데이터에서 여러 색상의 선 세그먼트 그리기
데이터 포인트를 선으로 시각화하려면 matplotlib를 사용할 수 있습니다. 여기에는 위도와 경도 좌표를 각각 나타내는 'latt'와 'lont'라는 두 개의 목록이 있습니다. 목표는 고유한 색상이 할당된 10개 점의 각 세그먼트를 사용하여 데이터 점을 연결하는 선을 그리는 것입니다.
접근 방법 1: 개별 선 도표
작은 규모의 경우 선 세그먼트 수에 따라 다양한 색상으로 각 세그먼트에 대해 개별 선 플롯을 생성할 수 있습니다. 다음 예제 코드는 이 접근 방식을 보여줍니다.
<code class="python">import numpy as np import matplotlib.pyplot as plt # Assume the list of latitude and longitude is provided # Generate uniqueish colors def uniqueish_color(): return plt.cm.gist_ncar(np.random.random()) # Create a plot fig, ax = plt.subplots() # Iterate through the data in segments of 10 for start, stop in zip(latt[:-1], latt[1:]): # Extract coordinates for each segment x = latt[start:stop] y = lont[start:stop] # Plot each segment with a unique color ax.plot(x, y, color=uniqueish_color()) # Display the plot plt.show()</code>
접근 방식 2: 대규모 데이터 세트를 위한 라인 컬렉션
다양한 라인 세그먼트가 포함된 대규모 데이터 세트의 경우 Line을 사용하여 컬렉션은 효율성을 향상시킬 수 있습니다. 예는 다음과 같습니다.
<code class="python">import numpy as np import matplotlib.pyplot as plt from matplotlib.collections import LineCollection # Prepare the data as a sequence of line segments segments = np.hstack([latt[:-1], latt[1:]]).reshape(-1, 1, 2) # Create a plot fig, ax = plt.subplots() # Create a LineCollection object coll = LineCollection(segments, cmap=plt.cm.gist_ncar) # Assign random colors to the segments coll.set_array(np.random.random(latt.shape[0])) # Add the LineCollection to the plot ax.add_collection(coll) ax.autoscale_view() # Display the plot plt.show()</code>
결론적으로 두 접근 방식 모두 데이터 포인트의 다양한 세그먼트에 대해 다양한 색상으로 선을 효과적으로 그릴 수 있습니다. 선택은 플롯할 선분의 수에 따라 다릅니다.
위 내용은 Python을 사용하여 데이터에서 여러 색상의 선분을 그리는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!
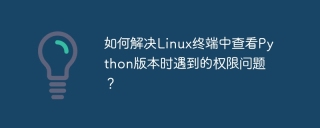
Linux 터미널에서 Python 버전을 보려고 할 때 Linux 터미널에서 Python 버전을 볼 때 권한 문제에 대한 솔루션 ... Python을 입력하십시오 ...
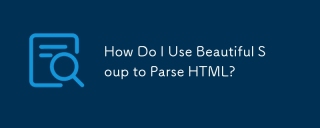
이 기사에서는 HTML을 구문 분석하기 위해 파이썬 라이브러리 인 아름다운 수프를 사용하는 방법을 설명합니다. 데이터 추출, 다양한 HTML 구조 및 오류 처리 및 대안 (SEL과 같은 Find (), find_all (), select () 및 get_text ()와 같은 일반적인 방법을 자세히 설명합니다.
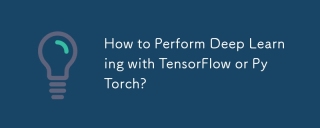
이 기사는 딥 러닝을 위해 텐서 플로와 Pytorch를 비교합니다. 데이터 준비, 모델 구축, 교육, 평가 및 배포와 관련된 단계에 대해 자세히 설명합니다. 프레임 워크, 특히 계산 포도와 관련하여 주요 차이점
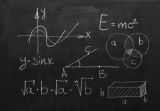
Python의 통계 모듈은 강력한 데이터 통계 분석 기능을 제공하여 생물 통계 및 비즈니스 분석과 같은 데이터의 전반적인 특성을 빠르게 이해할 수 있도록 도와줍니다. 데이터 포인트를 하나씩 보는 대신 평균 또는 분산과 같은 통계를보고 무시할 수있는 원래 데이터에서 트렌드와 기능을 발견하고 대형 데이터 세트를보다 쉽고 효과적으로 비교하십시오. 이 튜토리얼은 평균을 계산하고 데이터 세트의 분산 정도를 측정하는 방법을 설명합니다. 달리 명시되지 않는 한,이 모듈의 모든 함수는 단순히 평균을 합산하는 대신 평균 () 함수의 계산을 지원합니다. 부동 소수점 번호도 사용할 수 있습니다. 무작위로 가져옵니다 수입 통계 Fracti에서
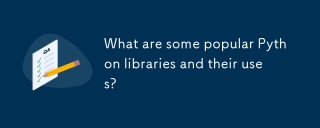
이 기사는 Numpy, Pandas, Matplotlib, Scikit-Learn, Tensorflow, Django, Flask 및 요청과 같은 인기있는 Python 라이브러리에 대해 설명하고 과학 컴퓨팅, 데이터 분석, 시각화, 기계 학습, 웹 개발 및 H에서의 사용에 대해 자세히 설명합니다.
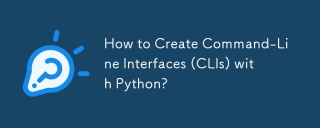
이 기사는 Python 개발자가 CLIS (Command-Line Interfaces) 구축을 안내합니다. Typer, Click 및 Argparse와 같은 라이브러리를 사용하여 입력/출력 처리를 강조하고 CLI 유용성을 향상시키기 위해 사용자 친화적 인 디자인 패턴을 홍보하는 세부 정보.

Python의 Pandas 라이브러리를 사용할 때는 구조가 다른 두 데이터 프레임 사이에서 전체 열을 복사하는 방법이 일반적인 문제입니다. 두 개의 dats가 있다고 가정 해
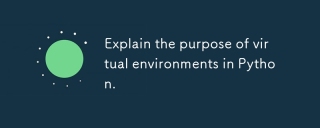
이 기사는 프로젝트 종속성 관리 및 충돌을 피하는 데 중점을 둔 Python에서 가상 환경의 역할에 대해 설명합니다. 프로젝트 관리 개선 및 종속성 문제를 줄이는 데있어 생성, 활성화 및 이점을 자세히 설명합니다.


핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

mPDF
mPDF는 UTF-8로 인코딩된 HTML에서 PDF 파일을 생성할 수 있는 PHP 라이브러리입니다. 원저자인 Ian Back은 자신의 웹 사이트에서 "즉시" PDF 파일을 출력하고 다양한 언어를 처리하기 위해 mPDF를 작성했습니다. HTML2FPDF와 같은 원본 스크립트보다 유니코드 글꼴을 사용할 때 속도가 느리고 더 큰 파일을 생성하지만 CSS 스타일 등을 지원하고 많은 개선 사항이 있습니다. RTL(아랍어, 히브리어), CJK(중국어, 일본어, 한국어)를 포함한 거의 모든 언어를 지원합니다. 중첩된 블록 수준 요소(예: P, DIV)를 지원합니다.

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.
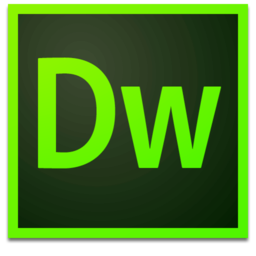
Dreamweaver Mac版
시각적 웹 개발 도구
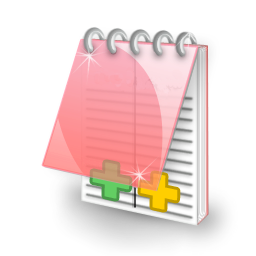
에디트플러스 중국어 크랙 버전
작은 크기, 구문 강조, 코드 프롬프트 기능을 지원하지 않음

안전한 시험 브라우저
안전한 시험 브라우저는 온라인 시험을 안전하게 치르기 위한 보안 브라우저 환경입니다. 이 소프트웨어는 모든 컴퓨터를 안전한 워크스테이션으로 바꿔줍니다. 이는 모든 유틸리티에 대한 액세스를 제어하고 학생들이 승인되지 않은 리소스를 사용하는 것을 방지합니다.
