Resolve race conditions in function pipeline communication: Use concurrency safety types (sync.Mutex) to synchronize access to pipeline data. Add buffering to the pipeline to temporarily store data and prevent data contention between goroutines. Limit the number of goroutines executing the function pipeline simultaneously, forcing serial execution.
Avoiding race conditions in Go language function pipeline communication
The essence of concurrent pipeline communication
In the Go language, pipes are a mechanism used for communication between goroutines. They are inherently concurrency safe, meaning there can be multiple goroutines reading and writing to the pipe at the same time.
Race conditions
However, when using function pipelines, race conditions may occur. This refers to unexpected behavior that can occur when multiple goroutines execute a function pipeline simultaneously. Specifically, it can cause unexpected output ordering or data loss.
Avoiding race conditions
There are several ways to circumvent race conditions in function pipelines:
Use concurrency-safe types
Use a concurrency-safe type (such as sync.Mutex
) to synchronize access to pipe data. This prevents race conditions by allowing only one goroutine to access the data at a time.
package main import ( "sync" ) func main() { var m sync.Mutex numbers := make([]int, 10) for i := 0; i < 10; i++ { go func(i int) { m.Lock() defer m.Unlock() numbers[i] = i * i }(i) } // 等待所有goroutine完成 }
Using channel buffering
By adding buffering to the pipe, we can temporarily store data and prevent data contention between goroutines.
package main func main() { // 创建一个通道,缓冲为 1 numbers := make(chan int, 1) for i := 0; i < 10; i++ { go func(i int) { // 写入通道,由于通道缓冲为 1,因此最多会有一个goroutine在写入 numbers <- i * i }(i) } // 从通道中读取 for i := 0; i < 10; i++ { fmt.Println(<-numbers) } }
Limit the number of goroutines
By limiting the number of goroutines that can execute a function pipeline at the same time, we can force serial execution and thus prevent race conditions.
package main import ( "context" "sync" ) func main() { // 创建带有并发限制 1 的goroutine池 pool, _ := context.WithCancel(context.Background()) poolSize := 1 wg := sync.WaitGroup{} for i := 0; i < 10; i++ { wg.Add(1) go func(i int) { defer wg.Done() // 限制goroutine池中的并发执行数量 _ = pool.Err() // 访问管道数据 } } }
By applying these techniques, we can avoid race conditions in function pipelines and ensure the reliability and correctness of concurrent operations.
The above is the detailed content of Avoiding race conditions in golang function pipeline communication. For more information, please follow other related articles on the PHP Chinese website!
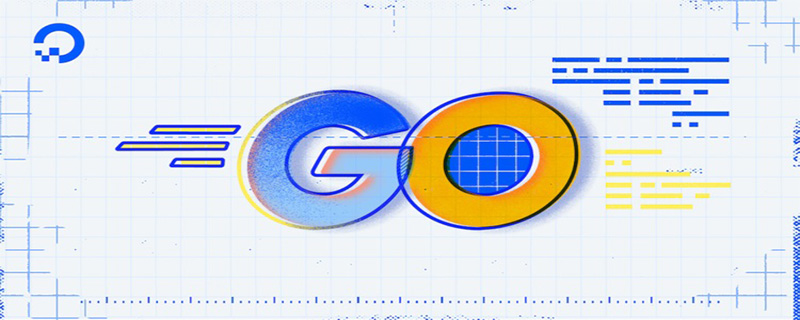
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
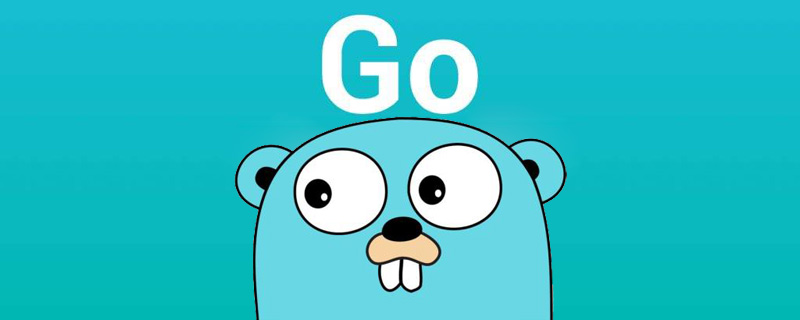
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
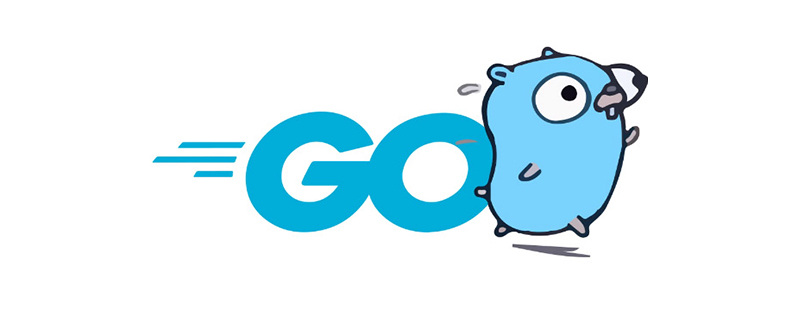
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
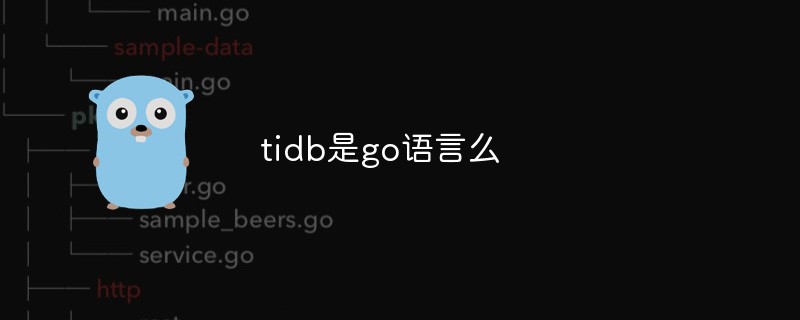
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
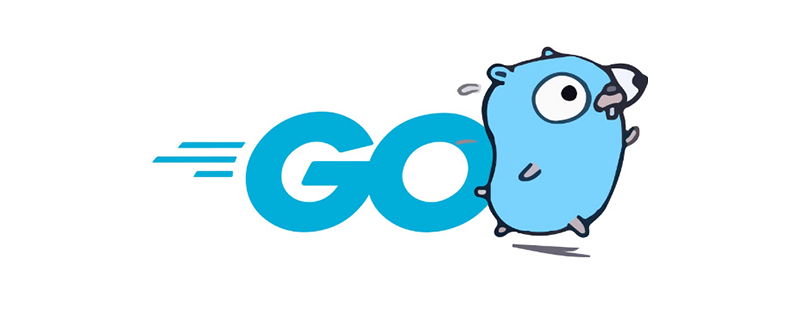
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
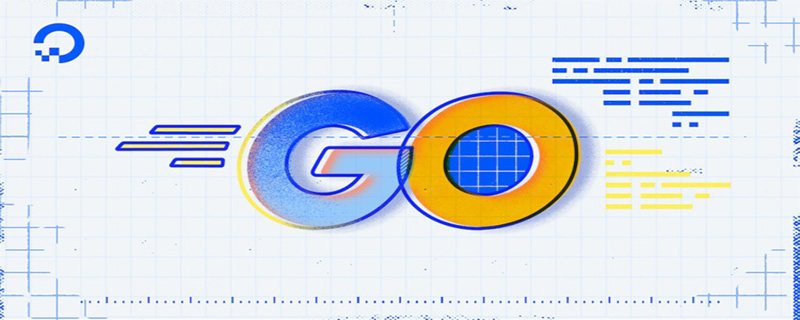
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
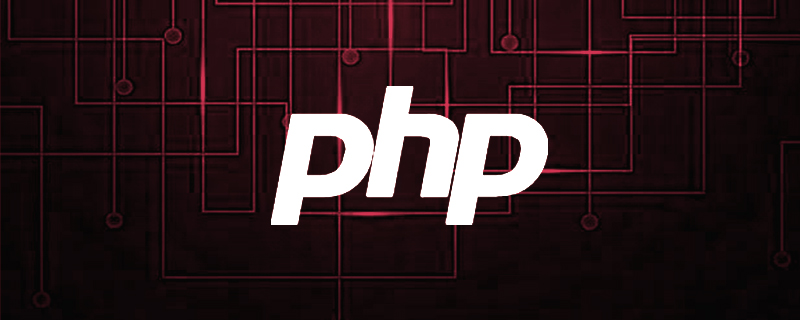
发现 Go 不仅允许我们创建更大的应用程序,并且能够将性能提高多达 40 倍。 有了它,我们能够扩展使用 PHP 编写的现有产品,并通过结合两种语言的优势来改进它们。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
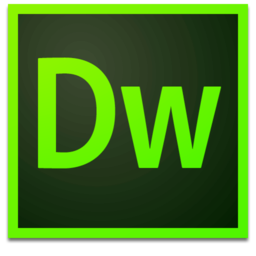
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
