JVM thread management: a powerful tool for concurrent programming
Question: How does the JVM manage threads? Answer: Thread creation and destruction: Create a thread through the Thread class or Runnable interface, and destroy the thread through the stop(), interrupt() or interrupted() method. Thread scheduling: Using a preemptive scheduling algorithm, each thread has a priority that determines its running time. Thread synchronization: Ensure safe access to shared resources through locking, atomic variables, or CAS operations. Thread communication: Communication between threads is achieved through shared variables, message passing, or pipes.
JVM thread management: a powerful tool for concurrent programming
Thread management is a key link in concurrent programming, and the Java Virtual Machine (JVM) ) provides powerful support for thread management. This article will delve into the thread management mechanism of JVM and demonstrate its application through practical cases.
Thread creation and destruction
Threads can be created through the Thread
class or the Runnable
interface. The following code shows how to create a thread:
class MyThread extends Thread { @Override public void run() { // 线程代码 } } MyThread thread = new MyThread(); thread.start();
Thread destruction can be achieved through the stop()
or interrupt()
method. However, it is recommended to use the interrupted()
method to determine whether the thread is interrupted, and then exit the thread yourself inside the loop.
Thread Scheduling
JVM uses a preemptive scheduling algorithm to manage threads. Each thread has a priority that determines the period during which it runs. The priority can be set through the setPriority()
method.
Thread Synchronization
Synchronization is a means of ensuring that shared resources (such as variables or objects) can be accessed safely in a concurrent environment. The JVM provides the following synchronization mechanism:
-
Lock: Use the
synchronized
keyword orReentrantLock
to lock resources. -
Atomic variables: Use atomic variables such as
AtomicInteger
orAtomicReference
. -
CAS: Use the
compareAndSet()
method to perform a compare and swap operation to update shared variables.
Thread communication
Communication between threads can be achieved in the following ways:
- Shared variables: Threads share access to the same variable.
-
Message delivery: Use message queues such as
BlockingQueue
orConcurrentLinkedQueue
to deliver messages. -
Pipeline: Use
PipedInputStream
andPipedOutputStream
to create pipelines for data flow communication.
Practical case
Producer-consumer queue
The following code shows a use BlockingQueue
Implemented producer-consumer queue:
import java.util.concurrent.BlockingQueue; class Producer implements Runnable { private BlockingQueue<Integer> queue; @Override public void run() { for (int i = 0; i < 10; i++) { queue.put(i); } } } class Consumer implements Runnable { private BlockingQueue<Integer> queue; @Override public void run() { while (!queue.isEmpty()) { Integer item = queue.take(); // 处理 item } } } BlockingQueue<Integer> queue = new ArrayBlockingQueue<>(10); Producer producer = new Producer(); Consumer consumer = new Consumer(); Thread producerThread = new Thread(producer); producerThread.start(); Thread consumerThread = new Thread(consumer); consumerThread.start();
Conclusion
The thread management mechanism of JVM provides powerful support for concurrent programming. By understanding thread creation, scheduling, synchronization, and communication, developers can effectively write concurrent code and improve application performance and reliability.
The above is the detailed content of JVM thread management: a powerful tool for concurrent programming. For more information, please follow other related articles on the PHP Chinese website!
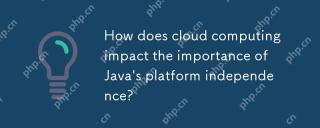
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
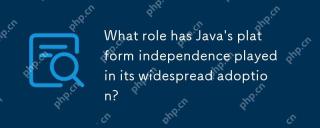
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
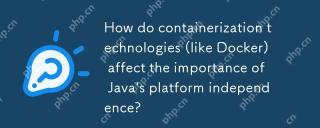
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.
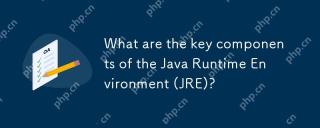
JRE is the environment in which Java applications run, and its function is to enable Java programs to run on different operating systems without recompiling. The working principle of JRE includes JVM executing bytecode, class library provides predefined classes and methods, configuration files and resource files to set up the running environment.
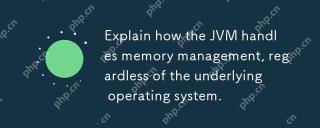
JVM ensures efficient Java programs run through automatic memory management and garbage collection. 1) Memory allocation: Allocate memory in the heap for new objects. 2) Reference count: Track object references and detect garbage. 3) Garbage recycling: Use the tag-clear, tag-tidy or copy algorithm to recycle objects that are no longer referenced.
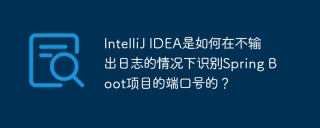
Start Spring using IntelliJIDEAUltimate version...
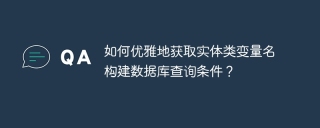
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
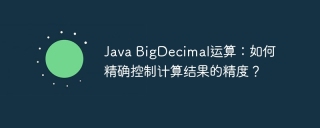
Java...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
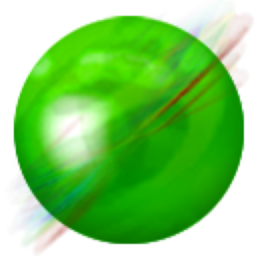
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
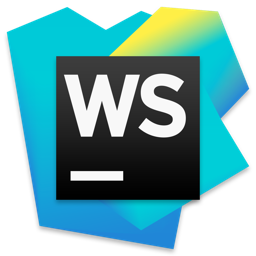
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor