MySQL에는 두 가지 연결 방법이 있습니다. 하나는 직접 연결이고 다른 하나는 풀 연결입니다.
혼란을 없애기 위해 직접 연결을 위한 코드를 다음과 같이 간략하게 작성했습니다.
var mysql = require('mysql'); var connection = mysql.createConnection({ host : 'localhost', user : 'ac', password : '123456', database : 'textPro' }); connection.connect(); connection.query('SELECT * from1 userInfo', function (error, results, fields) { if (error) throw error; console.log('The solution is: ', results); }); connection.end();
createConnection과 createPool의 차이점은 다음과 같습니다. createPool(연결 풀) 연결을 통해 서버 데이터를 보다 합리적으로 활용하고 데이터 사용량을 줄일 수 있습니다. 데이터 낭비
설치 후 db/index.js에 쓰기 시작:
const mysql = require("mysql") //创建连接池 const db= mysql.createPool({ host : 'localhost', //连接主机 port : 3306, //端口号 database : 'test', //连接的是哪一个库 user : 'root', //用户名 password : '', //密码 connectionLimit : 50, //用于指定连接池中最大的链接数,默认属性值为10. //用于指定允许挂起的最大连接数,如果挂起的连接数超过该数值,就会立即抛出一个错误, //默认属性值为0.代表不允许被挂起的最大连接数。 queueLimit:3 })
연결 풀을 설정한 후 연결 풀 개체의 getConnection 메소드를 사용하여 연결 풀에서 직접 연결을 가져올 수 있습니다. 연결 풀에 사용 가능한 연결이 없으면 암시적으로 데이터베이스 연결을 설정합니다.
const mysql = require("mysql") //创建连接池 const db= mysql.createPool({ host : 'localhost', //连接主机 port : 3306, //端口号 database : 'test', //连接的是哪一个库 user : 'root', //用户名 password : '', //密码 connectionLimit : 50, //用于指定连接池中最大的链接数,默认属性值为10. //用于指定允许挂起的最大连接数,如果挂起的连接数超过该数值,就会立即抛出一个错误, //默认属性值为0.代表不允许被挂起的最大连接数。 queueLimit:3 }) module.exports.query = (sql, values.callback) => { //err: 该参数是指操作失败时的错误对象。 //connection: 该值为一个对象,代表获取到的连接对象。当连接失败时,该值为undefined。 db.getConnection(function(err, connection) { if (err) { console.log('与mysql数据库建立连接失败'); pool.releaseConnection(); //释放链接 } else { console.log('与mysql数据库建立连接成功'); connection.query(sql,values,(err, res) => { if (err) { console.log('执行sql语句失败,查询数据失败'); //connection.release() 当一个连接不需要使用时,使用该方法将其归还到连接池中 release释放 connection.release(); callback(err,null) } else { console.log('执行sql语句成功'); callback(null,res) //pool.end() 当一个连接池不需要使用时,可以使用该方法关闭连接池 pool.end(); } }) } }) }
db 모듈 소개 및 호출
const query=require('./db').query; let sql='SELECT * FROM class WHERE class_id=? AND class_name=?' let userId=1; let userName='阿辰'; query(sql,[userId,userName],(err,res)=>{ if(err){ console.log('发生了错误***',err) return } console.log('找到了',res) })
const query=require('./db').query 와 const query=require('./db') 차이점
첫 번째 작성 방법 "./db" 모듈만 가져옵니다. 내보낸 다른 항목을 사용해야 하는 경우 해당 항목을 다시 가져와야 합니다.
두 번째 작성 방법은 "./db" 모듈에서 내보낸 모든 항목을 가져오며, 코드에서 내보낸 다른 항목을 반복적으로 가져올 필요가 없습니다.
위 내용은 node+mysql 데이터베이스 연결 풀 연결 방법은 무엇인가요?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
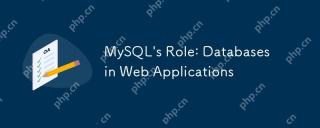
웹 응용 프로그램에서 MySQL의 주요 역할은 데이터를 저장하고 관리하는 것입니다. 1. MySQL은 사용자 정보, 제품 카탈로그, 트랜잭션 레코드 및 기타 데이터를 효율적으로 처리합니다. 2. SQL 쿼리를 통해 개발자는 데이터베이스에서 정보를 추출하여 동적 컨텐츠를 생성 할 수 있습니다. 3.mysql은 클라이언트-서버 모델을 기반으로 작동하여 허용 가능한 쿼리 속도를 보장합니다.
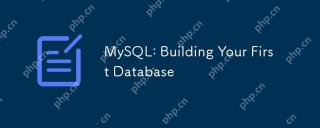
MySQL 데이터베이스를 구축하는 단계에는 다음이 포함됩니다. 1. 데이터베이스 및 테이블 작성, 2. 데이터 삽입 및 3. 쿼리를 수행하십시오. 먼저 CreateAbase 및 CreateTable 문을 사용하여 데이터베이스 및 테이블을 작성한 다음 InsertInto 문을 사용하여 데이터를 삽입 한 다음 최종적으로 SELECT 문을 사용하여 데이터를 쿼리하십시오.
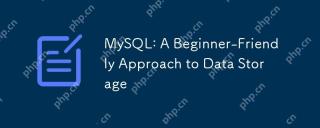
MySQL은 사용하기 쉽고 강력하기 때문에 초보자에게 적합합니다. 1.MySQL은 관계형 데이터베이스이며 CRUD 작업에 SQL을 사용합니다. 2. 설치가 간단하고 루트 사용자 비밀번호를 구성해야합니다. 3. 삽입, 업데이트, 삭제 및 선택하여 데이터 작업을 수행하십시오. 4. Orderby, Where and Join은 복잡한 쿼리에 사용될 수 있습니다. 5. 디버깅은 구문을 확인하고 쿼리를 분석하기 위해 설명을 사용해야합니다. 6. 최적화 제안에는 인덱스 사용, 올바른 데이터 유형 선택 및 우수한 프로그래밍 습관이 포함됩니다.
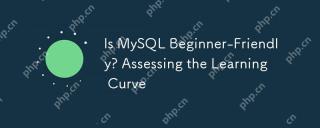
MySQL은 다음과 같은 초보자에게 적합합니다. 1) 설치 및 구성이 쉽고, 2) 풍부한 학습 리소스, 3) 직관적 인 SQL 구문, 4) 강력한 도구 지원. 그럼에도 불구하고 초보자는 데이터베이스 디자인, 쿼리 최적화, 보안 관리 및 데이터 백업과 같은 과제를 극복해야합니다.
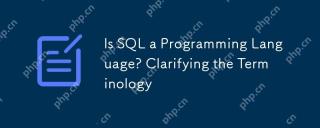
예, sqlisaprogramminglanguages-pecializedfordatamanagement.1) 그것은 초점을 맞추고, 초점을 맞추고, 초점을 맞추고, sqlisessentialforquerying, 삽입, 업데이트 및 adletingdataindataindationaldatabase.3) weburer infriendly, itrequires-quirestoamtoavase
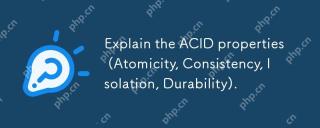
산성 속성에는 원자력, 일관성, 분리 및 내구성이 포함되며 데이터베이스 설계의 초석입니다. 1. 원자력은 거래가 완전히 성공적이거나 완전히 실패하도록합니다. 2. 일관성은 거래 전후에 데이터베이스가 일관성을 유지하도록합니다. 3. 격리는 거래가 서로를 방해하지 않도록합니다. 4. 지속성은 거래 제출 후 데이터가 영구적으로 저장되도록합니다.
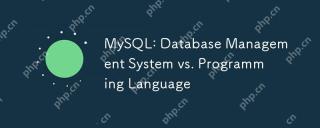
MySQL은 데이터베이스 관리 시스템 (DBMS) 일뿐 만 아니라 프로그래밍 언어와 밀접한 관련이 있습니다. 1) DBMS로서 MySQL은 데이터를 저장, 구성 및 검색하는 데 사용되며 인덱스 최적화는 쿼리 성능을 향상시킬 수 있습니다. 2) SQL과 같은 ORM 도구를 사용하여 Python에 내장 된 SQL과 프로그래밍 언어를 결합하면 작업을 단순화 할 수 있습니다. 3) 성능 최적화에는 인덱싱, 쿼리, 캐싱, 라이브러리 및 테이블 부서 및 거래 관리가 포함됩니다.
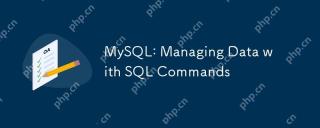
MySQL은 SQL 명령을 사용하여 데이터를 관리합니다. 1. 기본 명령에는 선택, 삽입, 업데이트 및 삭제가 포함됩니다. 2. 고급 사용에는 조인, 하위 쿼리 및 집계 함수가 포함됩니다. 3. 일반적인 오류에는 구문, 논리 및 성능 문제가 포함됩니다. 4. 최적화 팁에는 인덱스 사용, 선택*을 피하고 한계 사용이 포함됩니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

맨티스BT
Mantis는 제품 결함 추적을 돕기 위해 설계된 배포하기 쉬운 웹 기반 결함 추적 도구입니다. PHP, MySQL 및 웹 서버가 필요합니다. 데모 및 호스팅 서비스를 확인해 보세요.
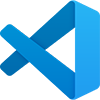
VSCode Windows 64비트 다운로드
Microsoft에서 출시한 강력한 무료 IDE 편집기
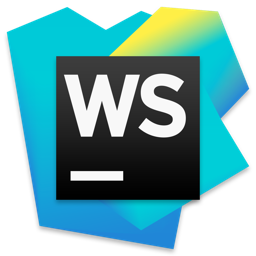
WebStorm Mac 버전
유용한 JavaScript 개발 도구
