JVM has a close relationship with the OS as it translates Java bytecode into machine-specific instructions, manages memory, and handles garbage collection. This relationship allows Java to run on various OS environments, but it also presents challenges like different JVM behaviors and OS-specific limitations.
JVM, or Java Virtual Machine, indeed has a close relationship with the operating system (OS). Let's dive into this fascinating world where Java meets the OS.
When you think about JVM, imagine it as a translator and a guardian. It translates your Java bytecode into machine-specific instructions that the OS can understand and execute. This is crucial because Java's "write once, run anywhere" philosophy relies on the JVM to adapt to different OS environments, whether it's Windows, Linux, macOS, or even more exotic systems.
Now, let's explore how this relationship plays out in practice.
The JVM sits between your Java code and the OS, acting as an intermediary. It's not just about translation; the JVM also manages memory, handles garbage collection, and ensures that your Java programs run smoothly. This means the JVM needs to be aware of the OS's capabilities and limitations. For instance, the JVM will use different strategies for memory management on a 32-bit versus a 64-bit OS.
Here's a little personal anecdote: I once worked on a project where we had to optimize a Java application for a specific Linux distribution. We noticed that the JVM's garbage collection was causing noticeable pauses in our application. By tweaking JVM parameters and understanding how the Linux kernel handled memory, we were able to significantly reduce these pauses. It was a great lesson in how the JVM and OS can work together—or sometimes, against each other.
Now, let's look at some code to see how the JVM interacts with the OS. Here's a simple Java program that demonstrates how the JVM can access OS-specific information:
import java.lang.management.ManagementFactory; import java.lang.management.OperatingSystemMXBean; public class OSInfo { public static void main(String[] args) { OperatingSystemMXBean osBean = ManagementFactory.getOperatingSystemMXBean(); System.out.println("OS Name: " osBean.getName()); System.out.println("OS Version: " osBean.getVersion()); System.out.println("OS Architecture: " osBean.getArch()); System.out.println("Available Processors: " osBean.getAvailableProcessors()); System.out.println("System Load Average: " osBean.getSystemLoadAverage()); } }
This code uses the OperatingSystemMXBean
to fetch OS-specific details. It's a neat way to see how the JVM can interact with the underlying OS to provide useful information.
But it's not all sunshine and rainbows. There are challenges and pitfalls to consider. For instance, different JVM implementations (like Oracle JDK, OpenJDK, or IBM J9) might behave differently on the same OS. This can lead to unexpected behavior or performance issues. Also, the JVM's abstraction layer can sometimes hide OS-specific features that could be beneficial for performance tuning.
To navigate these waters, here are some tips and insights:
Understand JVM Parameters: The JVM comes with a plethora of command-line options that can be tuned to better fit your OS environment. For example,
-XX:MaxRAMPercentage
can help you control how much memory the JVM uses on your system.Monitor and Profile: Use tools like JConsole or VisualVM to monitor JVM performance and see how it interacts with the OS. This can help you identify bottlenecks or issues related to OS-specific behavior.
Be Aware of OS Limitations: Different OS versions might have different limits on resources like file descriptors or threads. Make sure your JVM configuration respects these limits.
Test Across Environments: Since the JVM abstracts away many OS details, it's crucial to test your application on different OS environments to ensure compatibility and performance.
In conclusion, the JVM's relationship with the OS is both intimate and complex. It's a dance of translation, optimization, and sometimes, compromise. By understanding this relationship, you can better harness the power of Java across different operating systems, turning potential headaches into opportunities for optimization and innovation.
The above is the detailed content of JVM: Is JVM related to the OS?. For more information, please follow other related articles on the PHP Chinese website!
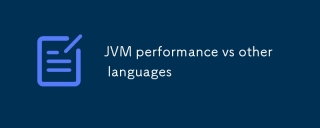
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
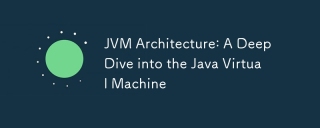
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
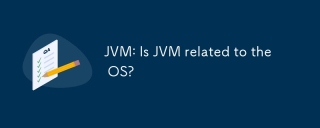
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
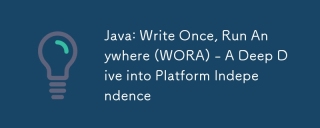
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
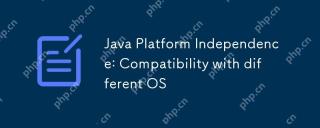
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
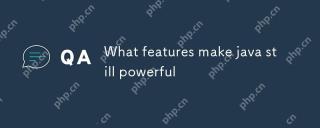
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
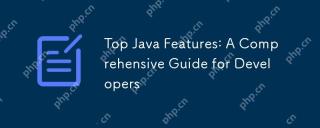
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
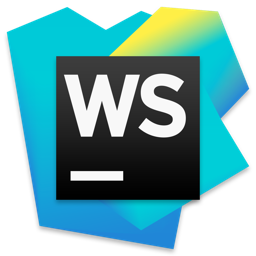
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
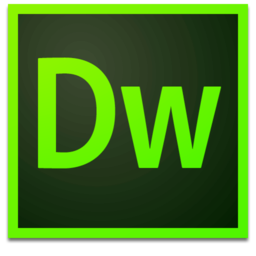
Dreamweaver Mac version
Visual web development tools
