


Go function performance optimization: impact of garbage collection mechanism and performance
Garbage collection (GC) has an impact on Go function performance because it interrupts execution by pausing the program to reclaim memory. Optimization strategies include: Reduce allocations Use pools Avoid allocations in loops Use pre-allocated memory Profile Application
Go function performance optimization: garbage collection mechanism and performance Impact
Preface
Garbage collection (GC) is an efficient mechanism for automatically managing memory in the Go language. However, GC can have an impact on function performance. This article will explore the impact of garbage collection in Go and provide practical examples of optimizing function performance.
Garbage Collection Overview
Garbage collection in Go consists of an allocator and a collector. The allocator is responsible for allocating memory, and the collector is responsible for reclaiming memory that is no longer in use. The GC process consists of the following steps:
- The allocator allocates a memory block to store new data.
- If the memory block is full, the allocator will ask the GC to reclaim the memory.
- GC pauses the program, scans the objects in the heap and marks objects that are no longer used.
- GC reclaims marked objects and releases memory.
Garbage collection and function performance
GC pauses interrupt program execution, thus affecting function performance. The pause time depends on the number of objects in the heap and the activity level of the application.
Practical case: Optimizing function performance
In order to reduce the impact of GC pauses on function performance, you can consider the following optimization strategies:
- Reduce allocation: Use allocated memory as much as possible to avoid unnecessary allocation.
- Use a pool: For frequently allocated structures or slices, using a pool can reduce allocation and GC pressure.
- Avoid allocations in loops: Allocating objects in a loop can generate a lot of GC allocations. Instead, you can allocate once outside the loop and then modify it using the loop variable.
- Use preallocated memory: Pre-allocate a block of memory and reuse it instead of allocating a new block each time.
- Profile Application: Profile your application's allocation and GC performance using profiling tools such as pprof to identify performance bottlenecks.
Code Example
The following code example demonstrates how to optimize function performance by reducing allocations and using pools:
// 原始函数 func SlowFunction(n int) []int { res := []int{} for i := 0; i < n; i++ { res = append(res, i) // 分配新的切片 } return res } // 优化后的函数 func FastFunction(n int) []int { res := make([]int, n) // 预分配切片 for i := 0; i < n; i++ { res[i] = i // 修改现有切片 } return res }
In this example , SlowFunction
allocates multiple new slices in a loop, while FastFunction
pre-allocates a slice and reuses it, thus avoiding a lot of GC allocations.
Conclusion
By understanding the impact of the garbage collection mechanism on Go function performance, we can leverage optimization strategies to reduce GC pauses and improve application performance. By reducing allocations, using pools, avoiding allocations in loops, using preallocated memory, and profiling the application, we can optimize functions and achieve better performance.
The above is the detailed content of Go function performance optimization: impact of garbage collection mechanism and performance. For more information, please follow other related articles on the PHP Chinese website!
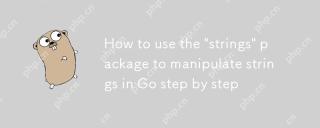
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
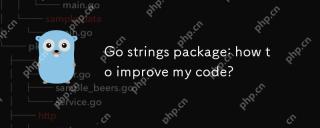
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
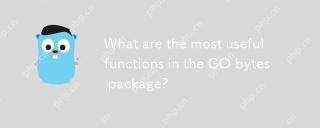
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
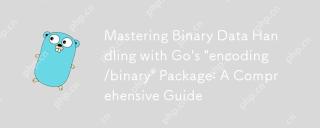
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
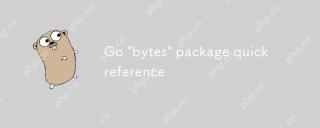
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
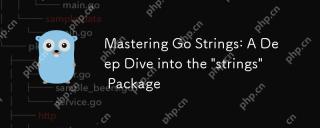
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
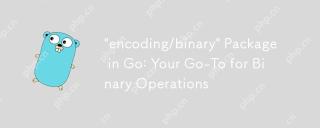
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
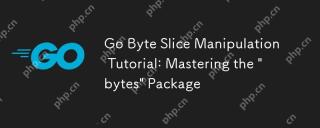
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
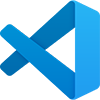
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
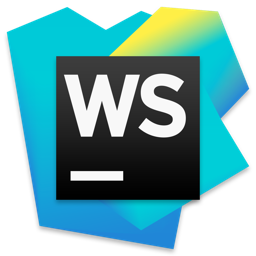
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Notepad++7.3.1
Easy-to-use and free code editor
