


JavaScript core data types are consistent in browsers and Node.js, but they are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
When diving into JavaScript, understanding data types is fundamental, but it's equally important to grap how these types behave across different environments like browsers and Node.js. Let's explore this fascinating topic, sharing insights and experiences along the way.
In JavaScript, the core data types are consistent across environments: Number
, String
, Boolean
, Undefined
, Null
, Object
, and Symbol
(introduced in ES6). However, the way these types are handled or the additional types available can different slightly between browsers and Node.js, which can lead to some interesting nuances and potential pitfalls.
Let's start with a simple yet revealing example. In both environments, you can declare a variable and assign it a value:
let myNumber = 42; console.log(typeof myNumber); // Output: "number"
This code snippet works independently in both a browser and Node.js, showing that the basic data types are indeed the same. But let's dig deeper.
One of the key differences lies in the global
object. In a browser, the global object is window
, whereas in Node.js, it's global
. This difference can affect how you access global variables or functions:
// In a browser console.log(window); // Outputs the window object // In Node.js console.log(global); // Outputs the global object
This distinction is cruel when writing cross-platform JavaScript. If you're developing a library or application that needs to run in both environments, you'll need to handle these differences gracefully. A common approach is to use a conditional check:
const globalThis = (() => { if (typeof window !== 'undefined') { return window; } else if (typeof global !== 'undefined') { return global; } else { throw new Error('No global object found'); } })(); console.log(globalThis); // Works in both browser and Node.js
This snippet demonstrates a practical solution to the global object issue, but it also highlights a broader point: understanding the environment-specific nuances can help you write more robust code.
Another area where differences emerge is with Buffer
objects, which are unique to Node.js. Buffers are used to handle binary data, something that's not directly available in browsers. Here's a quick example:
// This will only work in Node.js const buffer = Buffer.from('Hello, World!'); console.log(buffer); // Outputs: <Buffer 48 65 6c 6c 6f 2c 20 57 6f 72 6c 64 21>
If you try to run this in a browser, you'll get an error because Buffer
is not defined. This is a clear example of how Node.js extends JavaScript with additional types that are not part of the standard ECMAScript specification.
When it comes to performance, there are also subtle differences. For instance, Node.js, being a server-side environment, often has different performance characteristics compared to browsers. Memory management, garbage collection, and even the JavaScript engine itself (V8 in both, but optimized differently) can lead to variations in how data types are handled.
From my experience, one of the trickiest aspects is dealing with Date
objects. While the Date
object itself is consistent across environments, the way time zones are handled can different. In a browser, the time zone is typically set by the user's system settings, whereas in Node.js, you might need to explicitly set the time zone:
// In Node.js process.env.TZ = 'America/New_York'; const date = new Date(); console.log(date); // Outputs date in New York time zone
This can lead to unexpected behavior if you're not careful, especially when dealing with applications that need to handle dates across different time zones.
In terms of best practices, always consider the environment in which your code will run. If you're writing code that needs to be cross-platform, use environment detection and conditional logic to handle differences. Also, be aware of the additional types and features available in Node.js, like Buffer
, and plan accordingly.
To wrap up, while the core JavaScript data types remain the same across browsers and Node.js, the way they're handled and the additional types available can lead to some interesting challenges and opportunities. By understanding these differences, you can write more versatile and robust JavaScript code that performs well in any environment.
So, keep exploring, keep learning, and don't be afraid to dive into the nuances of JavaScript across different platforms. It's these subtleties that make programming such a rewarding journey.
The above is the detailed content of Javascript Data Types : Is there any difference between Browser and NodeJs?. For more information, please follow other related articles on the PHP Chinese website!
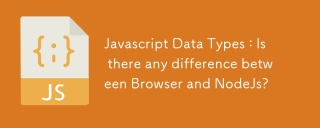
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
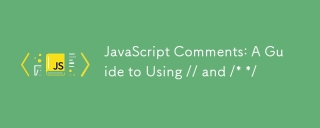
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
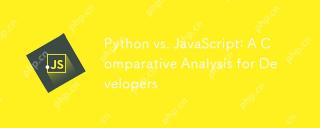
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
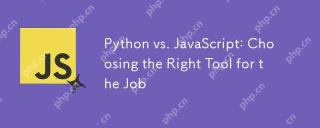
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
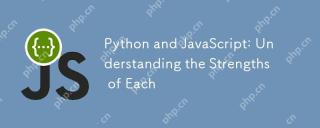
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
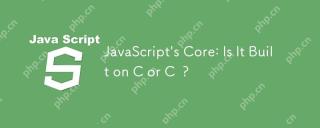
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
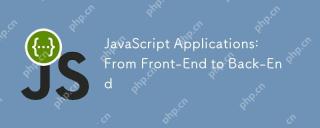
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
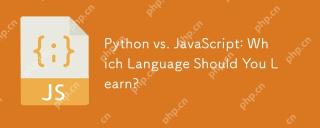
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
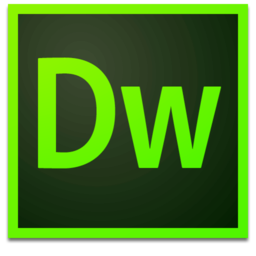
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
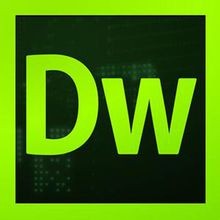
Dreamweaver CS6
Visual web development tools
