PHP 편집자 Xigua는 프로그래밍 과정에서 프로그램이 중단되는 문제에 자주 직면합니다. 프로그램 정지는 프로그램이 실행 중에 오류 메시지 없이 갑자기 응답을 멈추는 것을 의미합니다. 이러한 상황은 종종 사람들을 무엇이 잘못되었는지 혼란스럽게 만듭니다. 이 프로그램이 정확히 왜 중단되나요? 이 문서에서는 프로그램 중단의 몇 가지 일반적인 원인을 살펴보고 문제 해결에 도움이 되는 솔루션을 제공합니다. 초보자이시든, 숙련된 개발자이시든, 이 내용이 여러분에게 도움이 될 것이라고 믿습니다.
질문 내용
가서 채널간 통신할 수 있는 코드가 있습니다. 원하는 대로 수행되는 것처럼 보이지만 결국에는 멈춥니다. 왜 멈추는지 진단하려고 합니다.
코드는 httpbin.org
를 사용하여 임의의 uuid를 얻은 다음 세마포어 채널과 속도 채널을 통해 설정한 동시성 및 속도 제한을 준수하면서 이를 게시합니다.
package main import ( "bytes" "encoding/json" "fmt" "io" "net/http" "sync" "time" ) type HttpBinGetRequest struct { url string } type HttpBinGetResponse struct { Uuid string `json:"uuid"` StatusCode int } type HttpBinPostRequest struct { url string uuid string // Item to post to API } type HttpBinPostResponse struct { Data string `json:"data"` StatusCode int } func main() { // Prepare GET requests for n requests var requests []*HttpBinGetRequest for i := 0; i < 10; i++ { uri := "https://httpbin.org/uuid" request := &HttpBinGetRequest{ url: uri, } requests = append(requests, request) } // Create semaphore and rate limit for the GET endpoint getSemaphore := make(chan struct{}, 10) getRate := make(chan struct{}, 10) defer close(getRate) defer close(getSemaphore) for i := 0; i < cap(getRate); i++ { getRate <- struct{}{} } go func() { // ticker corresponding to 1/nth of a second // where n = rate limit // basically (1000 / rps) * time.Millisecond ticker := time.NewTicker(100 * time.Millisecond) defer ticker.Stop() for range ticker.C { _, ok := <-getRate if !ok { return } } }() // Send our GET requests to obtain a random UUID respChan := make(chan HttpBinGetResponse) var wg sync.WaitGroup for _, request := range requests { wg.Add(1) // cnt := c // Go func to make request and receive the response go func(r *HttpBinGetRequest) { defer wg.Done() // Check the rate limiter and block if it is empty getRate <- struct{}{} // fmt.Printf("Request #%d at: %s\n", cnt, time.Now().UTC().Format("2006-01-02T15:04:05.000Z07:00")) resp, _ := get(r, getSemaphore) fmt.Printf("%+v\n", resp) // Place our response into the channel respChan <- *resp // fmt.Printf("%+v,%s\n", resp, time.Now().UTC().Format("2006-01-02T15:04:05.000Z07:00")) }(request) } // Set up for POST requests 10/s postSemaphore := make(chan struct{}, 10) postRate := make(chan struct{}, 10) defer close(postRate) defer close(postSemaphore) for i := 0; i < cap(postRate); i++ { postRate <- struct{}{} } go func() { // ticker corresponding to 1/nth of a second // where n = rate limit // basically (1000 / rps) * time.Millisecond ticker := time.NewTicker(100 * time.Millisecond) defer ticker.Stop() for range ticker.C { _, ok := <-postRate if !ok { return } } }() // Read responses as they become available for ele := range respChan { postReq := &HttpBinPostRequest{ url: "https://httpbin.org/post", uuid: ele.Uuid, } go func(r *HttpBinPostRequest) { postRate <- struct{}{} postResp, err := post(r, postSemaphore) if err != nil { fmt.Println(err) } fmt.Printf("%+v\n", postResp) }(postReq) } wg.Wait() close(respChan) } func get(hbgr *HttpBinGetRequest, sem chan struct{}) (*HttpBinGetResponse, error) { // Add a token to the semaphore sem <- struct{}{} // Remove token when function is complete defer func() { <-sem }() httpResp := &HttpBinGetResponse{} client := &http.Client{} req, err := http.NewRequest("GET", hbgr.url, nil) if err != nil { fmt.Println("error making request") return httpResp, err } req.Header = http.Header{ "accept": {"application/json"}, } resp, err := client.Do(req) if err != nil { fmt.Println(err) fmt.Println("error getting response") return httpResp, err } // Read Response body, err := io.ReadAll(resp.Body) if err != nil { fmt.Println("error reading response body") return httpResp, err } json.Unmarshal(body, &httpResp) httpResp.StatusCode = resp.StatusCode return httpResp, nil } // Method to post data to httpbin func post(hbr *HttpBinPostRequest, sem chan struct{}) (*HttpBinPostResponse, error) { // Add a token to the semaphore sem <- struct{}{} defer func() { <-sem }() httpResp := &HttpBinPostResponse{} client := &http.Client{} req, err := http.NewRequest("POST", hbr.url, bytes.NewBuffer([]byte(hbr.uuid))) if err != nil { fmt.Println("error making request") return httpResp, err } req.Header = http.Header{ "accept": {"application/json"}, } resp, err := client.Do(req) if err != nil { fmt.Println("error getting response") return httpResp, err } // Read Response body, err := io.ReadAll(resp.Body) if err != nil { fmt.Println("error reading response body") return httpResp, err } json.Unmarshal(body, &httpResp) httpResp.StatusCode = resp.StatusCode return httpResp, nil }
해결 방법
range
语句从代码末尾的 respchan
을 통해 콘텐츠를 읽고 계십니다. 이 코드는 채널이 닫힐 때까지 종료되지 않습니다. 이는 이 코드 블록 이후에 발생합니다.
그래서 프로그램은 절대 종료되지 않습니다. 왜냐하면 이 모든 로직이 동일한 고루틴에 있기 때문입니다.
프로그램이 종료되기 전에 모든 레코드가 처리되도록 수정하고 확인하려면 채널 읽기 코드를 기본 고루틴에 유지하고 대기/닫기 로직을 자체 고루틴에 넣으세요.
으아악루프의 모든 하위 고루틴을 최종 기다리도록 편집 range
- 대기 그룹을 사용하는 더 깔끔한 방법이 있을 수 있지만 빠른 수정 방법은 다음과 같습니다.
위 내용은 이 프로그램이 왜 정지되나요?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
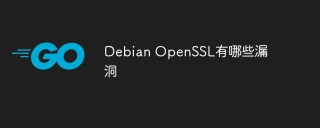
보안 통신에 널리 사용되는 오픈 소스 라이브러리로서 OpenSSL은 암호화 알고리즘, 키 및 인증서 관리 기능을 제공합니다. 그러나 역사적 버전에는 알려진 보안 취약점이 있으며 그 중 일부는 매우 유해합니다. 이 기사는 데비안 시스템의 OpenSSL에 대한 일반적인 취약점 및 응답 측정에 중점을 둘 것입니다. DebianopensSL 알려진 취약점 : OpenSSL은 다음과 같은 몇 가지 심각한 취약점을 경험했습니다. 심장 출혈 취약성 (CVE-2014-0160) :이 취약점은 OpenSSL 1.0.1 ~ 1.0.1F 및 1.0.2 ~ 1.0.2 베타 버전에 영향을 미칩니다. 공격자는이 취약점을 사용하여 암호화 키 등을 포함하여 서버에서 무단 읽기 민감한 정보를 사용할 수 있습니다.
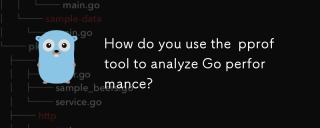
이 기사는 프로파일 링 활성화, 데이터 수집 및 CPU 및 메모리 문제와 같은 일반적인 병목 현상을 식별하는 등 GO 성능 분석을 위해 PPROF 도구를 사용하는 방법을 설명합니다.
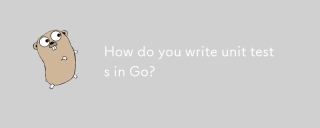
이 기사는 GO에서 단위 테스트 작성, 모범 사례, 조롱 기술 및 효율적인 테스트 관리를위한 도구를 다루는 것에 대해 논의합니다.
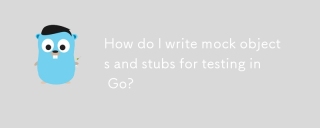
이 기사는 단위 테스트를 위해 이동 중에 모의와 스터브를 만드는 것을 보여줍니다. 인터페이스 사용을 강조하고 모의 구현의 예를 제공하며 모의 집중 유지 및 어설 션 라이브러리 사용과 같은 모범 사례에 대해 설명합니다. 기사
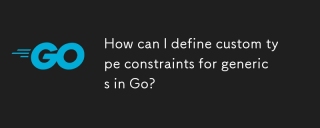
이 기사에서는 GO의 제네릭에 대한 사용자 정의 유형 제약 조건을 살펴 봅니다. 인터페이스가 일반 함수에 대한 최소 유형 요구 사항을 정의하여 유형 안전 및 코드 재사성을 향상시키는 방법에 대해 자세히 설명합니다. 이 기사는 또한 한계와 모범 사례에 대해 설명합니다
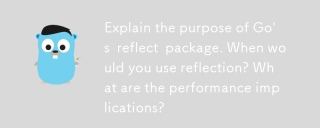
이 기사는 코드의 런타임 조작, 직렬화, 일반 프로그래밍에 유리한 런타임 조작에 사용되는 GO의 반사 패키지에 대해 설명합니다. 실행 속도가 느리고 메모리 사용이 높아짐, 신중한 사용 및 최고와 같은 성능 비용을 경고합니다.
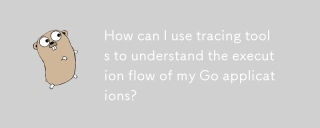
이 기사는 추적 도구를 사용하여 GO 응용 프로그램 실행 흐름을 분석합니다. 수동 및 자동 계측 기술, Jaeger, Zipkin 및 OpenTelemetry와 같은 도구 비교 및 효과적인 데이터 시각화를 강조합니다.
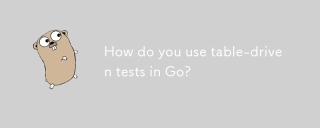
이 기사는 테스트 케이스 테이블을 사용하여 여러 입력 및 결과로 기능을 테스트하는 방법 인 GO에서 테이블 중심 테스트를 사용하는 것에 대해 설명합니다. 가독성 향상, 중복 감소, 확장 성, 일관성 및 A와 같은 이점을 강조합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구
