この記事では、PHP に関する関連知識を紹介します。主に PHP アダプター モードとコード例について説明します。興味のある方は以下を参照してください。お役に立てれば幸いです。
PHP アダプター パターンの説明とコード例
Adapter は構造設計パターンです。これにより、互換性のないオブジェクトが相互に連携できるようになります。
アダプターは 2 つのオブジェクト間のラッパーとして機能し、一方のオブジェクトへの呼び出しを受け取り、もう一方のオブジェクトが認識する形式とインターフェイスに変換します。
複雑さ: ******
人気度: ******
使用法例: アダプター パターンは、PHP コードで非常に一般的です。一部のレガシー コードに基づくシステムでは、このパターンがよく使用されます。この場合、アダプターを使用すると、レガシー コードが最新のクラスで動作できるようになります。
識別方法: アダプターは、さまざまな抽象型またはインターフェイス型のインスタンスをパラメーターとして受け取るコンストラクターを通じて識別できます。アダプターのメソッドが呼び出されると、パラメーターが適切な形式に変換され、カプセル化されたオブジェクト内の 1 つ以上のメソッドに呼び出しが指示されます。
実際の例
アダプターを使用すると、コードと互換性がない場合でも、サードパーティまたはレガシー システムのクラスを使用できます。たとえば、各サードパーティ サービスをサポートするためにアプリケーションの通知インターフェイスを書き直すことなく、アプリケーションによる呼び出しをサードパーティ クラスで必要なインターフェイスと形式に適合させる一連の特別なラッパーを作成できます ( DingTalk、WeChat、SMS、またはその他のサービス)。
index.php: 実際の例
<?php namespace RefactoringGuru\Adapter\RealWorld; /** * The Target interface represents the interface that your application's classes * already follow. */ interface Notification { public function send(string $title, string $message); } /** * Here's an example of the existing class that follows the Target interface. * * The truth is that many real apps may not have this interface clearly defined. * If you're in that boat, your best bet would be to extend the Adapter from one * of your application's existing classes. If that's awkward (for instance, * SlackNotification doesn't feel like a subclass of EmailNotification), then * extracting an interface should be your first step. */ class EmailNotification implements Notification { private $adminEmail; public function __construct(string $adminEmail) { $this->adminEmail = $adminEmail; } public function send(string $title, string $message): void { mail($this->adminEmail, $title, $message); echo "Sent email with title '$title' to '{$this->adminEmail}' that says '$message'."; } } /** * The Adaptee is some useful class, incompatible with the Target interface. You * can't just go in and change the code of the class to follow the Target * interface, since the code might be provided by a 3rd-party library. */ class SlackApi { private $login; private $apiKey; public function __construct(string $login, string $apiKey) { $this->login = $login; $this->apiKey = $apiKey; } public function logIn(): void { // Send authentication request to Slack web service. echo "Logged in to a slack account '{$this->login}'.\n"; } public function sendMessage(string $chatId, string $message): void { // Send message post request to Slack web service. echo "Posted following message into the '$chatId' chat: '$message'.\n"; } } /** * The Adapter is a class that links the Target interface and the Adaptee class. * In this case, it allows the application to send notifications using Slack * API. */ class SlackNotification implements Notification { private $slack; private $chatId; public function __construct(SlackApi $slack, string $chatId) { $this->slack = $slack; $this->chatId = $chatId; } /** * An Adapter is not only capable of adapting interfaces, but it can also * convert incoming data to the format required by the Adaptee. */ public function send(string $title, string $message): void { $slackMessage = "#" . $title . "# " . strip_tags($message); $this->slack->logIn(); $this->slack->sendMessage($this->chatId, $slackMessage); } } /** * The client code can work with any class that follows the Target interface. */ function clientCode(Notification $notification) { // ... echo $notification->send("Website is down!", "<strong style='color:red;font-size: 50px;'>Alert!</strong> " . "Our website is not responding. Call admins and bring it up!"); // ... } echo "Client code is designed correctly and works with email notifications:\n"; $notification = new EmailNotification("developers@example.com"); clientCode($notification); echo "\n\n"; echo "The same client code can work with other classes via adapter:\n"; $slackApi = new SlackApi("example.com", "XXXXXXXX"); $notification = new SlackNotification($slackApi, "Example.com Developers"); clientCode($notification);
Output.txt: 実行結果
Client code is designed correctly and works with email notifications: Sent email with title 'Website is down!' to 'developers@example.com' that says '<strong style='color:red;font-size: 50px;'>Alert!</strong> Our website is not responding. Call admins and bring it up!'. The same client code can work with other classes via adapter: Logged in to a slack account 'example.com'. Posted following message into the 'Example.com Developers' chat: '#Website is down!# Alert! Our website is not responding. Call admins and bring it up!'.
概念的な例
この例は、Adapter 設計パターンの構造を示しており、次の質問に答えることに重点を置いています。
- これはどのようなクラスで構成されていますか?
- これらのクラスはどのような役割を果たしますか?
- パターン内の要素は互いにどのように関係しているのでしょうか?
このパターンの構造を理解すると、次の実際の PHP アプリケーションのケースをより簡単に理解できるようになります。
index.php: 概念例
<?php namespace RefactoringGuru\Adapter\Conceptual; /** * The Target defines the domain-specific interface used by the client code. */ class Target { public function request(): string { return "Target: The default target's behavior."; } } /** * The Adaptee contains some useful behavior, but its interface is incompatible * with the existing client code. The Adaptee needs some adaptation before the * client code can use it. */ class Adaptee { public function specificRequest(): string { return ".eetpadA eht fo roivaheb laicepS"; } } /** * The Adapter makes the Adaptee's interface compatible with the Target's * interface. */ class Adapter extends Target { private $adaptee; public function __construct(Adaptee $adaptee) { $this->adaptee = $adaptee; } public function request(): string { return "Adapter: (TRANSLATED) " . strrev($this->adaptee->specificRequest()); } } /** * The client code supports all classes that follow the Target interface. */ function clientCode(Target $target) { echo $target->request(); } echo "Client: I can work just fine with the Target objects:\n"; $target = new Target(); clientCode($target); echo "\n\n"; $adaptee = new Adaptee(); echo "Client: The Adaptee class has a weird interface. See, I don't understand it:\n"; echo "Adaptee: " . $adaptee->specificRequest(); echo "\n\n"; echo "Client: But I can work with it via the Adapter:\n"; $adapter = new Adapter($adaptee); clientCode($adapter);
Output.txt: 実行結果
Client: I can work just fine with the Target objects: Target: The default target's behavior. Client: The Adaptee class has a weird interface. See, I don't understand it: Adaptee: .eetpadA eht fo roivaheb laicepS Client: But I can work with it via the Adapter: Adapter: (TRANSLATED) Special behavior of the Adaptee.
推奨調査:《PHP ビデオ チュートリアル>>
以上がPHP アダプター パターンの詳細な説明 (コード例付き)の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
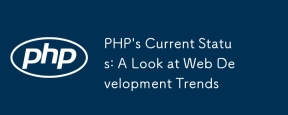
PHPは、現代のWeb開発、特にコンテンツ管理とeコマースプラットフォームで依然として重要です。 1)PHPには、LaravelやSymfonyなどの豊富なエコシステムと強力なフレームワークサポートがあります。 2)パフォーマンスの最適化は、Opcacheとnginxを通じて達成できます。 3)PHP8.0は、パフォーマンスを改善するためにJITコンパイラを導入します。 4)クラウドネイティブアプリケーションは、DockerおよびKubernetesを介して展開され、柔軟性とスケーラビリティを向上させます。
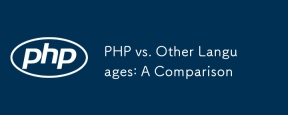
PHPは、特に迅速な開発や動的なコンテンツの処理に適していますが、データサイエンスとエンタープライズレベルのアプリケーションには良くありません。 Pythonと比較して、PHPはWeb開発においてより多くの利点がありますが、データサイエンスの分野ではPythonほど良くありません。 Javaと比較して、PHPはエンタープライズレベルのアプリケーションでより悪化しますが、Web開発により柔軟性があります。 JavaScriptと比較して、PHPはバックエンド開発により簡潔ですが、フロントエンド開発のJavaScriptほど良くありません。
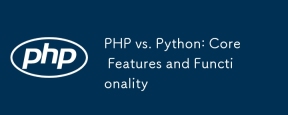
PHPとPythonにはそれぞれ独自の利点があり、さまざまなシナリオに適しています。 1.PHPはWeb開発に適しており、組み込みのWebサーバーとRich Functionライブラリを提供します。 2。Pythonは、簡潔な構文と強力な標準ライブラリを備えたデータサイエンスと機械学習に適しています。選択するときは、プロジェクトの要件に基づいて決定する必要があります。
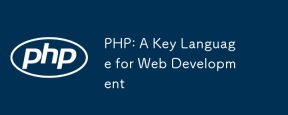
PHPは、サーバー側で広く使用されているスクリプト言語で、特にWeb開発に適しています。 1.PHPは、HTMLを埋め込み、HTTP要求と応答を処理し、さまざまなデータベースをサポートできます。 2.PHPは、ダイナミックWebコンテンツ、プロセスフォームデータ、アクセスデータベースなどを生成するために使用され、強力なコミュニティサポートとオープンソースリソースを備えています。 3。PHPは解釈された言語であり、実行プロセスには語彙分析、文法分析、編集、実行が含まれます。 4.PHPは、ユーザー登録システムなどの高度なアプリケーションについてMySQLと組み合わせることができます。 5。PHPをデバッグするときは、error_reporting()やvar_dump()などの関数を使用できます。 6. PHPコードを最適化して、キャッシュメカニズムを使用し、データベースクエリを最適化し、組み込み関数を使用します。 7
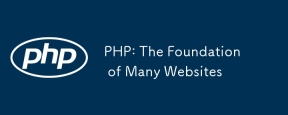
PHPが多くのWebサイトよりも優先テクノロジースタックである理由には、その使いやすさ、強力なコミュニティサポート、広範な使用が含まれます。 1)初心者に適した学習と使用が簡単です。 2)巨大な開発者コミュニティと豊富なリソースを持っています。 3)WordPress、Drupal、その他のプラットフォームで広く使用されています。 4)Webサーバーとしっかりと統合して、開発の展開を簡素化します。
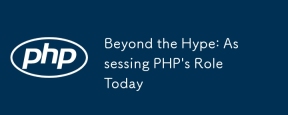
PHPは、特にWeb開発の分野で、最新のプログラミングで強力で広く使用されているツールのままです。 1)PHPは使いやすく、データベースとシームレスに統合されており、多くの開発者にとって最初の選択肢です。 2)動的コンテンツ生成とオブジェクト指向プログラミングをサポートし、Webサイトを迅速に作成および保守するのに適しています。 3)PHPのパフォーマンスは、データベースクエリをキャッシュおよび最適化することで改善でき、その広範なコミュニティと豊富なエコシステムにより、今日のテクノロジースタックでは依然として重要になります。
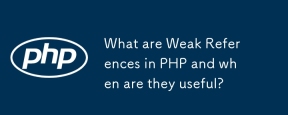
PHPでは、弱い参照クラスを通じて弱い参照が実装され、ガベージコレクターがオブジェクトの回収を妨げません。弱い参照は、キャッシュシステムやイベントリスナーなどのシナリオに適しています。オブジェクトの生存を保証することはできず、ごみ収集が遅れる可能性があることに注意する必要があります。
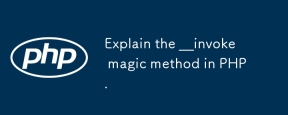
\ _ \ _ Invokeメソッドを使用すると、オブジェクトを関数のように呼び出すことができます。 1。オブジェクトを呼び出すことができるように\ _ \ _呼び出しメソッドを定義します。 2。$ obj(...)構文を使用すると、PHPは\ _ \ _ Invokeメソッドを実行します。 3。ロギングや計算機、コードの柔軟性の向上、読みやすさなどのシナリオに適しています。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
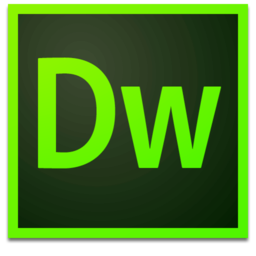
Dreamweaver Mac版
ビジュアル Web 開発ツール
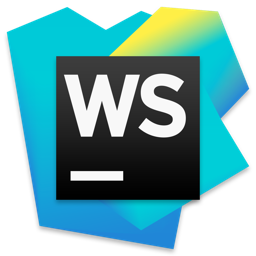
WebStorm Mac版
便利なJavaScript開発ツール
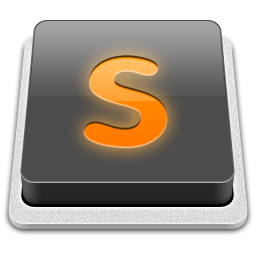
SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

mPDF
mPDF は、UTF-8 でエンコードされた HTML から PDF ファイルを生成できる PHP ライブラリです。オリジナルの作者である Ian Back は、Web サイトから「オンザフライ」で PDF ファイルを出力し、さまざまな言語を処理するために mPDF を作成しました。 HTML2FPDF などのオリジナルのスクリプトよりも遅く、Unicode フォントを使用すると生成されるファイルが大きくなりますが、CSS スタイルなどをサポートし、多くの機能強化が施されています。 RTL (アラビア語とヘブライ語) や CJK (中国語、日本語、韓国語) を含むほぼすべての言語をサポートします。ネストされたブロックレベル要素 (P、DIV など) をサポートします。
