Detailed explanation of jQuery's observer pattern_jquery
In jQuery, the on method can bind events to elements, and the trigger method can manually trigger events. Focusing on these two methods, let's experience the Observer Pattern in jQuery.
■ The on method binds built-in events and triggers them naturally
For example, if we bind a click event to the body element of the page, write like this.
hello
■ The on method binds built-in events and triggers them manually
Using the trigger method, you can also manually trigger the built-in event bound to the element.
In the above, there is no need to click the body. After the page is loaded, the body automatically triggers the click event.
We know that click is a built-in event of jquery. So, can the event be customized and triggered manually?
Above, we customized a someclick event, and the result is the same as above.
So, we found that we can bind a custom event to the element and trigger the event using the trigger method.
Of course, the name of the custom event can be written in the form of "namespace.custom event name", such as app.someclick. This is especially useful in large projects, which can effectively avoid custom event name conflicts.
From the perspective of "publish and subscribe", the on method is equivalent to a subscriber and an observer, and the trigger method is equivalent to a publisher.
■ Experience the jQuery observer mode from "Get json data asynchronously"
In the root directory, there is a data.json file.
{
"one" : "Hello",
"two" : "World"
}
Now, get the json data asynchronously.
This time, the result we got was undefined. Why is this?
How to solve this problem?
Above, the on method is like a subscriber, which subscribes to the custom event app.myevent; and the trigger method is like a publisher, which publishes events and parameters before the subscriber method is actually executed.
■ Extension methods of jQuery observer pattern
For this purpose, we can also write an extension method specifically for the jQuery observer pattern.
The above defines the global publish and subscribe methods, which we can call at any time.
Summary: jQuery's observer mode actually allows the custom event bound by the on method to not be executed until the trigger method is used to trigger the event. The advantage of using jQuery's observer pattern is: publish once and subscribe multiple times.
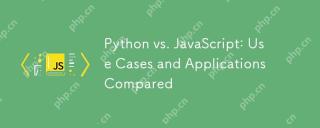
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
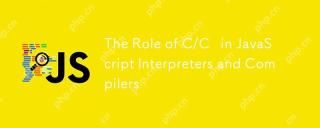
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
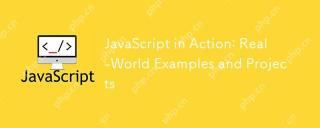
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
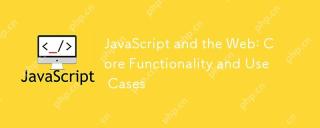
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
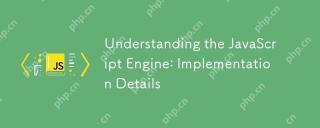
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
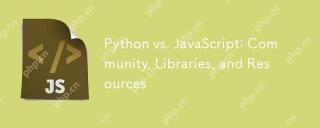
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
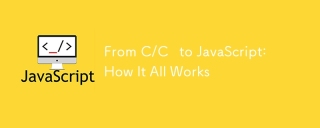
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
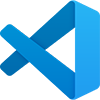
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software