Boost Asio での async_write 呼び出しのインターリーブを防ぐ方法
分散システムでは、クライアントがサーバーにメッセージを非同期に送信するのが一般的です。受信メッセージを処理するために、サーバーは通常、メッセージが受信順に順次処理されるキューベースのメカニズムを実装します。ただし、特定のシナリオでは、メッセージがインターリーブされ、予期しない動作が発生する可能性があります。
問題の説明
複数のクライアントからメッセージを同時に受信するサーバーが関係するシナリオを考えてみましょう。各クライアントのメッセージは、async_write を使用して非同期的に処理されます。クライアントが速いペースでメッセージを送信する場合、async_write 呼び出しがインターリーブされ、メッセージが順番どおりに処理されない可能性があります。
解決策: キューベースのアプローチ
これを防ぐにはasync_write 呼び出しのインターリーブでは、キューベースのアプローチを採用できます。その仕組みは次のとおりです。
- 各クライアントには専用の送信メッセージ キューがあります。
- クライアントがメッセージを送信すると、メッセージは送信キューに追加されます。
- サーバーは各クライアントの送信キューのサイズをチェックします。
- キューが空でない場合、サーバーはキューを開始します。 async_write オペレーションは、キュー内の最初のメッセージを送信します。
- async_write オペレーションが完了すると、サーバーはキューを再度チェックします。
- キュー内にさらにメッセージがある場合は、別の async_write オペレーションが実行されます。
- このプロセスは、キュー内のすべてのメッセージが送信されるまで繰り返されます。
実装例
次のコード スニペットは、このキューベースのアプローチを実装する方法を示しています。
// Include necessary headers #include <boost> #include <boost> #include <deque> #include <iostream> #include <string> class Connection { public: Connection( boost::asio::io_service& io_service ) : _io_service( io_service ), _strand( _io_service ), _socket( _io_service ), _outbox() { } void write( const std::string& message ) { _strand.post( boost::bind( &Connection::writeImpl, this, message ) ); } private: void writeImpl( const std::string& message ) { _outbox.push_back( message ); if ( _outbox.size() > 1 ) { // outstanding async_write return; } this->write(); } void write() { const std::string& message = _outbox[0]; boost::asio::async_write( _socket, boost::asio::buffer( message.c_str(), message.size() ), _strand.wrap( boost::bind( &Connection::writeHandler, this, boost::asio::placeholders::error, boost::asio::placeholders::bytes_transferred ) ) ); } void writeHandler( const boost::system::error_code& error, const size_t bytesTransferred ) { _outbox.pop_front(); if ( error ) { std::cerr write(); } } private: typedef std::deque<:string> Outbox; private: boost::asio::io_service& _io_service; boost::asio::io_service::strand _strand; boost::asio::ip::tcp::socket _socket; Outbox _outbox; }; int main() { boost::asio::io_service io_service; Connection foo( io_service ); }</:string></string></iostream></deque></boost></boost>
結論
キューベースのアプローチを実装すると、async_write 呼び出しのインターリーブを効果的に防止でき、メッセージが正しい順序で処理されることが保証されます。これは、メッセージ処理の順序がシステムの全体的な機能に大きな影響を与えるシナリオでは特に重要です。
以上がBoost Asio でインターリーブされた `async_write` 呼び出しを防ぐ方法は?の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。
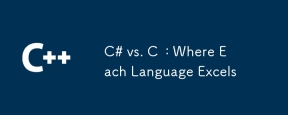
C#は、開発効率とクロスプラットフォームのサポートを必要とするプロジェクトに適していますが、Cは高性能で基礎となるコントロールを必要とするアプリケーションに適しています。 1)C#は、開発を簡素化し、ガベージコレクションとリッチクラスライブラリを提供します。これは、エンタープライズレベルのアプリケーションに適しています。 2)Cは、ゲーム開発と高性能コンピューティングに適した直接メモリ操作を許可します。
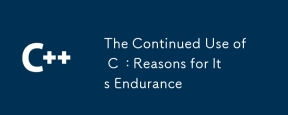
C継続的な使用の理由には、その高性能、幅広いアプリケーション、および進化する特性が含まれます。 1)高効率パフォーマンス:Cは、メモリとハードウェアを直接操作することにより、システムプログラミングと高性能コンピューティングで優れたパフォーマンスを発揮します。 2)広く使用されている:ゲーム開発、組み込みシステムなどの分野での輝き。3)連続進化:1983年のリリース以来、Cは競争力を維持するために新しい機能を追加し続けています。
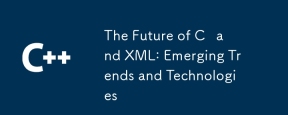
CとXMLの将来の開発動向は次のとおりです。1)Cは、プログラミングの効率とセキュリティを改善するためのC 20およびC 23の標準を通じて、モジュール、概念、CORoutinesなどの新しい機能を導入します。 2)XMLは、データ交換および構成ファイルの重要なポジションを引き続き占有しますが、JSONとYAMLの課題に直面し、XMLSchema1.1やXpath3.1の改善など、より簡潔で簡単な方向に発展します。
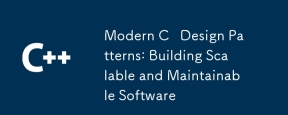
最新のCデザインモデルは、C 11以降の新機能を使用して、より柔軟で効率的なソフトウェアを構築するのに役立ちます。 1)ラムダ式とstd :: functionを使用して、オブザーバーパターンを簡素化します。 2)モバイルセマンティクスと完全な転送を通じてパフォーマンスを最適化します。 3)インテリジェントなポインターは、タイプの安全性とリソース管理を保証します。
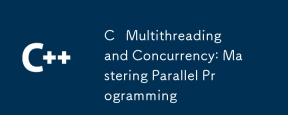
cマルチスレッドと同時プログラミングのコア概念には、スレッドの作成と管理、同期と相互排除、条件付き変数、スレッドプーリング、非同期プログラミング、一般的なエラーとデバッグ技術、パフォーマンスの最適化とベストプラクティスが含まれます。 1)STD ::スレッドクラスを使用してスレッドを作成します。この例は、スレッドが完了する方法を作成し、待つ方法を示しています。 2)共有リソースを保護し、データ競争を回避するために、STD :: MutexおよびSTD :: LOCK_GUARDを使用するための同期と相互除外。 3)条件変数は、std :: condition_variableを介したスレッド間の通信と同期を実現します。 4)スレッドプールの例は、スレッドプールクラスを使用してタスクを並行して処理して効率を向上させる方法を示しています。 5)非同期プログラミングはSTD :: ASを使用します
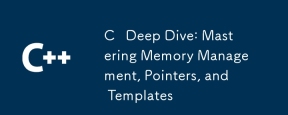
Cのメモリ管理、ポインター、テンプレートはコア機能です。 1。メモリ管理は、新規および削除を通じてメモリを手動で割り当ててリリースし、ヒープとスタックの違いに注意を払います。 2。ポインターにより、メモリアドレスを直接操作し、注意して使用します。スマートポインターは管理を簡素化できます。 3.テンプレートは、一般的なプログラミングを実装し、コードの再利用性と柔軟性を向上させ、タイプの派生と専門化を理解する必要があります。
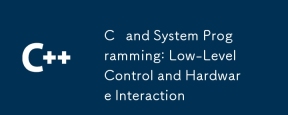
Cは、ハードウェアに近い制御機能とオブジェクト指向プログラミングの強力な機能を提供するため、システムプログラミングとハードウェアの相互作用に適しています。 1)cポインター、メモリ管理、ビット操作などの低レベルの機能、効率的なシステムレベル操作を実現できます。 2)ハードウェアの相互作用はデバイスドライバーを介して実装され、Cはこれらのドライバーを書き込み、ハードウェアデバイスとの通信を処理できます。
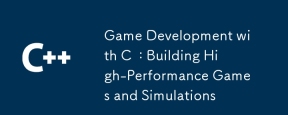
Cは、ハードウェア制御と効率的なパフォーマンスに近いため、高性能のゲームおよびシミュレーションシステムの構築に適しています。 1)メモリ管理:手動制御により、断片化が減少し、パフォーマンスが向上します。 2)コンパイル時間の最適化:インライン関数とループ拡張は、ランニング速度を改善します。 3)低レベルの操作:ハードウェアへの直接アクセス、グラフィックスおよび物理コンピューティングの最適化。


ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境
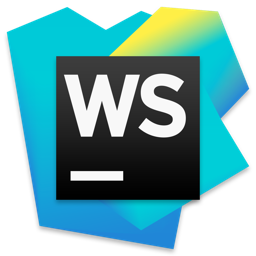
WebStorm Mac版
便利なJavaScript開発ツール

MantisBT
Mantis は、製品の欠陥追跡を支援するために設計された、導入が簡単な Web ベースの欠陥追跡ツールです。 PHP、MySQL、Web サーバーが必要です。デモおよびホスティング サービスをチェックしてください。

SublimeText3 Linux 新バージョン
SublimeText3 Linux 最新バージョン

メモ帳++7.3.1
使いやすく無料のコードエディター
