res.download(): Transfer the file with the specified path
res.get(): Return the specified HTTP header
res.json(): Transmit JSON response
res.jsonp(): Transmit JSONP response
res.location(): Only set the Location HTTP header of the response, without setting the status code or close response
res.redirect(): Set the Location HTTP header of the response, and set Status code 302
res.send(): Send HTTP response
res.sendFile(path[,options][,fn]) : Transfer the file with the specified path -Content-Type will be automatically set according to the file extension
- ##res.set(): Set the HTTP header, and the incoming object can set multiple headers at one time
- res.status(): Set the HTTP status code
- res.type(): Set the MIME type of Content-Type
RoutingWe have already understood the basic application of HTTP requests, and routing determines who (specified script) responds to the client request. In an HTTP request, we can extract the requested URL and GET/POST parameters through routing. Next we extend Hello World and add some functionality to handle more types of HTTP requests. Create the express_demo2.js file, the code is as follows:
var express = require('express');var app = express();// 主页输出 "Hello World"app.get('/', function (req, res) { console.log("主页 GET 请求"); res.send('Hello GET');})// POST 请求app.post('/', function (req, res) { console.log("主页 POST 请求"); res.send('Hello POST');})// /del_user 页面响应app.get('/del_user', function (req, res) { console.log("/del_user 响应 DELETE 请求"); res.send('删除页面');})// /list_user 页面 GET 请求app.get('/list_user', function (req, res) { console.log("/list_user GET 请求"); res.send('用户列表页面');})// 对页面 abcd, abxcd, ab123cd, 等响应 GET 请求app.get('/ab*cd', function(req, res) { console.log("/ab*cd GET 请求"); res.send('正则匹配');})var server = app.listen(8081, function () { var host = server.address().address var port = server.address().port console.log("应用实例,访问地址为 http://%s:%s", host, port)})Execute the above code:
$ node express_demo2.js 应用实例,访问地址为 http://0.0.0.0:8081Next you can try to access http://127.0.0.1:8081 Different addresses to see the effect. Access http://127.0.0.1:8081/list_user in the browser, the result is as shown below:
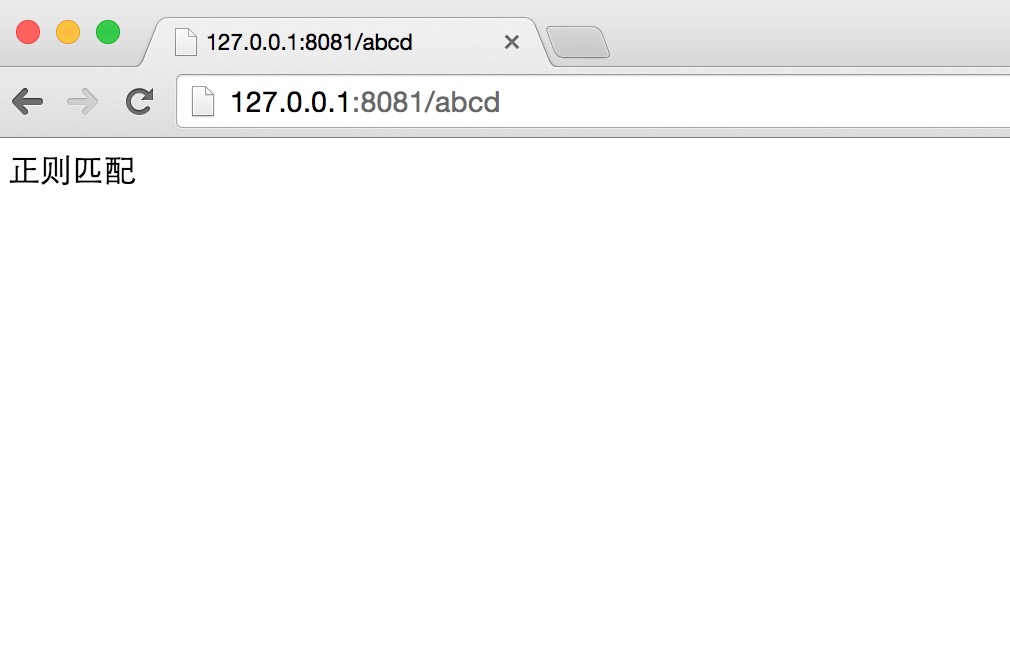
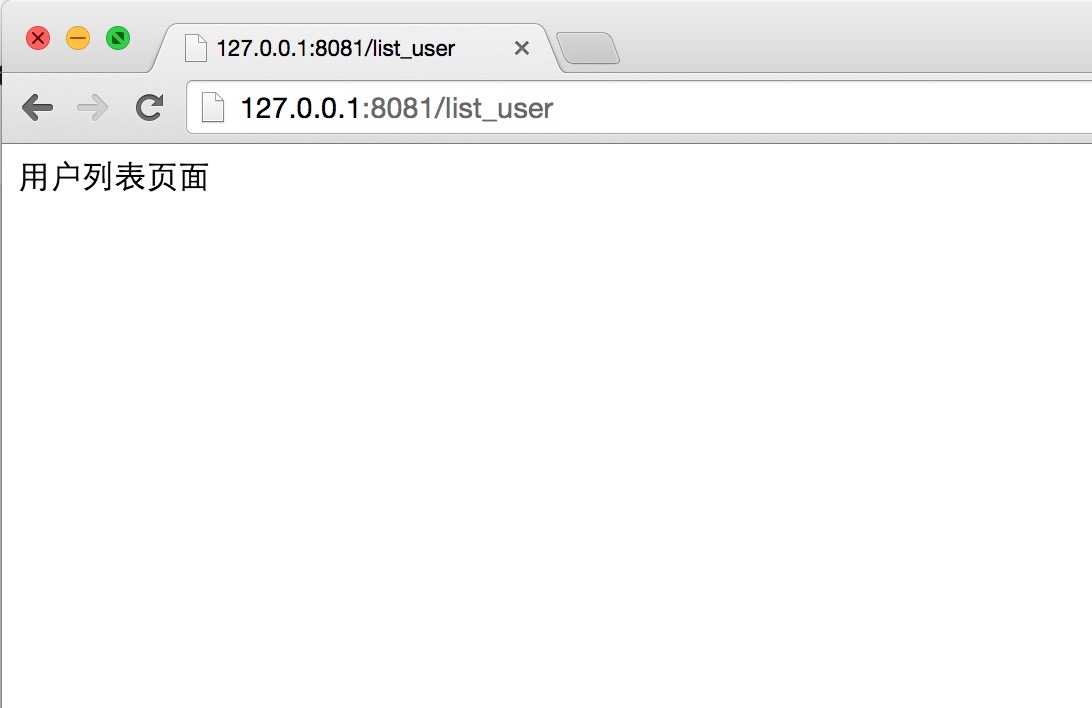
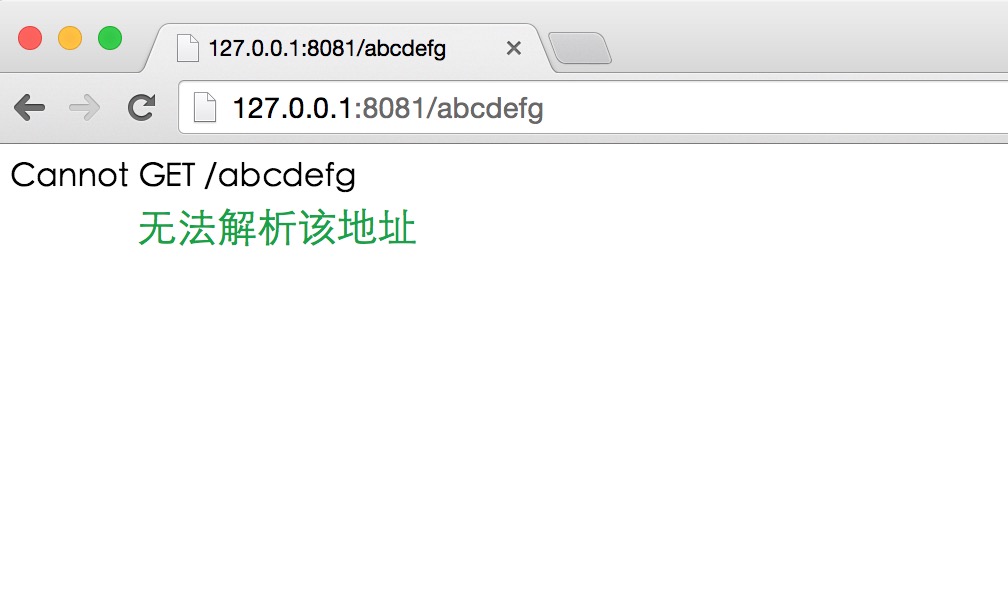
Static filesExpress provides built-in middleware
express.static to set static files such as images, CSS, JavaScript, etc.
You can use express.static middleware to set the static file path. For example, if you place images, CSS, and JavaScript files in the public directory, you can write:
app.use(express.static('public'));
We can put some images in the public/images directory, as follows:
node_modules server.jspublic/public/imagespublic/images/logo.png
Let us modify the "Hello Word" application to add the function of processing static files.
Create the express_demo3.js file, the code is as follows:
var express = require('express');var app = express();app.use(express.static('public'));app.get('/', function (req, res) { res.send('Hello World');})var server = app.listen(8081, function () { var host = server.address().address var port = server.address().port console.log("应用实例,访问地址为 http://%s:%s", host, port)})
Execute the above code:
$ node express_demo3.js 应用实例,访问地址为 http://0.0.0.0:8081
Execute the above code:
Access in the browser http://127.0.0.1:8081/images/logo.png (this example uses the logo of the rookie tutorial), the result is as shown below:

GET method
The following example demonstrates submitting two parameters through the GET method in the form. We can use the process_get router in the server.js file to process the input:
index.htm file code is as follows :
<html><body><form action="http://127.0.0.1:8081/process_get" method="GET">First Name: <input type="text" name="first_name"> <br>Last Name: <input type="text" name="last_name"><input type="submit" value="Submit"></form></body></html>
server.js file code is as follows:
var express = require('express');var app = express();app.use(express.static('public'));app.get('/index.htm', function (req, res) { res.sendFile( __dirname + "/" + "index.htm" );})app.get('/process_get', function (req, res) { // 输出 JSON 格式 response = { first_name:req.query.first_name, last_name:req.query.last_name }; console.log(response); res.end(JSON.stringify(response));})var server = app.listen(8081, function () { var host = server.address().address var port = server.address().port console.log("应用实例,访问地址为 http://%s:%s", host, port)})
Execute the above code:
node server.js 应用实例,访问地址为 http://0.0.0.0:8081
Browser access http://127.0.0.1:8081/index.htm, As shown in the figure:
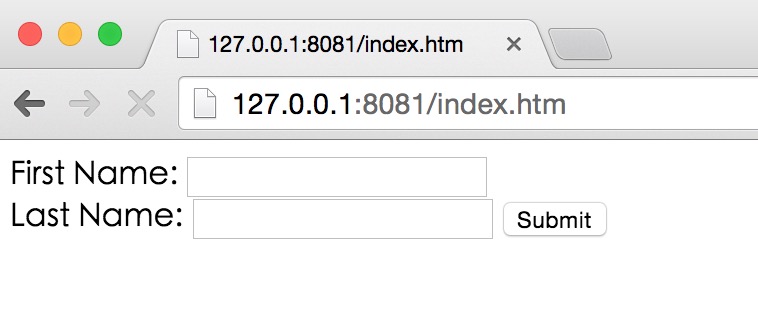
Now you can enter data into the form and submit it, as demonstrated below:

POST method
The following example Demonstrates submitting two parameters through the POST method in the form. We can use the process_post router in the server.js file to process the input:
index.htm file code is modified as follows:
<html><body><form action="http://127.0.0.1:8081/process_post" method="POST">First Name: <input type="text" name="first_name"> <br>Last Name: <input type="text" name="last_name"><input type="submit" value="Submit"></form></body></html>
server.js file code is modified as follows:
var express = require('express');var app = express();var bodyParser = require('body-parser');// 创建 application/x-www-form-urlencoded 编码解析var urlencodedParser = bodyParser.urlencoded({ extended: false })app.use(express.static('public'));app.get('/index.htm', function (req, res) { res.sendFile( __dirname + "/" + "index.htm" );})app.post('/process_post', urlencodedParser, function (req, res) { // 输出 JSON 格式 response = { first_name:req.body.first_name, last_name:req.body.last_name }; console.log(response); res.end(JSON.stringify(response));})var server = app.listen(8081, function () { var host = server.address().address var port = server.address().port console.log("应用实例,访问地址为 http://%s:%s", host, port)})
Execute the above code:
$ node server.js应用实例,访问地址为 http://0.0.0.0:8081
Browser access http://127.0.0.1:8081/index.htm, as shown in the figure As shown:
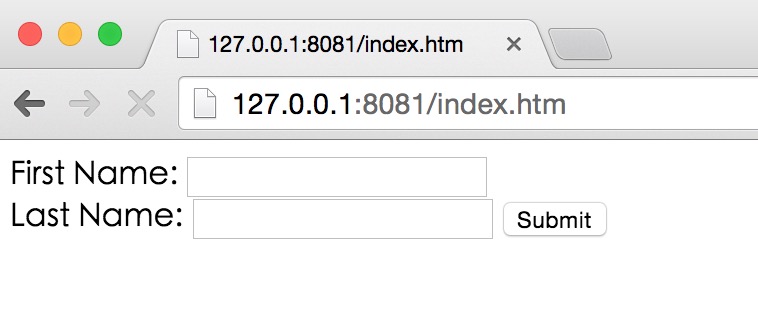
Now you can enter data into the form and submit it, as shown in the following demonstration:
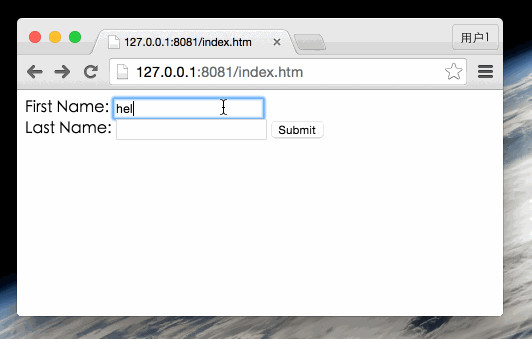
File upload
Let’s create a The form used to upload files, using the POST method, with the form enctype attribute set to multipart/form-data.
index.htm file code is modified as follows:
<html><head><title>文件上传表单</title></head><body><h3>文件上传:</h3>选择一个文件上传: <br /><form action="/file_upload" method="post" enctype="multipart/form-data"><input type="file" name="image" size="50" /><br /><input type="submit" value="上传文件" /></form></body></html>
server.js file code is modified as follows:
var express = require('express');var app = express();var fs = require("fs");var bodyParser = require('body-parser');var multer = require('multer');app.use(express.static('public'));app.use(bodyParser.urlencoded({ extended: false }));app.use(multer({ dest: '/tmp/'}).array('image'));app.get('/index.htm', function (req, res) { res.sendFile( __dirname + "/" + "index.htm" );})app.post('/file_upload', function (req, res) { console.log(req.files[0]); // 上传的文件信息 var des_file = __dirname + "/" + req.files[0].originalname; fs.readFile( req.files[0].path, function (err, data) { fs.writeFile(des_file, data, function (err) { if( err ){ console.log( err ); }else{ response = { message:'File uploaded successfully', filename:req.files[0].originalname }; } console.log( response ); res.end( JSON.stringify( response ) ); }); });})var server = app.listen(8081, function () { var host = server.address().address var port = server.address().port console.log("应用实例,访问地址为 http://%s:%s", host, port)})
Execute the above code:
$ node server.js 应用实例,访问地址为 http://0.0.0.0:8081
Browser access http://127.0.0.1:8081/index.htm, as shown in the figure:

Now you can enter data into the form and submit it, as shown below:
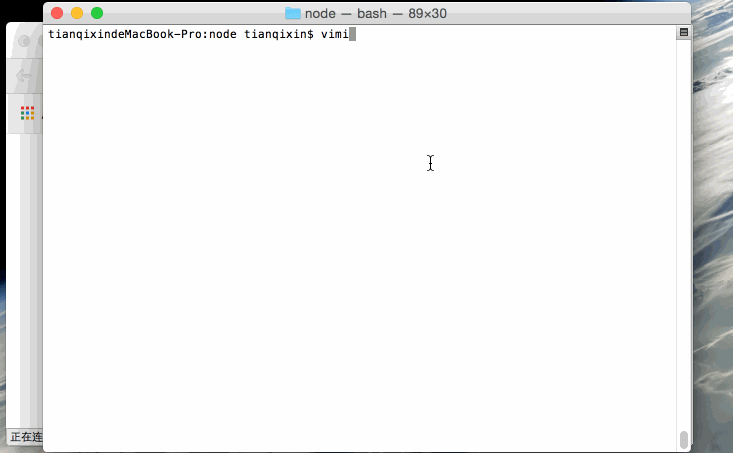
Cookie Management
We can use middleware to send cookie information to the Node.js server. The following code outputs the cookie information sent by the client:
// express_cookie.js 文件var express = require('express')var cookieParser = require('cookie-parser')var app = express()app.use(cookieParser())app.get('/', function(req, res) { console.log("Cookies: ", req.cookies)})app.listen(8081)
Execute the above code:
$ node express_cookie.js
Now you can access http://127.0.0.1:8081 and view the output of terminal information, as shown below:

Related information
Express official website: http://expressjs.com/
Express4.x API: http://expressjs.com/zh-cn/4x/api.html