


JavaScript Design Pattern Learning 'Class Inheritance'_javascript skills
Before doing something, you must first understand the benefits of doing it. I believe no one is willing to do something for no reason. Generally speaking, when we design a class, we actually hope to reduce repetitive code. Using inheritance can do this perfectly. With the inheritance mechanism, you can design again based on the existing class and fully Modifications to the design are made easier by taking advantage of the methods they already have. Without further ado, here are some examples:
function Person(name){
This.name = name;
}
Person.prototype.getname = function(){
Return this.name;
}
function Bloger(name,blog){
Person.call(this,name);
This.blog = blog;
}
var blogger = new Bloger("zhenn","http://www.jb51.net");
alert(bloger.name=="zhenn"); /*return true*/
alert(bloger.blog) /*Alert http://www.jb51.net*/
alert(bloger.getname()=="zhenn"); /*Prompt "bloger.getname is not a function"*/
As you can see from the above example, Blogger dynamically calls the native properties and methods of its parent class Person through call internally (for an explanation of call, please refer to http://www.jb51.net/article/62086.htm ), which can be understood as Bloger inherits Person and becomes a subclass of it, but careful students will find that the methods in the Person prototype object cannot be inherited simply by relying on call, which means "bloger. getname is not a function" is the reason. But don’t worry, you can solve this problem with a little processing of the above code!
function Person(name){
This.name = name;
}
Person.prototype.getname = function(){
Return this.name;
}
function Bloger(name,blog){
Person.call(this,name);
This.blog = blog;
}
/*Please pay attention to the following two lines of code*/
Bloger.prototype = new Person();
Bloger.prototype.constructor = Bloger;
var blogger = new Bloger("zhenn","http://www.jb51.net");
alert(bloger.name=="zhenn"); /*return true*/
alert(bloger.blog) /*Alert http://www.jb51.net*/
alert(bloger.getname()=="zhenn"); /*Prompt true*/
Here we need to explain these two lines of code. We know that each constructor has a prototype attribute, which points to the prototype object of the constructor. In fact, the prototype object is also an instance object, but in the prototype object The defined properties and methods can be shared by all instance objects. It can be concluded that the purpose of adding two lines of code is to set the prototype object of the subclass to point to an instantiated object of the parent class, and the instantiated object of the parent class All the prototype attribute methods of the parent class will be inherited, thus achieving our goal. The prototype of the subclass inherits all the properties and methods of the parent class instance object.
But you should also pay attention to the line of code Bloger.prototype.constructor = Bloger; because when you set the prototype of the subclass to an instance of the parent class, its constructor attribute will point to the parent class, so you need to set the constructor of the subclass prototype again. Point to the subclass. At this point, JavaScript class inheritance has been perfectly implemented!
In order to simplify the declaration of subclasses, the entire process of extending a subclass can be written in a function called extend. The function is to create a new class based on a given class structure:
function extend(childClass,parentClass){
var F = new Function();
F.prototype = parentClass.prototype;
childClass.prototype = new F();
childClass.prototype.constructor = childClass;
}
With this extend function, you can easily extend subclasses. Just call this function. The two lines of code added above can be changed to extend(Bloger,Person), and full inheritance can also be achieved!
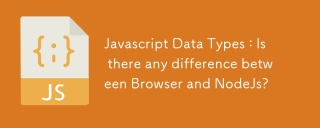
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
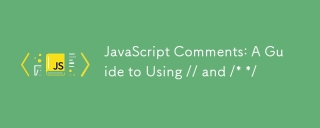
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
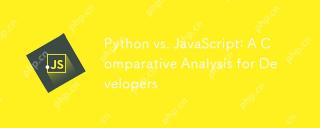
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
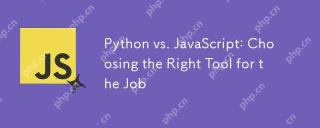
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
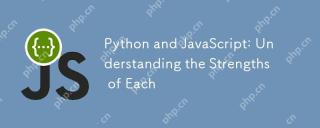
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
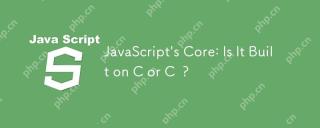
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
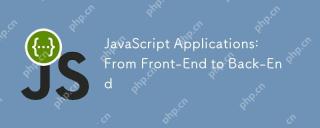
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
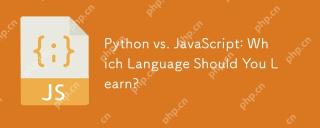
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
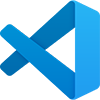
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
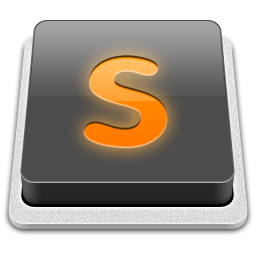
SublimeText3 Mac version
God-level code editing software (SublimeText3)
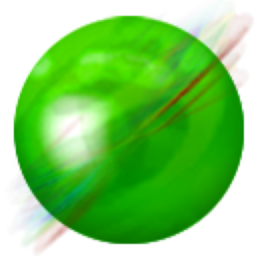
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
