Python을 사용하여 디스크 리소스 사용량 모니터링 및 서버 상태 업데이트 자동화
최적의 성능을 유지하고 다운타임을 방지하려면 서버 디스크 사용량을 모니터링하는 것이 중요합니다. 이 블로그 게시물에서는 Python 스크립트를 사용하여 디스크 리소스 모니터링을 자동화하고 API를 통해 서버 상태를 업데이트하는 방법을 살펴보겠습니다. 또한 정기적으로 스크립트를 실행하기 위해 cron 작업을 설정하는 방법에 대해서도 논의하겠습니다.
전제 조건
- Python 프로그래밍에 대한 기본 지식
- Linux 명령줄 작업에 대한 지식
- Python 스크립트를 실행하고 크론 작업을 설정할 수 있는 서버에 액세스
- 서버 상태를 업데이트하기 위한 API 엔드포인트(실제 API URL 및 토큰으로 대체)
Python 스크립트 설명
아래는 API를 통해 디스크 리소스 모니터링을 수행하고 서버 상태를 업데이트하는 Python 스크립트입니다.
Health API 생성은 이 블로그 게시물에서 다루지 않습니다. 필요한 경우 댓글을 달아 해당 API 생성 단계도 게시하겠습니다.
import subprocess import requests import argparse class Resource: file_system = '' disk_size = 0.0 used = 0.0 avail = 0.0 use_percent = 0.0 mounted_on = 0.0 disk_free_threshold = 1 mount_partition = "/" class ResourcesMonitor(Resource): def __init__(self): self.__file_system = Resource.file_system self.__disk_size = Resource.disk_size self.__used = Resource.used self.__avail = Resource.avail self.__use_percent = Resource.use_percent self.__mounted_on = Resource.mounted_on self.__disk_free_threshold = Resource.disk_free_threshold self.__mount_partition = Resource.mount_partition def show_resource_usage(self): """ Print the resource usage of disk. """ print("file_system", "disk_size", "used", "avail", "use_percent", "mounted_on") print(self.__file_system, self.__disk_size, self.__used, self.__avail, self.__use_percent, self.__mounted_on) def check_resource_usage(self): """ Check the disk usage by running the Unix 'df -h' command. """ response_df = subprocess.Popen(["df", "-h"], stdout=subprocess.PIPE) for line in response_df.stdout: split_line = line.decode().split() if split_line[5] == self.__mount_partition: if int(split_line[4][:-1]) > self.__disk_free_threshold: self.__file_system, self.__disk_size, self.__used = split_line[0], split_line[1], split_line[2] self.__avail, self.__use_percent, self.__mounted_on = split_line[3], split_line[4], split_line[5] self.show_resource_usage() self.update_resource_usage_api(self) def update_resource_usage_api(self, resource): """ Call the update API using all resource details. """ update_resource_url = url.format( resource.__file_system, resource.__disk_size, resource.__used, resource.__avail, resource.__use_percent, resource_id ) print(update_resource_url) payload = {} files = {} headers = { 'token': 'Bearer APITOKEN' } try: response = requests.request("GET", update_resource_url, headers=headers, data=payload, files=files) if response.ok: print(response.json()) except Exception as ex: print("Error while calling update API") print(ex) if __name__ == '__main__': url = "http://yourapi.com/update_server_health_by_server_id?path={}&size={}" \ "&used={}&avail={}&use_percent={}&id={}" parser = argparse.ArgumentParser(description='Disk Resource Monitor') parser.add_argument('-id', metavar='id', help='ID record of server', default=7, type=int) args = parser.parse_args() resource_id = args.id print(resource_id) resource_monitor = ResourcesMonitor() resource_monitor.check_resource_usage()
리소스 및 ResourcesMonitor 클래스
리소스 클래스는 파일 시스템, 디스크 크기, 사용된 공간 등과 같은 디스크 사용량과 관련된 속성을 정의합니다. ResourcesMonitor 클래스는 Resource에서 상속하고 이러한 속성을 초기화합니다.
디스크 사용량 확인
check_resource_usage 메소드는 Unix df -h 명령을 실행하여 디스크 사용량 통계를 가져옵니다. 출력을 구문 분석하여 지정된 마운트 파티션(기본값은 /)의 디스크 사용량을 찾습니다. 디스크 사용량이 임계값을 초과하면 리소스 세부 정보를 업데이트하고 API 업데이트 메소드를 호출합니다.
API를 통해 서버 상태 업데이트
update_resource_usage_api 메소드는 리소스 세부정보로 API 요청 URL을 구성하고 GET 요청을 보내 서버 상태를 업데이트합니다. http://yourapi.com/update_server_health_by_server_id를 실제 API 엔드포인트로 바꾸고 올바른 API 토큰을 제공하세요.
스크립트 사용
스크립트를 resources_monitor.py로 저장하고 Python 3을 사용하여 실행하세요.
명령줄 인수
- -id: 상태 데이터를 업데이트할 서버 ID(기본값은 7)입니다. 이는 ID만 변경하여 여러 서버에서 동일한 스크립트를 실행하는 데 도움이 됩니다.
예제 사용법 및 출력
$ python3 resource_monitor.py -id=7 Output: file_system disk_size used avail use_percent mounted_on /dev/root 39G 31G 8.1G 80% / API GET Request: http://yourapi.com/update_server_health_by_server_id?path=/dev/root&size=39G&used=31G&avail=8.1G&use_percent=80%&id=7 Response {'success': 'Servers_health data Updated.', 'data': {'id': 7, 'server_id': 1, 'server_name': 'web-server', 'server_ip': '11.11.11.11', 'size': '39G', 'path': '/dev/root', 'used': '31G', 'avail': '8.1G', 'use_percent': '80%', 'created_at': '2021-08-28T13:45:28.000000Z', 'updated_at': '2024-10-27T08:02:43.000000Z'}}
Cron을 사용한 자동화
30분마다 스크립트 실행을 자동화하려면 다음과 같이 cron 작업을 추가하세요.
*/30 * * * * python3 /home/ubuntu/resource_monitor.py -id=7 &
crontab -e를 실행하고 위 줄을 추가하여 cron 작업을 편집할 수 있습니다. 이렇게 하면 스크립트가 30분마다 실행되어 서버 상태 데이터가 최신 상태로 유지됩니다.
결론
디스크 리소스 모니터링 및 서버 상태 업데이트를 자동화하면 서버 성능을 사전에 관리하고 디스크 공간 부족으로 인한 잠재적인 문제를 방지할 수 있습니다. 이 Python 스크립트는 출발점 역할을 하며 특정 요구 사항에 맞게 사용자 정의할 수 있습니다.
위 내용은 Python을 사용하여 디스크 리소스 사용량 모니터링 및 서버 상태 업데이트 자동화의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
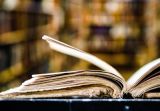
이 튜토리얼은 Python을 사용하여 Zipf의 법칙의 통계 개념을 처리하는 방법을 보여주고 법을 처리 할 때 Python의 읽기 및 대형 텍스트 파일을 정렬하는 효율성을 보여줍니다. ZIPF 분포라는 용어가 무엇을 의미하는지 궁금 할 것입니다. 이 용어를 이해하려면 먼저 Zipf의 법칙을 정의해야합니다. 걱정하지 마세요. 지침을 단순화하려고 노력할 것입니다. Zipf의 법칙 Zipf의 법칙은 단순히 : 큰 자연어 코퍼스에서 가장 자주 발생하는 단어는 두 번째 빈번한 단어, 세 번째 빈번한 단어보다 세 번, 네 번째 빈번한 단어 등 4 배나 자주 발생합니다. 예를 살펴 보겠습니다. 미국 영어로 브라운 코퍼스를 보면 가장 빈번한 단어는 "TH입니다.
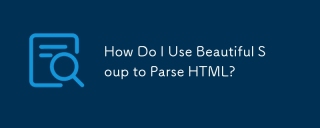
이 기사에서는 HTML을 구문 분석하기 위해 파이썬 라이브러리 인 아름다운 수프를 사용하는 방법을 설명합니다. 데이터 추출, 다양한 HTML 구조 및 오류 처리 및 대안 (SEL과 같은 Find (), find_all (), select () 및 get_text ()와 같은 일반적인 방법을 자세히 설명합니다.
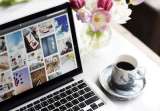
시끄러운 이미지를 다루는 것은 특히 휴대폰 또는 저해상도 카메라 사진에서 일반적인 문제입니다. 이 튜토리얼은 OpenCV를 사용 하여이 문제를 해결하기 위해 Python의 이미지 필터링 기술을 탐구합니다. 이미지 필터링 : 강력한 도구 이미지 필터
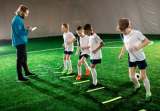
데이터 과학 및 처리가 가장 좋아하는 Python은 고성능 컴퓨팅을위한 풍부한 생태계를 제공합니다. 그러나 Python의 병렬 프로그래밍은 독특한 과제를 제시합니다. 이 튜토리얼은 이러한 과제를 탐구하며 전 세계 해석에 중점을 둡니다.
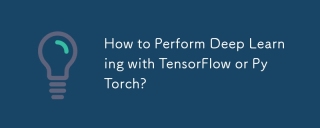
이 기사는 딥 러닝을 위해 텐서 플로와 Pytorch를 비교합니다. 데이터 준비, 모델 구축, 교육, 평가 및 배포와 관련된 단계에 대해 자세히 설명합니다. 프레임 워크, 특히 계산 포도와 관련하여 주요 차이점
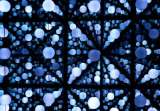
이 튜토리얼은 Python 3에서 사용자 정의 파이프 라인 데이터 구조를 작성하여 클래스 및 작업자 과부하를 활용하여 향상된 기능을 보여줍니다. 파이프 라인의 유연성은 일련의 기능을 데이터 세트, GE에 적용하는 능력에 있습니다.
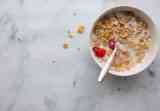
파이썬 객체의 직렬화 및 사막화는 사소한 프로그램의 주요 측면입니다. 무언가를 Python 파일에 저장하면 구성 파일을 읽거나 HTTP 요청에 응답하는 경우 객체 직렬화 및 사태화를 수행합니다. 어떤 의미에서, 직렬화와 사제화는 세계에서 가장 지루한 것들입니다. 이 모든 형식과 프로토콜에 대해 누가 걱정합니까? 일부 파이썬 객체를 지속하거나 스트리밍하여 나중에 완전히 검색하려고합니다. 이것은 세상을 개념적 차원에서 볼 수있는 좋은 방법입니다. 그러나 실제 수준에서 선택한 직렬화 체계, 형식 또는 프로토콜은 속도, 보안, 유지 보수 상태 및 프로그램의 기타 측면을 결정할 수 있습니다.
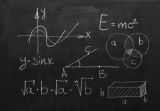
Python의 통계 모듈은 강력한 데이터 통계 분석 기능을 제공하여 생물 통계 및 비즈니스 분석과 같은 데이터의 전반적인 특성을 빠르게 이해할 수 있도록 도와줍니다. 데이터 포인트를 하나씩 보는 대신 평균 또는 분산과 같은 통계를보고 무시할 수있는 원래 데이터에서 트렌드와 기능을 발견하고 대형 데이터 세트를보다 쉽고 효과적으로 비교하십시오. 이 튜토리얼은 평균을 계산하고 데이터 세트의 분산 정도를 측정하는 방법을 설명합니다. 달리 명시되지 않는 한,이 모듈의 모든 함수는 단순히 평균을 합산하는 대신 평균 () 함수의 계산을 지원합니다. 부동 소수점 번호도 사용할 수 있습니다. 무작위로 가져옵니다 수입 통계 Fracti에서


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구
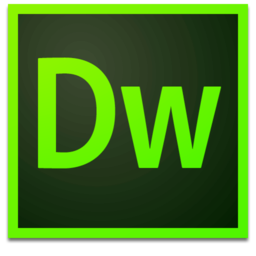
Dreamweaver Mac版
시각적 웹 개발 도구

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.
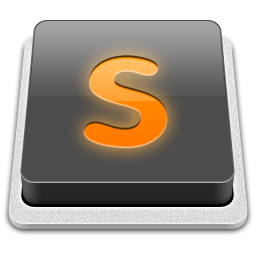
SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

SublimeText3 영어 버전
권장 사항: Win 버전, 코드 프롬프트 지원!

DVWA
DVWA(Damn Vulnerable Web App)는 매우 취약한 PHP/MySQL 웹 애플리케이션입니다. 주요 목표는 보안 전문가가 법적 환경에서 자신의 기술과 도구를 테스트하고, 웹 개발자가 웹 응용 프로그램 보안 프로세스를 더 잘 이해할 수 있도록 돕고, 교사/학생이 교실 환경 웹 응용 프로그램에서 가르치고 배울 수 있도록 돕는 것입니다. 보안. DVWA의 목표는 다양한 난이도의 간단하고 간단한 인터페이스를 통해 가장 일반적인 웹 취약점 중 일부를 연습하는 것입니다. 이 소프트웨어는
