h x w의 그리드가 있다고 가정합니다. 그리드는 'initGrid'라는 2차원 배열로 표시되며 그리드의 각 셀은 '#' 또는 '.'으로 표시됩니다. '#'은 그리드에 장애물이 있음을 의미하고, '.'은 해당 셀에 경로가 있음을 의미합니다. 이제 로봇은 행 번호 x와 열 번호 y가 있는 그리드의 'c' 셀에 배치됩니다. 로봇은 행 번호가 p이고 열 번호가 q인 셀 'd'에서 다른 셀로 이동해야 합니다. 셀 좌표 c와 d는 모두 정수 쌍으로 제공됩니다. 이제 로봇은 다음과 같이 한 셀에서 다른 셀로 이동할 수 있습니다.
로봇이 이동하려는 셀이 현재 셀과 수직 또는 수평으로 인접해 있는 경우 로봇은 한 셀에서 다른 셀로 직접 걸어갈 수 있습니다.
로봇은 현재 위치를 중심으로 5x5 영역의 모든 셀로 점프할 수 있습니다.
로봇은 장애물이 없는 그리드의 다른 셀로만 이동할 수 있습니다. 로봇도 그리드를 떠날 수 없습니다.
로봇이 목표에 도달하는 데 걸리는 홉 수를 알아내야 합니다.
그러므로 입력이 h = 4, w = 4, c = {2, 1}, d = {4, 4}, initGrid = {"#...", ".##.", ".. .#", "..#."}이면 출력은 1이 됩니다. 로봇은 목적지에 도달하기 위해 한 번의 점프만 하면 됩니다.
이 문제를 해결하기 위해 다음 단계를 따릅니다.
N:= 100 Define intger pairs s and t. Define an array grid of size: N. Define an array dst of size: N x N. Define a struct node that contains integer values a, b, and e. Define a function check(), this will take a, b, return a >= 0 AND a < h AND b >= 0 AND b < w Define a function bfs(), this will take a, b, for initialize i := 0, when i < h, update (increase i by 1), do: for initialize j := 0, when j < w, update (increase j by 1), do: dst[i, j] := infinity dst[a, b] := 0 Define one deque doubleq Insert a node containing values {a, b, and dst[a, b]} at the end of doubleq while (not doubleq is empty), do: nd := first element of doubleq if e value of nd > dst[a value of nd, b value of nd], then: Ignore the following part, skip to the next iteration for initialize diffx := -2, when diffx <= 2, update (increase diffx by 1), do: for initialize diffy := -2, when diffy <= 2, update (increase diffy by 1), do: tm := |diffx + |diffy|| nx := a value of nd + diffx, ny = b value of nd + diffy if check(nx, ny) and grid[nx, ny] is same as '.', then: w := (if tm > 1, then 1, otherwise 0) if dst[a value of nd, b value of nd] + w < dst[nx, ny], then: dst[nx, ny] := dst[a value of nd, b value of nd] + w if w is same as 0, then: insert node containing values ({nx, ny, dst[nx, ny]}) at the beginning of doubleq. Otherwise insert node containing values ({nx, ny, dst[nx, ny]}) at the end of doubleq. s := c t := d (decrease first value of s by 1) (decrease second value of s by 1) (decrease first value of t by 1) (decrease second value of t by 1) for initialize i := 0, when i < h, update (increase i by 1), do: grid[i] := initGrid[i] bfs(first value of s, second value of s) print(if dst[first value of t, second value of t] is same as infinity, then -1, otherwise dst[first value of t, second value of t])
Example
더 나은 이해를 위해 아래 구현을 살펴보겠습니다. −
#include <bits/stdc++.h> using namespace std; const int INF = 1e9; #define N 100 int h, w; pair<int, int> s, t; string grid[N]; int dst[N][N]; struct node { int a, b, e; }; bool check(int a, int b) { return a >= 0 && a < h && b >= 0 && b < w; } void bfs(int a, int b) { for (int i = 0; i < h; i++) { for (int j = 0; j < w; j++) dst[i][j] = INF; } dst[a][b] = 0; deque<node> doubleq; doubleq.push_back({a, b, dst[a][b]}); while (!doubleq.empty()) { node nd = doubleq.front(); doubleq.pop_front(); if (nd.e > dst[nd.a][nd.b]) continue; for (int diffx = -2; diffx <= 2; diffx++) { for (int diffy = -2; diffy <= 2; diffy++) { int tm = abs(diffx) + abs(diffy); int nx = nd.a + diffx, ny = nd.b + diffy; if (check(nx, ny) && grid[nx][ny] == '.') { int w = (tm > 1) ? 1 : 0; if (dst[nd.a][nd.b] + w < dst[nx][ny]) { dst[nx][ny] = dst[nd.a][nd.b] + w; if (w == 0) doubleq.push_front({nx, ny, dst[nx][ny]}); else doubleq.push_back({nx, ny, dst[nx][ny]}); } } } } } } void solve(pair<int,int> c, pair<int, int> d, string initGrid[]){ s = c; t = d; s.first--, s.second--, t.first--, t.second--; for(int i = 0; i < h; i++) grid[i] = initGrid[i]; bfs(s.first, s.second); cout << (dst[t.first][t.second] == INF ? -1 : dst[t.first][t.second]) << '\n'; } int main() { h = 4, w = 4; pair<int,int> c = {2, 1}, d = {4, 4}; string initGrid[] = {"#...", ".##.", "...#", "..#."}; solve(c, d, initGrid); return 0; }
Input
4, 4, {2, 1}, {4, 4}, {"#...", ".##.", "...#", "..#."}
Output
1
위 내용은 로봇이 그리드의 특정 셀에 도달하기 위해 필요한 점프 횟수를 찾는 C++ 프로그램의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
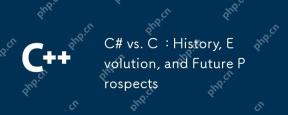
C#과 C의 역사와 진화는 독특하며 미래의 전망도 다릅니다. 1.C는 1983 년 Bjarnestroustrup에 의해 발명되어 객체 지향 프로그래밍을 C 언어에 소개했습니다. Evolution 프로세스에는 자동 키워드 소개 및 Lambda Expressions 소개 C 11, C 20 도입 개념 및 코 루틴과 같은 여러 표준화가 포함되며 향후 성능 및 시스템 수준 프로그래밍에 중점을 둘 것입니다. 2.C#은 2000 년 Microsoft에 의해 출시되었으며 C와 Java의 장점을 결합하여 진화는 단순성과 생산성에 중점을 둡니다. 예를 들어, C#2.0은 제네릭과 C#5.0 도입 된 비동기 프로그래밍을 소개했으며, 이는 향후 개발자의 생산성 및 클라우드 컴퓨팅에 중점을 둘 것입니다.
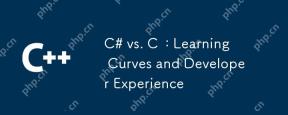
C# 및 C 및 개발자 경험의 학습 곡선에는 상당한 차이가 있습니다. 1) C#의 학습 곡선은 비교적 평평하며 빠른 개발 및 기업 수준의 응용 프로그램에 적합합니다. 2) C의 학습 곡선은 가파르고 고성능 및 저수준 제어 시나리오에 적합합니다.
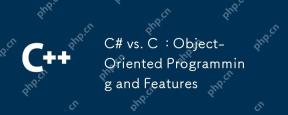
C# 및 C가 객체 지향 프로그래밍 (OOP)의 구현 및 기능에 상당한 차이가 있습니다. 1) C#의 클래스 정의 및 구문은 더 간결하고 LINQ와 같은 고급 기능을 지원합니다. 2) C는 시스템 프로그래밍 및 고성능 요구에 적합한 더 미세한 입상 제어를 제공합니다. 둘 다 고유 한 장점이 있으며 선택은 특정 응용 프로그램 시나리오를 기반으로해야합니다.
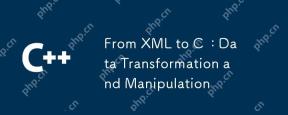
XML에서 C로 변환하고 다음 단계를 통해 수행 할 수 있습니다. 1) TinyxML2 라이브러리를 사용하여 XML 파일을 파싱하는 것은 2) C의 데이터 구조에 데이터를 매핑, 3) 데이터 운영을 위해 std :: 벡터와 같은 C 표준 라이브러리를 사용합니다. 이러한 단계를 통해 XML에서 변환 된 데이터를 효율적으로 처리하고 조작 할 수 있습니다.
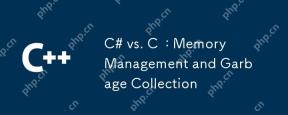
C#은 자동 쓰레기 수집 메커니즘을 사용하는 반면 C는 수동 메모리 관리를 사용합니다. 1. C#의 쓰레기 수집기는 메모리 누출 위험을 줄이기 위해 메모리를 자동으로 관리하지만 성능 저하로 이어질 수 있습니다. 2.C는 유연한 메모리 제어를 제공하며, 미세 관리가 필요한 애플리케이션에 적합하지만 메모리 누출을 피하기 위해주의해서 처리해야합니다.

C는 여전히 현대 프로그래밍과 관련이 있습니다. 1) 고성능 및 직접 하드웨어 작동 기능은 게임 개발, 임베디드 시스템 및 고성능 컴퓨팅 분야에서 첫 번째 선택이됩니다. 2) 스마트 포인터 및 템플릿 프로그래밍과 같은 풍부한 프로그래밍 패러다임 및 현대적인 기능은 유연성과 효율성을 향상시킵니다. 학습 곡선은 가파르지만 강력한 기능은 오늘날의 프로그래밍 생태계에서 여전히 중요합니다.
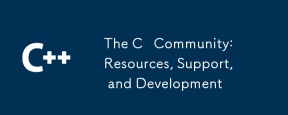
C 학습자와 개발자는 StackoverFlow, Reddit의 R/CPP 커뮤니티, Coursera 및 EDX 코스, GitHub의 오픈 소스 프로젝트, 전문 컨설팅 서비스 및 CPPCon에서 리소스와 지원을받을 수 있습니다. 1. StackoverFlow는 기술적 인 질문에 대한 답변을 제공합니다. 2. Reddit의 R/CPP 커뮤니티는 최신 뉴스를 공유합니다. 3. Coursera와 Edx는 공식적인 C 과정을 제공합니다. 4. LLVM 및 부스트 기술 향상과 같은 GitHub의 오픈 소스 프로젝트; 5. JetBrains 및 Perforce와 같은 전문 컨설팅 서비스는 기술 지원을 제공합니다. 6. CPPCON 및 기타 회의는 경력을 돕습니다
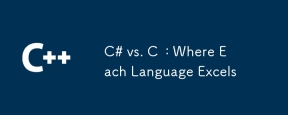
C#은 높은 개발 효율성과 크로스 플랫폼 지원이 필요한 프로젝트에 적합한 반면 C#은 고성능 및 기본 제어가 필요한 응용 프로그램에 적합합니다. 1) C#은 개발을 단순화하고, 쓰레기 수집 및 리치 클래스 라이브러리를 제공하며, 엔터프라이즈 레벨 애플리케이션에 적합합니다. 2) C는 게임 개발 및 고성능 컴퓨팅에 적합한 직접 메모리 작동을 허용합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SecList
SecLists는 최고의 보안 테스터의 동반자입니다. 보안 평가 시 자주 사용되는 다양한 유형의 목록을 한 곳에 모아 놓은 것입니다. SecLists는 보안 테스터에게 필요할 수 있는 모든 목록을 편리하게 제공하여 보안 테스트를 더욱 효율적이고 생산적으로 만드는 데 도움이 됩니다. 목록 유형에는 사용자 이름, 비밀번호, URL, 퍼징 페이로드, 민감한 데이터 패턴, 웹 셸 등이 포함됩니다. 테스터는 이 저장소를 새로운 테스트 시스템으로 간단히 가져올 수 있으며 필요한 모든 유형의 목록에 액세스할 수 있습니다.

PhpStorm 맥 버전
최신(2018.2.1) 전문 PHP 통합 개발 도구

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기
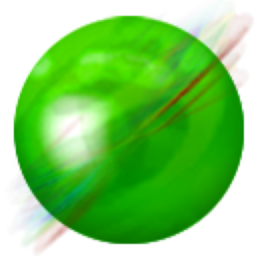
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경
