1. 腐食と拡張
1.1 腐食操作
import cv2 import numpy as np img = cv2.imread('DataPreprocessing/img/dige.png') cv2.imshow("img", img) cv2.waitKey(0) cv2.destroyAllWindows()
dige.png 元の画像 1 表示 (注: 元の画像がない場合は、スクリーンショットを撮って、ローカルに保存します。
1 ラウンドの腐食後~ (反復 = 1)
kernel = np.ones((3, 3), np.uint8) erosion = cv2.erode(img, kernel, iterations=1) cv2.imshow('erosion', erosion) cv2.waitKey(0) cv2.destroyAllWindows()
腐食結果の表示画像 2:
円を複数回腐食する効果と腐食の原理
pie = cv2.imread('DataPreprocessing/img/pie.png') cv2.imshow('pie', pie) cv2.waitKey(0) cv2.destroyAllWindows()
pie.png元画像 3:
図 4:
kernel = np.ones((30, 30), np.uint8) erosion_1 = cv2.erode(pie, kernel, iterations=1) erosion_2 = cv2.erode(pie, kernel, iterations=2) erosion_3 = cv2.erode(pie, kernel, iterations=3) res = np.hstack((erosion_1, erosion_2, erosion_3)) cv2.imshow('res', res) cv2.waitKey(0) cv2.destroyAllWindows()3 つの円形腐食結果の表示
図 5:
kernel = np.ones((3, 3), np.uint8)
dige_dilate = erosion
dige_dilate = cv2.dilate(erosion, kernel, iterations=1)
cv2.imshow('dilate', dige_dilate)
cv2.waitKey(0)
cv2.destroyAllWindows()
拡張前 (図 2)線が太くなり、元の画像とほぼ同じになっていることがわかりましたが、消えていました。あの長いひげのノイズ、図 6:
円を複数回拡張すると、拡張原理が腐食とは逆になり、白ドット フィルタリングを使用すると、フィルタ内のすべてのデータが白になります。
pie = cv2.imread('DataPreprocessing/img/pie.png') kernel = np.ones((30, 30), np.uint8) dilate_1 = cv2.dilate(pie, kernel, iterations=1) dilate_2 = cv2.dilate(pie, kernel, iterations=2) dilate_3 = cv2.dilate(pie, kernel, iterations=3) res = np.hstack((dilate_1, dilate_2, dilate_3)) cv2.imshow('res', res) cv2.waitKey(0) cv2.destroyAllWindows()
拡張の結果3 回円を描きます。図 7:
# 开:先腐蚀,再膨胀
img = cv2.imread('DataPreprocessing/img/dige.png')
kernel = np.ones((5, 5), np.uint8)
opening = cv2.morphologyEx(img, cv2.MORPH_OPEN, kernel)
cv2.imshow('opening', opening)
cv2.waitKey(0)
cv2.destroyAllWindows()
最初に元のピクチャ 1 を腐食し、次にそれを拡張してオープニング操作の結果を取得します。図 8:
# 闭:先膨胀,再腐蚀
img = cv2.imread('DataPreprocessing/img/dige.png')
kernel = np.ones((5, 5), np.uint8)
closing = cv2.morphologyEx(img, cv2.MORPH_CLOSE, kernel)
cv2.imshow('closing', closing)
cv2.waitKey(0)
cv2.destroyAllWindows()
最初に元の画像 1 を展開し、次に腐食して、オープン操作の結果を取得します図 9:
# 梯度=膨胀-腐蚀 pie = cv2.imread('DataPreprocessing/img/pie.png') kernel = np.ones((7, 7), np.uint8) dilate = cv2.dilate(pie, kernel, iterations=5) erosion = cv2.erode(pie, kernel, iterations=5) res = np.hstack((dilate, erosion)) cv2.imshow('res', res) cv2.waitKey(0) cv2.destroyAllWindows() gradient = cv2.morphologyEx(pie, cv2.MORPH_GRADIENT, kernel) cv2.imshow('gradient', gradient) cv2.waitKey(0) cv2.destroyAllWindows()勾配演算結果を取得します
図 10:
##4. シルクハットとブラックハット
4.1 シルクハット
#シルクハット=元の入力オープン操作の結果# 礼帽
img = cv2.imread('DataPreprocessing/img/dige.png')
tophat = cv2.morphologyEx(img, cv2.MORPH_TOPHAT, kernel)
cv2.imshow('tophat', tophat)
cv2.waitKey(0)
cv2.destroyAllWindows()
トップハット結果の取得
4.2 Black Hat
# 黑帽
img = cv2.imread('DataPreprocessing/img/dige.png')
blackhat = cv2.morphologyEx(img, cv2.MORPH_BLACKHAT, kernel)
cv2.imshow('blackhat ', blackhat)
cv2.waitKey(0)
cv2.destroyAllWindows()
トップハット結果の取得
以上がPython+OpenCVでのモルフォロジーの演算方法とはの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
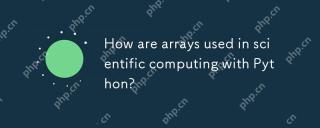
Arraysinpython、特にvianumpy、arecrucialinscientificComputing fortheirefficienty andversitility.1)彼らは、fornumericaloperations、data analysis、andmachinelearning.2)numpy'simplementation incensuresfasteroperationsthanpasteroperations.3)arayableminablecickick
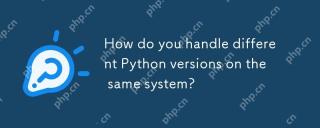
Pyenv、Venv、およびAnacondaを使用して、さまざまなPythonバージョンを管理できます。 1)Pyenvを使用して、複数のPythonバージョンを管理します。Pyenvをインストールし、グローバルバージョンとローカルバージョンを設定します。 2)VENVを使用して仮想環境を作成して、プロジェクトの依存関係を分離します。 3)Anacondaを使用して、データサイエンスプロジェクトでPythonバージョンを管理します。 4)システムレベルのタスク用にシステムPythonを保持します。これらのツールと戦略を通じて、Pythonのさまざまなバージョンを効果的に管理して、プロジェクトのスムーズな実行を確保できます。
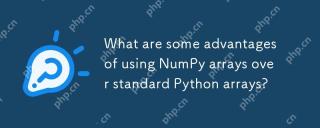
numpyarrayshaveveraladvantages-averstandardpythonarrays:1)thealmuchfasterduetocベースのインプレンテーション、2)アレモレメモリ効率、特にlargedatasets、および3)それらは、拡散化された、構造化された形成術科療法、
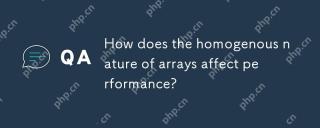
パフォーマンスに対する配列の均一性の影響は二重です。1)均一性により、コンパイラはメモリアクセスを最適化し、パフォーマンスを改善できます。 2)しかし、タイプの多様性を制限し、それが非効率につながる可能性があります。要するに、適切なデータ構造を選択することが重要です。
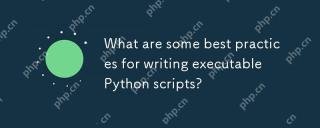
craftexecutablepythonscripts、次のようになります
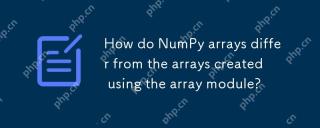
numpyarraysarasarebetterfornumeroperations andmulti-dimensionaldata、whilethearraymoduleissuitable forbasic、1)numpyexcelsinperformance and forlargedatasentassandcomplexoperations.2)thearraymuremememory-effictientivearientfa
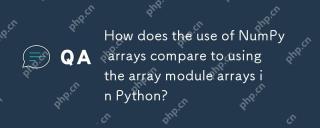
NumPyArraySareBetterforHeavyNumericalComputing、whilethearrayarayismoreSuitableformemory-constrainedprojectswithsimpledatatypes.1)numpyarraysofferarays andatiledance andpeperancedatasandatassandcomplexoperations.2)thearraymoduleisuleiseightweightandmemememe-ef
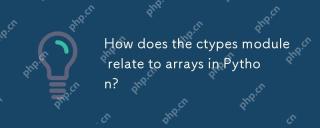
ctypesallowsinging andmanipulatingc-stylearraysinpython.1)usectypestointerfacewithclibrariesforperformance.2)createc-stylearraysfornumericalcomputations.3)passarraystocfunctions foreffientientoperations.how、how、becuutiousmorymanagemation、performanceo


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

SAP NetWeaver Server Adapter for Eclipse
Eclipse を SAP NetWeaver アプリケーション サーバーと統合します。

DVWA
Damn Vulnerable Web App (DVWA) は、非常に脆弱な PHP/MySQL Web アプリケーションです。その主な目的は、セキュリティ専門家が法的環境でスキルとツールをテストするのに役立ち、Web 開発者が Web アプリケーションを保護するプロセスをより深く理解できるようにし、教師/生徒が教室環境で Web アプリケーションを教え/学習できるようにすることです。安全。 DVWA の目標は、シンプルでわかりやすいインターフェイスを通じて、さまざまな難易度で最も一般的な Web 脆弱性のいくつかを実践することです。このソフトウェアは、
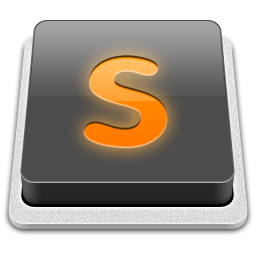
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

メモ帳++7.3.1
使いやすく無料のコードエディター
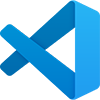
VSCode Windows 64 ビットのダウンロード
Microsoft によって発売された無料で強力な IDE エディター

ホットトピック









