배경
오늘날의 웹 개발 환경에서 JavaScript는 동적인 대화형 웹 애플리케이션을 만들기 위해 오랫동안 선택되어 왔습니다.
Go 개발자로서 Javascript를 사용하고 싶지 않지만 여전히 반응형 웹 애플리케이션을 구현하고 싶다면 어떻게 해야 할까요?
전체 페이지를 다시 로드하지 않고도 작업을 확인하면 즉시 업데이트되는 멋진 할 일 목록 앱을 상상해 보세요. 이것이 바로 Golang과 htmx의 힘입니다!
Go와 htmx를 결합하면 JavaScript를 한 줄도 작성하지 않고도 반응형 및 대화형 웹 애플리케이션을 만들 수 있습니다.
이 블로그에서는 htmx와 Golang을 사용하여 웹 애플리케이션을 구축하는 방법을 살펴보겠습니다. (다른 즐겨찾는 플랫폼에서도 사용할 수 있습니다.)
학습의 일환으로 사용자를 위한 기본 생성 및 삭제 작업을 구현해 보겠습니다.
htmx란 무엇인가요?
htmx는 브라우저와 서버 간의 양방향 통신을 추가하는 최신 HTML 확장 프로그램입니다.
AJAX, 서버 전송 이벤트 등에 대한 액세스를 HTML로 직접 제공하므로 JavaScript를 작성하지 않고도 동적 웹 페이지를 만들 수 있습니다.
htmx는 어떻게 작동하나요?
- 사용자가 htmx 속성이 있는 요소와 상호작용(예: 버튼 클릭)하면 브라우저가 지정된 이벤트를 트리거합니다.
- htmx는 이벤트를 가로채고 속성에 지정된 서버측 엔드포인트(예: hx-get="/my-endpoint")에 HTTP 요청을 보냅니다.
- 서버측 엔드포인트는 요청을 처리하고 HTML 응답을 생성합니다.
- htmx는 응답을 수신하고 hx-target 및 hx-swap 속성에 따라 DOM을 업데이트합니다. 여기에는 다음이 포함될 수 있습니다.
— 전체 요소의 콘텐츠를 교체합니다.
— 요소 앞이나 뒤에 새 콘텐츠를 삽입합니다.
— 요소 끝에 콘텐츠를 추가합니다.
예문을 통해 좀 더 깊이 이해해 보겠습니다.
<button hx-get="/fetch-data" hx-target="#data-container"> Fetch Data </button> <div> <p>In the above code, when the button is clicked:</p> <ol> <li>htmx sends a GET request to /fetch-data. </li> <li>The server-side endpoint fetches data and renders it as HTML.</li> <li>The response is inserted into the #data-container element.</li> </ol> <h3> Create and delete the user </h3> <p>Below are the required tools/frameworks to build this basic app.</p> <ul> <li>Gin (Go framework)</li> <li>Tailwind CSS </li> <li>htmx</li> </ul> <p><strong>Basic setup</strong> </p> <ul> <li>Create main.go file at the root directory.</li> </ul> <p><strong>main.go</strong><br> </p> <pre class="brush:php;toolbar:false">package main import ( "fmt" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.Run(":8080") fmt.Println("Server is running on port 8080") }
포트 8080에서 실행되는 기본 Go 서버를 설정합니다.
go run main.go를 실행하여 애플리케이션을 실행하세요.
- 사용자 목록을 렌더링하려면 루트 디렉터리에 HTML 파일을 생성하세요.
users.html
<title>Go + htmx app </title> <script src="https://unpkg.com/htmx.org@2.0.0" integrity="sha384-wS5l5IKJBvK6sPTKa2WZ1js3d947pvWXbPJ1OmWfEuxLgeHcEbjUUA5i9V5ZkpCw" crossorigin="anonymous"></script> <script src="https://cdn.tailwindcss.com"></script> <blockquote> <p>We have included,</p> <p><strong>htmx</strong> using the script tag — <u>https://unpkg.com/htmx.org@2.0.0</u></p> <p><strong>Tailwind CSS</strong> with cdn link —<br> <u>https://cdn.tailwindcss.com</u></p> </blockquote> <p>Now, we can use Tailwind CSS classes and render the templates with htmx.</p> <p>As we see in users.html, we need to pass users array to the template, so that it can render the users list. </p> <p>For that let’s create a hardcoded static list of users and create a route to render users.html .</p> <h3> Fetch users </h3> <p><strong>main.go</strong><br> </p> <pre class="brush:php;toolbar:false">package main import ( "fmt" "net/http" "text/template" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/", func(c *gin.Context) { users := GetUsers() tmpl := template.Must(template.ParseFiles("users.html")) err := tmpl.Execute(c.Writer, gin.H{"users": users}) if err != nil { panic(err) } }) router.Run(":8080") fmt.Println("Server is running on port 8080") } type User struct { Name string Email string } func GetUsers() []User { return []User{ {Name: "John Doe", Email: "johndoe@example.com"}, {Name: "Alice Smith", Email: "alicesmith@example.com"}, } }
사용자 목록을 렌더링하고 정적 사용자 목록을 제공하기 위해 / 경로를 추가했습니다(앞으로 새 사용자를 추가할 예정입니다).
그게 다입니다. 서버를 다시 시작하고 — http://localhost:8080/를 방문하여 사용자 목록을 렌더링하는지 확인하세요. 아래와 같이 사용자 목록이 렌더링됩니다.
사용자 생성
user_row.html 파일을 만듭니다. 사용자 테이블에 새 사용자 행을 추가하는 역할을 담당합니다.
user_row.html
<button hx-get="/fetch-data" hx-target="#data-container"> Fetch Data </button> <div> <p>In the above code, when the button is clicked:</p> <ol> <li>htmx sends a GET request to /fetch-data. </li> <li>The server-side endpoint fetches data and renders it as HTML.</li> <li>The response is inserted into the #data-container element.</li> </ol> <h3> Create and delete the user </h3> <p>Below are the required tools/frameworks to build this basic app.</p> <ul> <li>Gin (Go framework)</li> <li>Tailwind CSS </li> <li>htmx</li> </ul> <p><strong>Basic setup</strong> </p> <ul> <li>Create main.go file at the root directory.</li> </ul> <p><strong>main.go</strong><br> </p> <pre class="brush:php;toolbar:false">package main import ( "fmt" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.Run(":8080") fmt.Println("Server is running on port 8080") }
양식 입력에서 이름 및 이메일을 가져와 user_row.html을 실행합니다.
테이블에 새 사용자를 추가해 보겠습니다. http://localhost:8080/을 방문하여 사용자 추가 버튼을 클릭하세요.
야이야! 목록에 새로운 사용자를 성공적으로 추가했습니다 ?.
자세한 구현 가이드를 자세히 알아보려면 Canopas에서 전체 가이드를 확인하세요.
읽은 내용이 마음에 드셨다면 꼭 ? 단추! — 작가로서 그것은 세상을 의미합니다!
아래 댓글 섹션에서 여러분의 생각을 공유해 주시기 바랍니다. 귀하의 의견은 우리의 콘텐츠를 풍성하게 할 뿐만 아니라 귀하를 위해 더 가치 있고 유익한 기사를 작성하려는 동기를 부여합니다.
즐거운 코딩 되셨나요!?
위 내용은 Golang htmx Tailwind CSS: 반응형 웹 애플리케이션 만들기의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
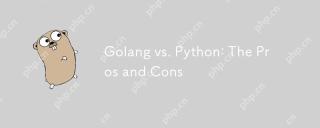
golangisidealforbuildingscalablesystemsdueToitsefficiencyandconcurrency
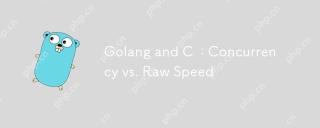
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
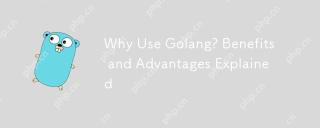
Golang을 선택하는 이유는 다음과 같습니다. 1) 높은 동시성 성능, 2) 정적 유형 시스템, 3) 쓰레기 수집 메커니즘, 4) 풍부한 표준 라이브러리 및 생태계는 효율적이고 신뢰할 수있는 소프트웨어를 개발하기에 이상적인 선택입니다.
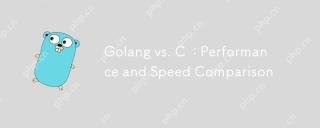
Golang은 빠른 개발 및 동시 시나리오에 적합하며 C는 극도의 성능 및 저수준 제어가 필요한 시나리오에 적합합니다. 1) Golang은 쓰레기 수집 및 동시성 메커니즘을 통해 성능을 향상시키고, 고전성 웹 서비스 개발에 적합합니다. 2) C는 수동 메모리 관리 및 컴파일러 최적화를 통해 궁극적 인 성능을 달성하며 임베디드 시스템 개발에 적합합니다.
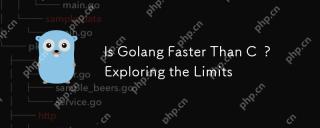
Golang은 컴파일 시간과 동시 처리에서 더 나은 성능을 발휘하는 반면 C는 달리기 속도 및 메모리 관리에서 더 많은 장점을 가지고 있습니다. 1. 골랑은 빠른 컴파일 속도를 가지고 있으며 빠른 개발에 적합합니다. 2.C는 빠르게 실행되며 성능 크리티컬 애플리케이션에 적합합니다. 3. Golang은 동시 처리에 간단하고 효율적이며 동시 프로그래밍에 적합합니다. 4.C 수동 메모리 관리는 더 높은 성능을 제공하지만 개발 복잡성을 증가시킵니다.
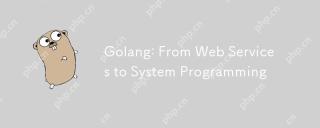
웹 서비스 및 시스템 프로그래밍에서 Golang의 응용 프로그램은 주로 단순성, 효율성 및 동시성에 반영됩니다. 1) 웹 서비스에서 Golang은 강력한 HTTP 라이브러리 및 동시 처리 기능을 통해 고성능 웹 애플리케이션 및 API의 생성을 지원합니다. 2) 시스템 프로그래밍에서 Golang은 운영 체제 개발 및 임베디드 시스템에 적합하기 위해 하드웨어에 가까운 기능 및 C 언어와 호환성을 사용합니다.
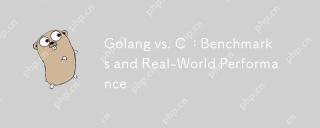
Golang과 C는 성능 비교에서 고유 한 장점과 단점이 있습니다. 1. Golang은 높은 동시성과 빠른 발전에 적합하지만 쓰레기 수집은 성능에 영향을 줄 수 있습니다. 2.C는 더 높은 성능과 하드웨어 제어를 제공하지만 개발 복잡성이 높습니다. 선택할 때는 프로젝트 요구 사항과 팀 기술을 포괄적 인 방식으로 고려해야합니다.
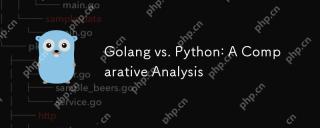
Golang은 고성능 및 동시 프로그래밍 시나리오에 적합하지만 Python은 빠른 개발 및 데이터 처리에 적합합니다. 1. Golang은 단순성과 효율성을 강조하며 백엔드 서비스 및 마이크로 서비스에 적합합니다. 2. Python은 간결한 구문 및 풍부한 라이브러리로 유명하며 데이터 과학 및 기계 학습에 적합합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는

맨티스BT
Mantis는 제품 결함 추적을 돕기 위해 설계된 배포하기 쉬운 웹 기반 결함 추적 도구입니다. PHP, MySQL 및 웹 서버가 필요합니다. 데모 및 호스팅 서비스를 확인해 보세요.

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.
