


JS object attributes related (check attributes, enumeration attributes, etc.)_javascript skills
1. Delete attributes
The delete operator can delete the properties of an object
delete person.age //That is, person no longer has the attribute age
delete person['age'] //or this
Delete only disconnects the attribute from the host object, but does not operate the attributes in the attribute. See that after deleting a.p, b.x is still 1
var a = {p:{x:1}}; var b = a.p; console.log(a.p.x); //1 delete a.p; console.log(a.p.x); //TypeError a.p is undefined console.log(a.p); //undefined console.log(b.x); //1
delete can only delete own properties, not inherited properties (to delete an inherited property, you must delete it from the prototype object that defines this property. Of course, this will affect all objects that inherit from this prototype)
function inherit(p){ if(p == null){ // 不能从null中继承 throw TypeError(); } if(Object.create){ //如果有这个方法就直接使用 return Object.create(p); } var t = typeof p; if(t !== "object" || t !== "function"){ //要继承的对象 类型要符合 throw TypeError(); } function f(){ }; //定义一个空的构造函数 f.prototype = p; //原型指向要继承的对象p return new f(); //创建f对象,此对象继承自p } var obj = {x:1}; var obj1 = inherit(obj); obj1.y = 2; console.log("x = "+obj1.x+" y = "+obj1.y); //x = 1 y = 2 delete obj1.x; delete obj1.y; console.log("x = "+obj1.x+" y = "+obj1.y); //x = 1 y = undefined
Of course, delete can only be used for configurable properties
For example
delete Object.prototype; // 不能删除 不可配置 var x = 1; delete this.x; //不能删除 this.y = 1; delete y; //这样可以删除 function f(){ } delete this.f; //不能删除
2. Detect attributes
Use "in"
Thein operator expects its left operand to be a string or convertible to a string, and its right operand to be an object
var data = [5,6,7]; console.log("0" in data); //有下标0 console.log(1 in data); //1可以转换成"1" console.log("4" in data); //下标只有 1 2 3 var obj = {x:1}; console.log("x" in obj); //true console.log("y" in obj); //false console.log("toString" in obj); //true 因为obj继承了这个方法
Use hasOwnProperty() or propertyIsEnumerable() --- the latter is an enhancement of the former
Gu Mingsi thought
var obj = {x:1}; console.log(obj.hasOwnProperty("x")); //true console.log(obj.hasOwnProperty("y")); //false console.log(obj.hasOwnProperty("toString")); //false 因为obj继承了这个方法,但不是它自己的
Only when it is detected that it is a free attribute and an enumerable attribute, the latter will return true
var obj = {x:1}; console.log(obj.propertyIsEnumerable("x")); //true console.log(obj.propertyIsEnumerable("y")); //false console.log(obj.propertyIsEnumerable("toString")); //false 因为obj继承了这个方法,但不是它自己的 console.log(Object.prototype.propertyIsEnumerable("toString")); //false 因为最原始的的 toString就是不可枚举的
Of course, you can also directly use the "!==" operator to determine
var obj = {x:1}; console.log(obj.x !== undefined);//true console.log(obj.y !== undefined);//false console.log(obj.toString !== undefined); //true
3. Enumeration attributes
var obj = {x:1,y:2}; for(p in obj){ console.log(p);//x y console.log(obj.p);// undefined undefined console.log(obj[p]);//1 2 }
Expansion 1:
Each object has its associated prototype, class, and extensibility
To detect whether an object is the prototype of another object (or is in a prototype chain), you can use the isPrototypeOf() method
var p = {x:1}; //p原型对象继承自Object.prototype var o = Object.create(p); //o对象继承自p console.log(p.isPrototypeOf(o));//true console.log(Object.prototype.isPrototypeOf(o));//true console.log(Object.prototype.isPrototypeOf(p));//true
Of course, the isPrototypeOf() method is very similar to the instanceof operator
The instanceof operator expects its left operand to be an object and its right operand to identify the class of the object. If the object on the left is an instance of the class on the right, the expression returns true, otherwise it returns false
var p = {x:1}; console.log(p instanceof Object);//true var d = new Date(); console.log(d instanceof Date);//true console.log(d instanceof Object);//true console.log(d instanceof Number);//false
Expansion 2:
The class attribute of an object is a string that represents the type information of the object
Generally, after calling the toString() method, it returns a form of [object class]
For example
var obj = {x:1,y:2};
console.log(obj.toString());//[object Object]
So if you want to get the class of the object, you can find the "class" field in the returned string and use slice (8, -1)
For example
function classOf(obj){ // 找出类名 if(obj === null){ return "Null"; } if(obj === undefined){ return "Undefined"; } return Object.prototype.toString.call(obj).slice(8,-1); } console.log(classOf(1)); //Number //注意,实际上是这些类型的变量调用toString()方法,而不是通过他们自身直接调用 //console.log(1.toString()); //会报错 var a = 1; console.log(Number(1).toString()); //1 console.log(a.toString()); //1 console.log({x:1}.toString()); //[object Object] console.log(classOf(1)); //Number console.log(classOf("")); //String console.log(classOf("str")); //String console.log(classOf(null)); //Null console.log(classOf(false)); //Boolean console.log(classOf({})); //Object console.log(classOf([])); //Array console.log(classOf(new Date())); //Date function f(){} console.log(classOf(new f())); //Object
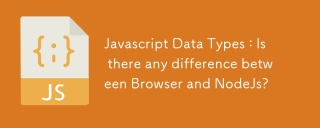
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
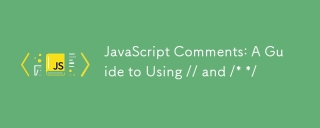
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
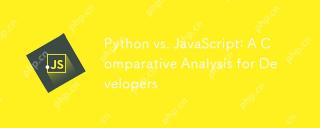
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
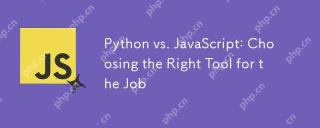
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
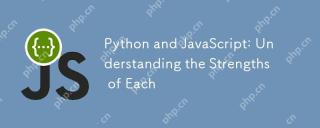
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
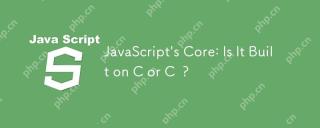
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
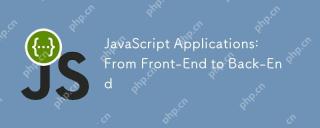
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
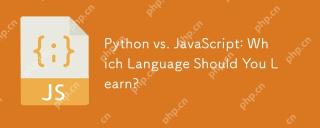
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
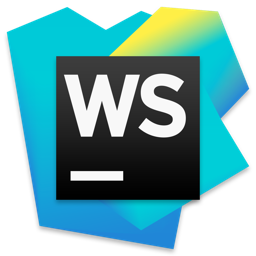
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
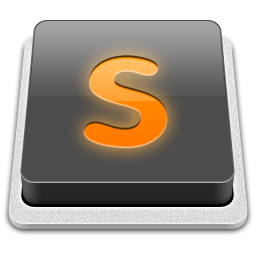
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
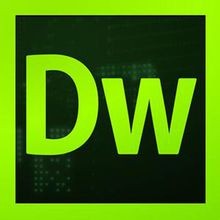
Dreamweaver CS6
Visual web development tools
