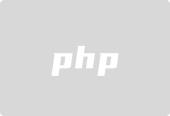
How to use PHP functions to process data efficiently
PHP provides a powerful function library that can efficiently process various data types. Understanding these functions will greatly simplify your code and improve its performance.
Array processing function
-
array_map(): Apply the callback function to each element in the array and return an array containing the result.
-
array_filter(): Filter the array and only retain elements that match the callback function.
-
array_reduce(): Pass the array elements to the callback function one by one and return a cumulative result.
-
array_unique(): Remove duplicate elements from the array.
-
sort()/rsort(): Sort the array (forward or reverse order).
String processing function
-
strlen(): Returns the length of the string.
-
strtoupper()/strtolower(): Convert string to uppercase/lowercase.
-
explode(): Split the string using the delimiter as the delimiter.
-
implode(): Connect arrays using delimiters as delimiters.
-
ereg_replace(): Replace the content in the string with a regular expression pattern.
Mathematical processing function
-
abs(): Returns the absolute value of the number.
-
max()/min(): Returns the maximum/minimum value in an array or multiple numbers.
-
round(): Rounds a number to the specified precision.
-
pow(): Returns the power of the number.
-
log(): Returns the natural logarithm of the number.
Date and time processing functions
-
date(): Return the current date and time according to the specified format.
-
strtotime(): Convert a date/time string to a timestamp.
-
mktime(): Create a timestamp (from the given year, month, day, hour, minute, and second).
-
time(): Returns the current timestamp.
-
gmstrftime(): Format time in GMT time zone.
Practical case
Processing large arrays containing student scores:
<?php
// 初始数组
$grades = [
['name' => 'John', 'grade' => 90],
['name' => 'Mary', 'grade' => 85],
['name' => 'Bob', 'grade' => 75],
['name' => 'Alice', 'grade' => 95],
];
// 使用 array_map() 计算平均成绩
$average_grades = array_map(function($student) {
return $student['grade'] / 100;
}, $grades);
// 使用 array_filter() 获取平均成绩大于 0.9 的学生
$top_students = array_filter($grades, function($student) {
return $student['grade'] / 100 > 0.9;
});
// 使用 array_reduce() 计算总平均成绩
$total_average = array_reduce($average_grades, function($carry, $item) {
return $carry + $item;
}, 0) / count($average_grades);
// 打印结果
echo "平均成绩:", $total_average, "\n";
echo "平均成绩大于 0.9 的学生:", implode(", ", array_column($top_students, 'name')), "\n";
The above is the detailed content of How to use PHP functions to process data efficiently?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn