Confusion for Java Beginners: Application of Algorithms and Data Structures
#Java Beginner’s Guide: Real-life Applications of Algorithms and Data Structures
Algorithms and data structures are the cornerstones of Java programming. Understanding their application is critical to writing efficient, maintainable code. This article explores common uses of algorithms and data structures in real-world scenarios to help you understand their value.
Sort algorithm
The sort algorithm is used to arrange the list of elements in an orderly manner. For example:
int[] numbers = {5, 2, 8, 3, 9}; // 使用快速排序算法对 numbers 数组进行排序 Arrays.sort(numbers); // 输出排序后的数组 for (int number : numbers) { System.out.println(number); }
Output:
2 3 5 8 9
Find algorithm
Find algorithm is used to search for a specific element in a list or data structure. For example:
String[] names = {"John", "Mary", "Bob", "Alice"}; // 使用二分查找算法在 names 数组中查找 "Bob" int index = Arrays.binarySearch(names, "Bob"); // 输出索引或未找到的指示 if (index >= 0) { System.out.println("Bob 的索引:" + index); } else { System.out.println("未找到 Bob"); }
Output:
Bob 的索引:2
Data structure
Data structures are used to organize and store data so that it can be accessed and manipulated quickly and efficiently. A common example is a list:
// 创建一个 ArrayList 以存储整数组 List<Integer> numbers = new ArrayList<>(); // 添加元素 numbers.add(5); numbers.add(2); numbers.add(8); // 访问元素(基于索引) int secondNumber = numbers.get(1);
Output:
2
Queue and stack
Queue and stack are a special linear data structure that follows the first-in-first-out (FIFO ) and the last-in-first-out (LIFO) principle. They are common in the following scenarios:
- Queue: Processing tasks, message queues, print requests
- Stack: Recursive function calls , bracket matching, undo/redo operations
Maps and Collections
Maps and collections are data structures used to store and retrieve key-value pairs and unique elements. For example:
// 创建一个 HashMap 以存储名称和分数 Map<String, Integer> scores = new HashMap<>(); // 添加元素 scores.put("John", 90); scores.put("Mary", 85); // 基于键获取值 int johnScore = scores.get("John");
Output:
90
Summary
Algorithms and data structures are an integral part of Java programming. They enable us to efficiently solve complex problems and organize and manage data. By understanding their application in real-world scenarios, you'll be able to improve the efficiency, readability, and maintainability of your code.
The above is the detailed content of Confusion for Java Beginners: Application of Algorithms and Data Structures. For more information, please follow other related articles on the PHP Chinese website!
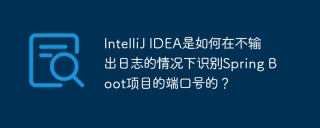
Start Spring using IntelliJIDEAUltimate version...
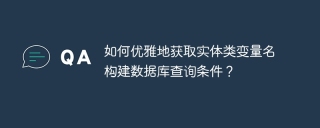
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
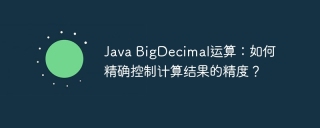
Java...
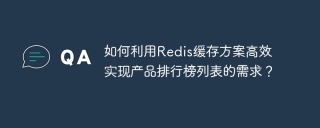
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
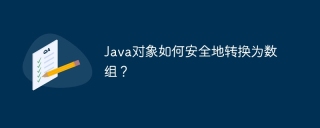
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
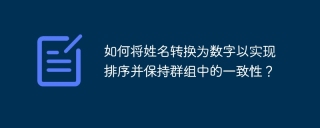
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
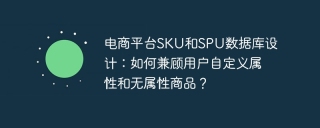
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
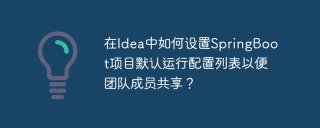
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
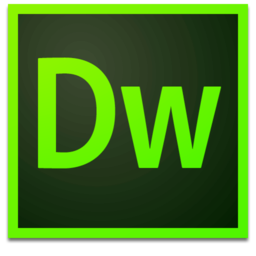
Dreamweaver Mac version
Visual web development tools
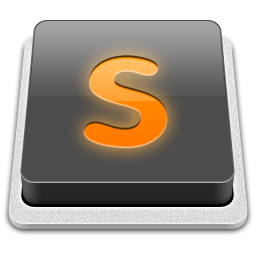
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
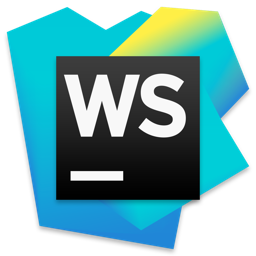
WebStorm Mac version
Useful JavaScript development tools