스패닝 트리는 모든 정점을 연결하는 방향성 무방향 그래프 하위 그래프입니다. 그래프에는 스패닝 트리가 많이 있을 수 있습니다. 각 그래프의 최소 스패닝 트리(MST)는 다른 모든 스패닝 트리와 동일하거나 작은 가중치를 갖습니다. 스패닝 트리의 가장자리에 가중치가 할당되고 그 합은 각 가장자리에 할당된 가중치입니다. V는 그래프의 정점 수이므로 최소 스패닝 트리는 간선 수(V - 1)를 가지며, 여기서 V는 간선 수입니다.
Kruskal 알고리즘을 사용하여 최소 스패닝 트리를 찾습니다
모든 모서리는 가중치를 기준으로 내림차순이 아닌 순서로 정렬되어야 합니다.
가장 작은 면을 선택하세요. 루프가 형성되지 않으면 가장자리가 포함됩니다.
스패닝 트리에 (V-1) 모서리가 생길 때까지 2단계를 수행해야 합니다.
이 경우 탐욕스러운 접근 방식을 사용하라는 지시를 받습니다. 그리디 옵션은 가중치가 가장 작은 모서리를 선택하는 것입니다. 예를 들어 이 그래프의 최소 스패닝 트리는 (9-1)= 8개의 모서리입니다.
After sorting: Weight Src Dest 21 27 26 22 28 22 22 26 25 24 20 21 24 22 25 26 28 26 27 22 23 27 27 28 28 20 27 28 21 22 29 23 24 30 25 24 31 21 27 34 23 25
이제 정렬을 기반으로 모든 가장자리를 선택해야 합니다.
루프가 형성되지 않았기 때문에 가장자리 26-27->가 포함됩니다.
루프가 형성되지 않았기 때문에 가장자리가 28-22->를 포함합니다.
루프가 형성되지 않았기 때문에 가장자리 26-25->를 포함합니다.
Edge 20-21->는 루프가 형성되지 않았기 때문에 포함되었습니다.
Edge 22-25->는 루프가 형성되지 않았기 때문에 포함되었습니다.
Edge 28-26-> 루프 형성으로 인해 삭제
Edge 22-23-> > 루프가 형성되지 않아 포함됨
Edge 27-28-> 루프 형성으로 인해 삭제
Edge 20 -27->포함 루프가 형성되지 않았기 때문에
Edge 21-22->루프가 형성되었기 때문에 폐기
Edge 23-24->루프가 형성되지 않았기 때문에 포함
에지 개수가 (V-1)이므로 알고리즘은 여기서 끝납니다.
Example
#include <stdio.h> #include <stdlib.h> #include <string.h> struct Edge { int src, dest, weight; }; struct Graph { int V, E; struct Edge* edge; }; struct Graph* createGraph(int V, int E){ struct Graph* graph = (struct Graph*)(malloc(sizeof(struct Graph))); graph->V = V; graph->E = E; graph->edge = (struct Edge*)malloc(sizeof( struct Edge)*E); return graph; } struct subset { int parent; int rank; }; int find(struct subset subsets[], int i){ if (subsets[i].parent != i) subsets[i].parent = find(subsets, subsets[i].parent); return subsets[i].parent; } void Union(struct subset subsets[], int x, int y){ int xroot = find(subsets, x); int yroot = find(subsets, y); if (subsets[xroot].rank < subsets[yroot].rank) subsets[xroot].parent = yroot; else if (subsets[xroot].rank > subsets[yroot].rank) subsets[yroot].parent = xroot; else{ subsets[yroot].parent = xroot; subsets[xroot].rank++; } } int myComp(const void* a, const void* b){ struct Edge* a1 = (struct Edge*)a; struct Edge* b1 = (struct Edge*)b; return a1->weight > b1->weight; } void KruskalMST(struct Graph* graph){ int V = graph->V; struct Edge result[V]; int e = 0; int i = 0; qsort(graph->edge, graph->E, sizeof(graph->edge[0]), myComp); struct subset* subsets = (struct subset*)malloc(V * sizeof(struct subset)); for (int v = 0; v < V; ++v) { subsets[v].parent = v; subsets[v].rank = 0; } while (e < V - 1 && i < graph->E) { struct Edge next_edge = graph->edge[i++]; int x = find(subsets, next_edge.src); int y = find(subsets, next_edge.dest); if (x != y) { result[e++] = next_edge; Union(subsets, x, y); } } printf("Following are the edges in the constructed MST\n"); int minimumCost = 0; for (i = 0; i < e; ++i){ printf("%d -- %d == %d\n", result[i].src, result[i].dest, result[i].weight); minimumCost += result[i].weight; } printf("Minimum Cost Spanning tree : %d",minimumCost); return; } int main(){ /* Let us create the following weighted graph 30 0--------1 | \ | 26| 25\ |15 | \ | 22--------23 24 */ int V = 24; int E = 25; struct Graph* graph = createGraph(V, E); graph->edge[0].src = 20; graph->edge[0].dest = 21; graph->edge[0].weight = 30; graph->edge[1].src = 20; graph->edge[1].dest = 22; graph->edge[1].weight = 26; graph->edge[2].src = 20; graph->edge[2].dest = 23; graph->edge[2].weight = 25; graph->edge[3].src = 21; graph->edge[3].dest = 23; graph->edge[3].weight = 35; graph->edge[4].src = 22; graph->edge[4].dest = 23; graph->edge[4].weight = 24; KruskalMST(graph); return 0; }
Output
Following are the edges in the constructed MST 22 -- 23 == 24 20 -- 23 == 25 20 -- 21 == 30 Minimum Cost Spanning tree : 79
결론
이 튜토리얼에서는 Kruskal의 최소 스패닝 트리 알고리즘(Greedy 방법 및 C++ 코드)을 사용하여 이 문제를 해결하는 방법을 보여줍니다. 이 코드를 Java, Python 및 기타 언어로 작성할 수도 있습니다. 크루스칼(Kruskal)의 개념을 모델로 한 것이다. 이 프로그램은 주어진 그래프에서 가장 짧은 스패닝 트리를 찾습니다. 이 튜토리얼이 도움이 되었기를 바랍니다.
위 내용은 Kruskal의 최소 스패닝 트리 알고리즘 - C++의 탐욕 알고리즘의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
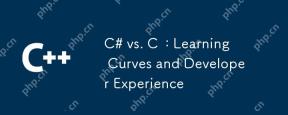
C# 및 C 및 개발자 경험의 학습 곡선에는 상당한 차이가 있습니다. 1) C#의 학습 곡선은 비교적 평평하며 빠른 개발 및 기업 수준의 응용 프로그램에 적합합니다. 2) C의 학습 곡선은 가파르고 고성능 및 저수준 제어 시나리오에 적합합니다.
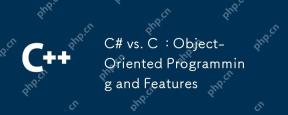
C# 및 C가 객체 지향 프로그래밍 (OOP)의 구현 및 기능에 상당한 차이가 있습니다. 1) C#의 클래스 정의 및 구문은 더 간결하고 LINQ와 같은 고급 기능을 지원합니다. 2) C는 시스템 프로그래밍 및 고성능 요구에 적합한 더 미세한 입상 제어를 제공합니다. 둘 다 고유 한 장점이 있으며 선택은 특정 응용 프로그램 시나리오를 기반으로해야합니다.
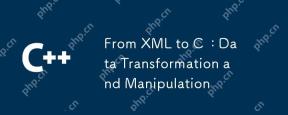
XML에서 C로 변환하고 다음 단계를 통해 수행 할 수 있습니다. 1) TinyxML2 라이브러리를 사용하여 XML 파일을 파싱하는 것은 2) C의 데이터 구조에 데이터를 매핑, 3) 데이터 운영을 위해 std :: 벡터와 같은 C 표준 라이브러리를 사용합니다. 이러한 단계를 통해 XML에서 변환 된 데이터를 효율적으로 처리하고 조작 할 수 있습니다.
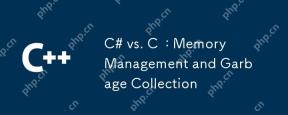
C#은 자동 쓰레기 수집 메커니즘을 사용하는 반면 C는 수동 메모리 관리를 사용합니다. 1. C#의 쓰레기 수집기는 메모리 누출 위험을 줄이기 위해 메모리를 자동으로 관리하지만 성능 저하로 이어질 수 있습니다. 2.C는 유연한 메모리 제어를 제공하며, 미세 관리가 필요한 애플리케이션에 적합하지만 메모리 누출을 피하기 위해주의해서 처리해야합니다.

C는 여전히 현대 프로그래밍과 관련이 있습니다. 1) 고성능 및 직접 하드웨어 작동 기능은 게임 개발, 임베디드 시스템 및 고성능 컴퓨팅 분야에서 첫 번째 선택이됩니다. 2) 스마트 포인터 및 템플릿 프로그래밍과 같은 풍부한 프로그래밍 패러다임 및 현대적인 기능은 유연성과 효율성을 향상시킵니다. 학습 곡선은 가파르지만 강력한 기능은 오늘날의 프로그래밍 생태계에서 여전히 중요합니다.
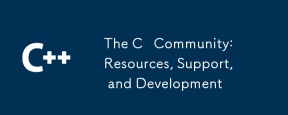
C 학습자와 개발자는 StackoverFlow, Reddit의 R/CPP 커뮤니티, Coursera 및 EDX 코스, GitHub의 오픈 소스 프로젝트, 전문 컨설팅 서비스 및 CPPCon에서 리소스와 지원을받을 수 있습니다. 1. StackoverFlow는 기술적 인 질문에 대한 답변을 제공합니다. 2. Reddit의 R/CPP 커뮤니티는 최신 뉴스를 공유합니다. 3. Coursera와 Edx는 공식적인 C 과정을 제공합니다. 4. LLVM 및 부스트 기술 향상과 같은 GitHub의 오픈 소스 프로젝트; 5. JetBrains 및 Perforce와 같은 전문 컨설팅 서비스는 기술 지원을 제공합니다. 6. CPPCON 및 기타 회의는 경력을 돕습니다
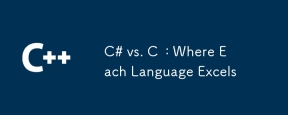
C#은 높은 개발 효율성과 크로스 플랫폼 지원이 필요한 프로젝트에 적합한 반면 C#은 고성능 및 기본 제어가 필요한 응용 프로그램에 적합합니다. 1) C#은 개발을 단순화하고, 쓰레기 수집 및 리치 클래스 라이브러리를 제공하며, 엔터프라이즈 레벨 애플리케이션에 적합합니다. 2) C는 게임 개발 및 고성능 컴퓨팅에 적합한 직접 메모리 작동을 허용합니다.
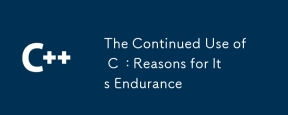
C 지속적인 사용 이유에는 고성능, 광범위한 응용 및 진화 특성이 포함됩니다. 1) 고효율 성능 : C는 메모리 및 하드웨어를 직접 조작하여 시스템 프로그래밍 및 고성능 컴퓨팅에서 훌륭하게 수행합니다. 2) 널리 사용 : 게임 개발, 임베디드 시스템 등의 분야에서의 빛나기.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
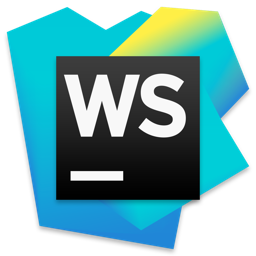
WebStorm Mac 버전
유용한 JavaScript 개발 도구

SublimeText3 Linux 새 버전
SublimeText3 Linux 최신 버전

Atom Editor Mac 버전 다운로드
가장 인기 있는 오픈 소스 편집기

SublimeText3 영어 버전
권장 사항: Win 버전, 코드 프롬프트 지원!

Eclipse용 SAP NetWeaver 서버 어댑터
Eclipse를 SAP NetWeaver 애플리케이션 서버와 통합합니다.
