


Troublesome problems faced by newbies in Golang technology and their analysis
Common troublesome problems and solutions for Golang novices: Input/output operations: Use the standard io package to import correctly, use os.Open() or os.Create() operations, handle errors, and use correct permissions. Function handling: Force passing by value or use pointer passing, use reference passing only when needed. Concurrent programming: Use synchronization mechanisms, use coroutines carefully, and utilize concurrency safety libraries. HTTP request handling: verify request paths, inspect controllers, debug middleware.
Troublesome problems faced by Golang novices and their analysis
As a Golang novice, you may have encountered some thorny challenges. This article will dive into some common pain points and provide clear solutions to help you embark on a successful Golang journey.
1. Input/Output Operations
Problem: An error was encountered while trying to use the standard io
package to read and write files.
Parsing:
Make sure the necessary packages have been imported correctly: import "os"
Use os.Open()
or os.Create()
Start reading and writing operations.
Handling errors: Use defer
to ensure the file is closed even if an error occurs.
Make sure to use the correct file permissions: use os.O_RDONLY
for read-only operations and os.O_WRONLY
for write-only operations.
2. Function handling
Problem: A parameter passed to a function is passed as a reference, causing unexpected behavior.
Analysis:
Clearly pass the type of parameter: use func(argType)
to force value passing.
Use *
Pointers: Use func(argType *Type)
to declare function parameters as pointers.
Make sure to use pass-by-reference only when needed: pass-by-value is usually preferred because it prevents accidental modifications and data races.
3. Concurrent programming
Problem: In a concurrent environment, data race conditions lead to unpredictable behavior.
Analysis:
Use synchronization mechanism: sync.Mutex
, sync.WaitGroup
, etc. to prevent concurrent access to shared resources.
Be careful when using go coroutines: ensure the security of coroutines and avoid modifying shared state.
Use concurrency-safe libraries: Investigate built-in packages such as sync
and context
to simplify concurrent programming.
4. HTTP request processing
Problem: 404 or 500 errors are encountered when processing HTTP requests.
Parsing:
Verify the request path: Ensure that the requested URL correctly matches the route.
Check Controller: The handler function must handle the correct HTTP method and return a status code.
Debug middleware: If something goes wrong in the middleware, it may prevent the request from reaching the controller.
Practical case:
Create a simple HTTP server, listen to port 8080 and print "Hello, Gophers":
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, Gophers!") }) http.ListenAndServe(":8080", nil) }
Learning Golang can be an exciting time journey of. By understanding these common pitfalls and following the clear explanations provided in this article, you can confidently overcome challenges and build great Golang applications.
The above is the detailed content of Troublesome problems faced by newbies in Golang technology and their analysis. For more information, please follow other related articles on the PHP Chinese website!
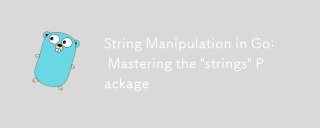
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
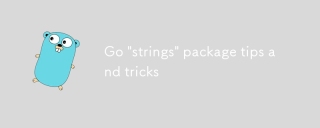
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
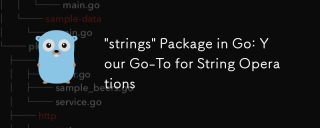
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
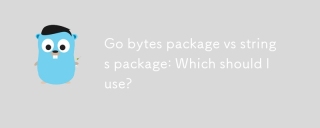
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
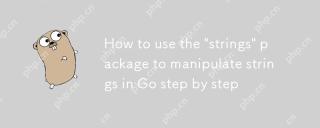
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
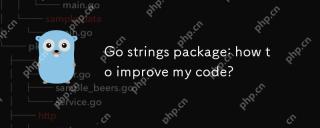
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
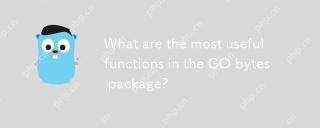
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
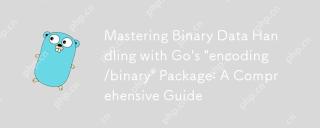
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
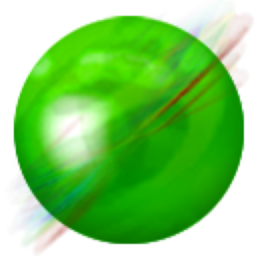
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
