PHP에서 계산기 만들기
PHP의 태그는 특히 원래 표기법을 보존할 때 어려울 수 있습니다. 문자열을 계산된 값으로 바꾸는 무차별 접근 방식은 처음에는 실행 가능해 보이지만 비효율적입니다.
Shunting Yard 알고리즘 활용
더 효과적인 솔루션은 Shunting입니다. 야드 알고리즘. 입력 표현식을 역폴란드 표기법(RPN)으로 변환합니다. RPN은 피연산자 바로 뒤에 연산자가 오기 때문에 계산을 단순화합니다.
구현 예
다음 코드는 PHP에서 Shunting Yard 알고리즘의 구현을 제공합니다.
class Expression { protected $tokens; protected $output; // Shunting Yard Algorithm public function shuntingYard($input) { $stack = []; $tokens = $this->tokenize($input); foreach ($tokens as $token) { if (is_numeric($token)) { $this->output[] = $token; } else { switch ($token) { case '(': $stack[] = $token; break; case ')': while ($stack && end($stack) != '(') { $this->output[] = array_pop($stack); } if (!empty($stack)) { array_pop($stack); } break; default: while ($stack && $this->precedence($token) precedence(end($stack))) { $this->output[] = array_pop($stack); } $stack[] = $token; break; } } } while (!empty($stack)) { $this->output[] = array_pop($stack); } } // Tokenize the input public function tokenize($input) { preg_match_all('~\d+|~|~U', $input, $tokens); return $tokens[0]; } // Operator precedence public function precedence($operator) { switch ($operator) { case '+': case '-': return 1; case '*': case '/': return 2; default: return 0; } } // Evaluate the RPN expression public function evaluate() { $stack = []; foreach ($this->output as $token) { if (is_numeric($token)) { $stack[] = $token; } else { $operand2 = array_pop($stack); $operand1 = array_pop($stack); switch ($token) { case '+': $result = $operand1 + $operand2; break; case '-': $result = $operand1 - $operand2; break; case '*': $result = $operand1 * $operand2; break; case '/': $result = $operand1 / $operand2; break; } $stack[] = $result; } } return array_pop($stack); } } $expression = new Expression(); $expression->shuntingYard('8*(5+1)'); $result = $expression->evaluate(); echo $result; // Output: 48
이 코드는 입력을 RPN으로 변환하고 결과 스택을 평가한 후 최종 결과.
위 내용은 Shunting Yard 알고리즘이 PHP에서 수학적 표현 평가를 어떻게 단순화할 수 있습니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
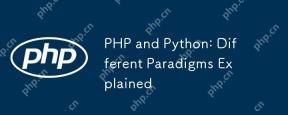
PHP는 주로 절차 적 프로그래밍이지만 객체 지향 프로그래밍 (OOP)도 지원합니다. Python은 OOP, 기능 및 절차 프로그래밍을 포함한 다양한 패러다임을 지원합니다. PHP는 웹 개발에 적합하며 Python은 데이터 분석 및 기계 학습과 같은 다양한 응용 프로그램에 적합합니다.
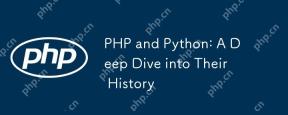
PHP는 1994 년에 시작되었으며 Rasmuslerdorf에 의해 개발되었습니다. 원래 웹 사이트 방문자를 추적하는 데 사용되었으며 점차 서버 측 스크립팅 언어로 진화했으며 웹 개발에 널리 사용되었습니다. Python은 1980 년대 후반 Guidovan Rossum에 의해 개발되었으며 1991 년에 처음 출시되었습니다. 코드 가독성과 단순성을 강조하며 과학 컴퓨팅, 데이터 분석 및 기타 분야에 적합합니다.
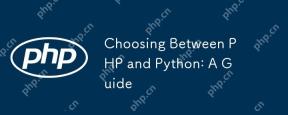
PHP는 웹 개발 및 빠른 프로토 타이핑에 적합하며 Python은 데이터 과학 및 기계 학습에 적합합니다. 1.PHP는 간단한 구문과 함께 동적 웹 개발에 사용되며 빠른 개발에 적합합니다. 2. Python은 간결한 구문을 가지고 있으며 여러 분야에 적합하며 강력한 라이브러리 생태계가 있습니다.
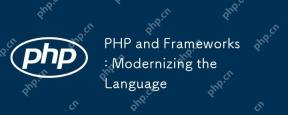
PHP는 현대화 프로세스에서 많은 웹 사이트 및 응용 프로그램을 지원하고 프레임 워크를 통해 개발 요구에 적응하기 때문에 여전히 중요합니다. 1.PHP7은 성능을 향상시키고 새로운 기능을 소개합니다. 2. Laravel, Symfony 및 Codeigniter와 같은 현대 프레임 워크는 개발을 단순화하고 코드 품질을 향상시킵니다. 3. 성능 최적화 및 모범 사례는 응용 프로그램 효율성을 더욱 향상시킵니다.
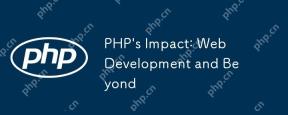
phphassignificallyimpactedwebdevelopmentandextendsbeyondit
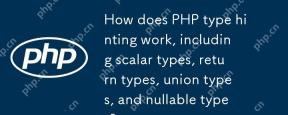
PHP 유형은 코드 품질과 가독성을 향상시키기위한 프롬프트입니다. 1) 스칼라 유형 팁 : PHP7.0이므로 int, float 등과 같은 기능 매개 변수에 기본 데이터 유형을 지정할 수 있습니다. 2) 반환 유형 프롬프트 : 기능 반환 값 유형의 일관성을 확인하십시오. 3) Union 유형 프롬프트 : PHP8.0이므로 기능 매개 변수 또는 반환 값에 여러 유형을 지정할 수 있습니다. 4) Nullable 유형 프롬프트 : NULL 값을 포함하고 널 값을 반환 할 수있는 기능을 포함 할 수 있습니다.
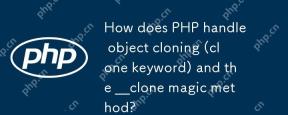
PHP에서는 클론 키워드를 사용하여 객체 사본을 만들고 \ _ \ _ Clone Magic 메소드를 통해 클로닝 동작을 사용자 정의하십시오. 1. 복제 키워드를 사용하여 얕은 사본을 만들어 객체의 속성을 복제하지만 객체의 속성은 아닙니다. 2. \ _ \ _ 클론 방법은 얕은 복사 문제를 피하기 위해 중첩 된 물체를 깊이 복사 할 수 있습니다. 3. 복제의 순환 참조 및 성능 문제를 피하고 클로닝 작업을 최적화하여 효율성을 향상시키기 위해주의를 기울이십시오.
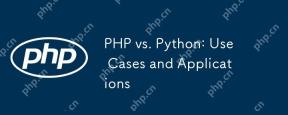
PHP는 웹 개발 및 컨텐츠 관리 시스템에 적합하며 Python은 데이터 과학, 기계 학습 및 자동화 스크립트에 적합합니다. 1.PHP는 빠르고 확장 가능한 웹 사이트 및 응용 프로그램을 구축하는 데 잘 작동하며 WordPress와 같은 CMS에서 일반적으로 사용됩니다. 2. Python은 Numpy 및 Tensorflow와 같은 풍부한 라이브러리를 통해 데이터 과학 및 기계 학습 분야에서 뛰어난 공연을했습니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

MinGW - Windows용 미니멀리스트 GNU
이 프로젝트는 osdn.net/projects/mingw로 마이그레이션되는 중입니다. 계속해서 그곳에서 우리를 팔로우할 수 있습니다. MinGW: GCC(GNU Compiler Collection)의 기본 Windows 포트로, 기본 Windows 애플리케이션을 구축하기 위한 무료 배포 가능 가져오기 라이브러리 및 헤더 파일로 C99 기능을 지원하는 MSVC 런타임에 대한 확장이 포함되어 있습니다. 모든 MinGW 소프트웨어는 64비트 Windows 플랫폼에서 실행될 수 있습니다.
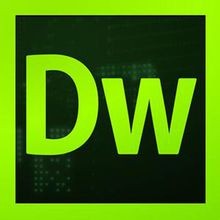
드림위버 CS6
시각적 웹 개발 도구
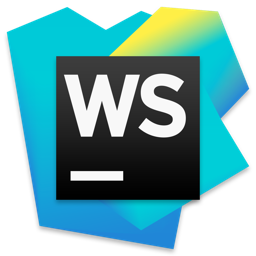
WebStorm Mac 버전
유용한 JavaScript 개발 도구
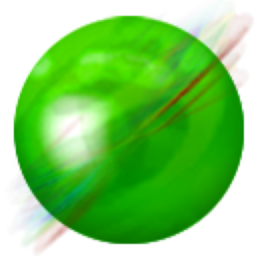
ZendStudio 13.5.1 맥
강력한 PHP 통합 개발 환경

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기
