GUI programming tool: Java provides Swing and AWT toolkits for creating user-friendly graphical interfaces. Swing is more feature-rich and AWT is more lightweight. GUI programming pitfalls include: cross-platform compatibility issues, complexity, and performance issues. Practical case: Use Swing to create a text input and display window, demonstrating the application of GUI programming.
Java Beginner’s Confusion: GUI Programming Tools and Pitfalls
GUI Programming Tool
Java provides powerful GUI programming toolkits (Swing and AWT) to help developers quickly create user-friendly graphical interfaces.
Swing: A more feature-rich toolkit that provides a wider range of controls and customization options.
import javax.swing.*; public class SimpleGUI { public static void main(String[] args) { JFrame frame = new JFrame("简单 GUI"); frame.setSize(400, 300); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }
AWT: A more lightweight toolkit targeted at smaller and simpler GUIs.
import java.awt.*; public class AWTExample { public static void main(String[] args) { Frame frame = new Frame("AWT 示例"); frame.setSize(400, 300); frame.setVisible(true); } }
Pitfalls in GUI programming
Cross-platform compatibility issues: Swing and AWT are based on native components, which may lead to differences in appearance and behavior on different platforms Inconsistent.
Complexity: Creating and managing complex GUI interfaces can become very complex, especially when large amounts of user interaction are involved.
Performance Issues: GUI components have high resource requirements, and large-scale or animation-intensive applications may experience performance issues.
Practical case
Create a simple text input and display window:
import javax.swing.*; public class TextInputGUI { public static void main(String[] args) { // 创建一个文本字段和按钮 JTextField textField = new JTextField(); JButton button = new JButton("显示"); // 为按钮添加事件侦听器 button.addActionListener(e -> { String text = textField.getText(); JOptionPane.showMessageDialog(null, text); }); // 创建面板并添加组件 JPanel panel = new JPanel(); panel.add(textField); panel.add(button); // 创建帧并添加面板 JFrame frame = new JFrame("文本输入 GUI"); frame.add(panel); frame.setSize(400, 300); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }
The above is the detailed content of Confusion for Java Beginners: Tools and Pitfalls of GUI Programming. For more information, please follow other related articles on the PHP Chinese website!
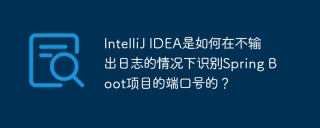
Start Spring using IntelliJIDEAUltimate version...
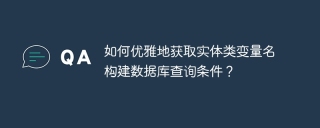
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
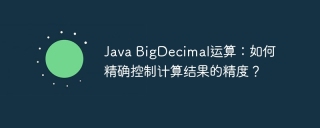
Java...
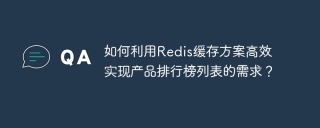
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
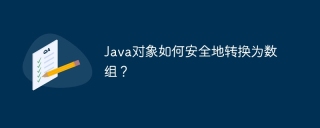
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
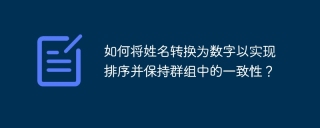
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
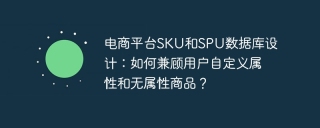
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
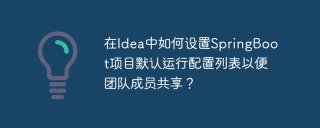
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
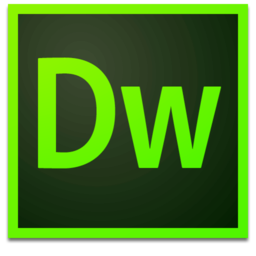
Dreamweaver Mac version
Visual web development tools
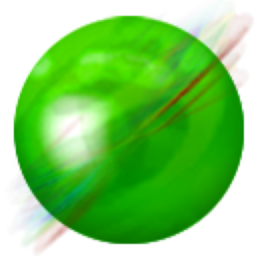
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software