


Introduction to Java Basics to Practical Applications: Frequently Asked Questions
Getting Started with Java: Create and run simple programs, and become familiar with the syntax and compilation process. Loops: Use a for loop to repeatedly execute a block of code. Array: Declare the array type, length, and fill elements. Method: Use classes to encapsulate code blocks and pass parameters to call methods. Exception handling: Use try-catch blocks to catch and handle exceptions. Practical application: Calculate and print the Fibonacci sequence using arrays and loops.
Java Basics to Practical Applications: Frequently Asked Questions
Question 1: How to get started with Java?
public class Main { public static void main(String[] args) { System.out.println("Hello, Java!"); } }
To get started with Java, create and run a simple program (like the example above) to become familiar with Java syntax and the compilation process.
Question 2: How to use loops?
for (int i = 0; i < 10; i++) { System.out.println(i); }
This loop will print out all numbers from 0 to 9 (including 0). Use a for
loop to repeatedly execute a block of code.
Question 3: How to use arrays?
int[] numbers = new int[5]; numbers[0] = 1; numbers[1] = 2;
An array is a container that stores values of the same type. To use an array, declare the array type, length, and padding elements.
Question 4: How to use the method?
public class MyClass { public static int sum(int a, int b) { return a + b; } }
Methods are functions that encapsulate a block of code to perform a specific task. To use a method, call it by its name and pass the appropriate parameters.
Question 5: How to use exception handling?
try { // 可能引发异常的代码 } catch (Exception e) { // 处理异常 }
Exception handling is a mechanism for handling errors when a program is running. try-catch
blocks allow you to catch exceptions and provide custom handling.
Practical case: Calculating the Fibonacci sequence
import java.util.Scanner; public class Fibonacci { public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter the number of terms: "); int n = input.nextInt(); int[] fib = new int[n]; // 初始化斐波那契数列 fib[0] = 0; fib[1] = 1; // 计算斐波那契数列 for (int i = 2; i < n; i++) { fib[i] = fib[i - 1] + fib[i - 2]; } // 打印斐波那契数列 for (int i : fib) { System.out.print(i + " "); } System.out.println(); } }
This program uses an array to store the Fibonacci sequence and uses a loop to calculate the value of each sequence item. , and print the final result.
The above is the detailed content of Introduction to Java Basics to Practical Applications: Frequently Asked Questions. For more information, please follow other related articles on the PHP Chinese website!
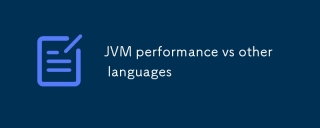
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
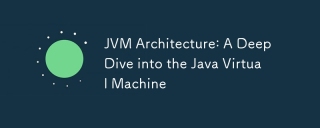
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
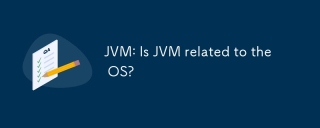
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
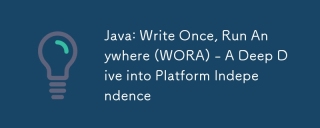
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
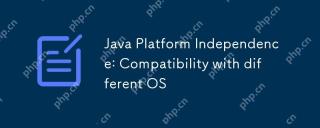
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
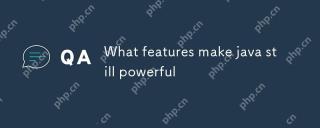
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
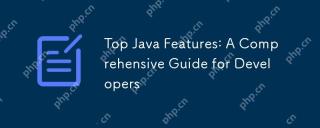
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
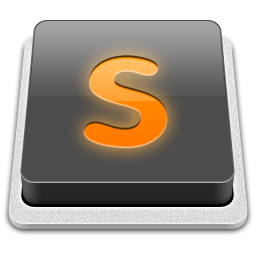
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
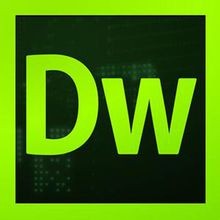
Dreamweaver CS6
Visual web development tools
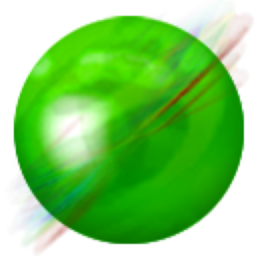
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
